Flask Request Form
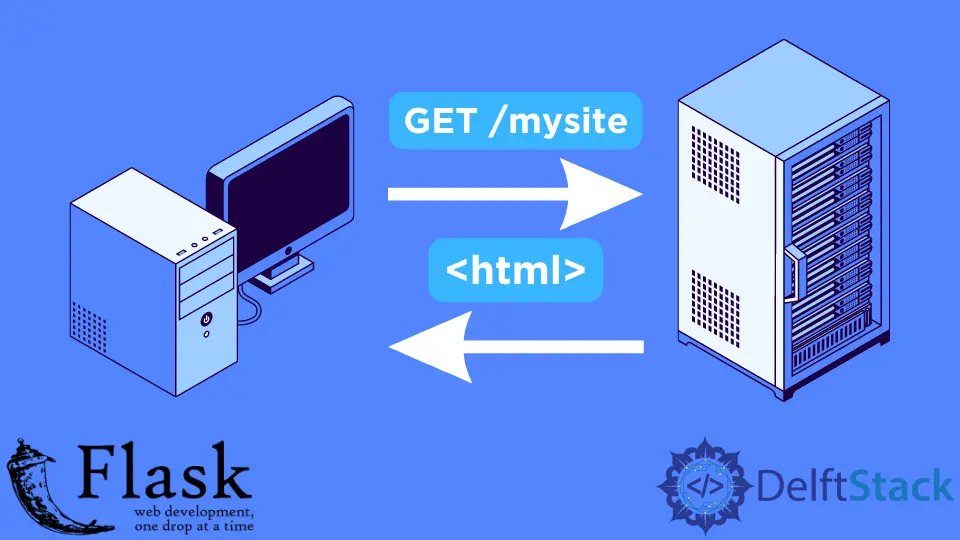
We will learn, with this explanation, about two things that happen when we submit a form and how we can receive and get the form data with the help of Flask and Python.
Receive and Get the Form Data With the Help of Flask and Python
Browsers make requests to servers; that is how they communicate. Get
requests are normally used to retrieve data from a server or ask the server for some data; however, a request is just a few pieces of data that a browser sends to a server.
The server then sees those pieces and hopefully understands what they mean. For example, the get
part of a request is just a string included in the request.
When the server sees that, and if it is programmed to respond to get
requests, it can respond. But instead of making a get
request, you could make a sandwich
request.
The server would have no idea what you are talking about unless you coded the server to understand that specifically.
If you used a sandwich
request, you would no longer be making an HTTP request. HTTP is a protocol which means a set of rules, and if you follow the rules like using HTTP methods that actually exist, then you are using HTTP, and if you do not, then you are not.
This is important because if we want to use HTTP, there are rules we need to know. For example, post
requests are another type of request that can have a payload called a body.
That is longer data, and it is included inside the request; that could be a string of data or something more formalized like JSON. It could also be a file, or it could be form data.
Some HTTP requests can have a payload, and others can not. Get
requests, for example, can not have a payload but post
and put
can.
We can tell our form to use different request types depending on how we want it to send data. If we tell our form to use get
, then it can not put the data in the payload; it puts it in the URL.
If we tell it to use post
, it will put the data in the payload.
Let’s create a Flask app to receive this form data like the post
. We will create a file called app.py
that will contain our Flask code, and we will also create a virtual environment and install a Flask in it.
We know that Flask uses functions to respond to browser requests, and now we want to respond to the browser request with our HTML page so that the browser can actually display it when we first access the Flask app.
To do that with the Flask, we need to do some initial setup. We will need to create a templates
folder, which has to be in the same place as app.py
.
Inside templates
, we are going to put our index.html
file; then, inside this file, we will use the bootstrap starter template that is open source for everyone.
Then we find the body
tag and write a basic HTML form with two fields.
<!doctype html>
<html lang="en">
<head>
<!-- Required meta tags -->
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<!-- Bootstrap CSS -->
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-EVSTQN3/azprG1Anm3QDgpJLIm9Nao0Yz1ztcQTwFspd3yD65VohhpuuCOmLASjC" crossorigin="anonymous">
<title>Hello, world!</title>
</head>
<body>
<h1>Hello, this is about flask!</h1>
<form action="#">
<p>Name:</p>
<p><input type="text" name="candidate-name" /></p>
<p>City:</p>
<p><input type="text" name="candidate-city" /></p>
<p><input type="submit" value="submit"/></p>
</form>
<!-- Optional JavaScript; choose one of the two! -->
<!-- Option 1: Bootstrap Bundle with Popper -->
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/js/bootstrap.bundle.min.js" integrity="sha384-MrcW6ZMFYlzcLA8Nl+NtUVF0sA7MsXsP1UyJoMp4YLEuNSfAP+JcXn/tWtIaxVXM" crossorigin="anonymous"></script>
<!-- Option 2: Separate Popper and Bootstrap JS -->
<!--
<script src="https://cdn.jsdelivr.net/npm/@popperjs/core@2.9.2/dist/umd/popper.min.js" integrity="sha384-IQsoLXl5PILFhosVNubq5LC7Qb9DXgDA9i+tQ8Zj3iwWAwPtgFTxbJ8NT4GN1R8p" crossorigin="anonymous"></script>
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/js/bootstrap.min.js" integrity="sha384-cVKIPhGWiC2Al4u+LWgxfKTRIcfu0JTxR+EQDz/bgldoEyl4H0zUF0QKbrJ0EcQF" crossorigin="anonymous"></script>
-->
</body>
</html>
Then, we need to use the Flask to return the HTML code to the user. To do this, we must import a Flask and render_template
inside app.py
.
Now, we will need to add an endpoint using a decorator, then return render_template()
of index.html
.
Let’s import a request
from the Flask, and inside our endpoint, add a print
statement to display the contents of request.args
where the Flask will put the query strings, and it will receive any request.
from flask import Flask, render_template, request
app = Flask(__name__)
@app.route("/")
def INDEX():
print(request.args)
return render_template("index.html")
if __name__ == "__main__":
app.run(debug=True)
Note that we can only access request.args
inside a function that responds to a request.
Now, we can start the Flask app. Make sure you have activated a virtual environment first, and when we get to the endpoint, we will see the form is displayed, then fill in and submit the form.
Now, we can see that something got printed out.
If we change the form method to post
in the HTML code, we will no longer receive query strings. Instead, we will need to change the Flask code to access the request.form
where the Flask puts any form data received in a request.
<form action="#" method="post" >
We also have to tell the endpoint might receive get
and post
requests. If we want to access particular form data fields, we can use request.form.get()
.
This is a method that takes the name of the field that we want to access and returns its value, for example, request.form.get("candidate-name")
and request.form.get("candidate-city")
will give us their values.
from flask import Flask, request, render_template
app = Flask(__name__)
@app.route("/", methods=["GET", "POST"])
def INDEX():
print(request.form)
print(request.form.get("candidate-name"))
print(request.form.get("candidate-city"))
return render_template("index.html")
if __name__ == "__main__":
app.run(debug=True)
Output:
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn