Flask request.args.get
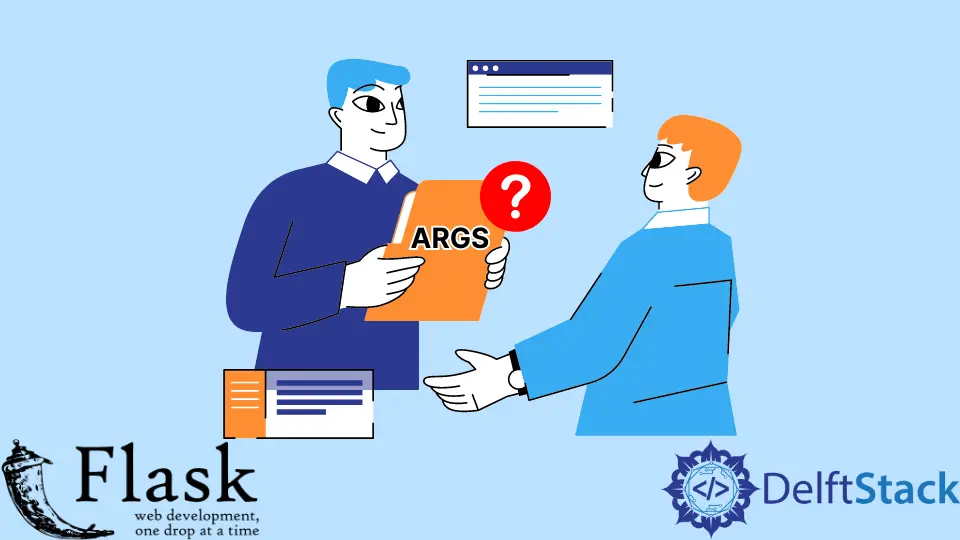
We will learn, with this explanation, how to work with request.args.get
. We will also learn the possible way to get an error when we use the request object and its attribute in Flask.
Use request.args.get
to Get Query String in Flask
When we require the client’s information in the request, we use the request
variable that is part of the Flask module. We can see that this is our view function, which does not take any arguments; it is just connected to the route of our application.
We use this request
variable that looks like a global variable but is not; it is imported from Flask.
What happens if this is a multi-threaded application and two requests come in simultaneously from different clients? The function will be running simultaneously in two different threads, and both are using a referencing request.
from flask import Flask, request
app = Flask(__name__)
app.config["SECRET_KEY"] = "NO_SECRETS"
@app.route("/")
def Main_Page():
Employee_Name = request.args.get("name")
return "<h2>Hello {}</h2>".format(Employee_Name)
if __name__ == "__main__":
app.run(debug=True)
Each thread will get the correct request
object. But this causes a lot of confusion, and many people do not understand how that works, so we will try to show you how the request
object works.
Possible Way to Get an Error When Using the request
Object in Flask
We will show some examples that people experience very obscure errors, and nobody understands what is sometimes happening. We open the Python shell, and if we import a request
from the Flask, there is no problem with it.
If we use the request.args.get()
to obtain an item from the query string of the URL using http://127.0.0.1:5000/?name=jack
, and when we use the Flask request
object inside the console, we get the famous error that is Working outside of request context
. This is one way in which people will get this error.
>>> from flask import Flask ,request
>>> request.args.get('name')
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "C:\python\lib\site-packages\werkzeug\local.py", line 432, in __get__
obj = instance._get_current_object()
File "C:\python\lib\site-packages\werkzeug\local.py", line 554, in _get_current_object
return self.__local() # type: ignore
File "C:\python\lib\site-packages\flask\globals.py", line 33, in _lookup_req_object
raise RuntimeError(_request_ctx_err_msg)
RuntimeError: Working outside of request context.
This typically means you attempted to use functionality that needed
an active HTTP request. Consult the documentation on testing for
information about how to avoid this problem.
Imagine that Flask is receiving a request from a client, and it needs to invoke your view function. It takes the information the web browser provides and then creates a request
object.
A request context is a structure that Flask maintains. It maintains one request context per thread.
Each thread will have a different request context; it puts the request object for that request in the context. The context is a data structure that is a list of objects, and when a new request arrives, Flask will invoke your view function.
Whenever we try to access an attribute, Flask invokes your view function to that request object that maps to the client request. Because each thread has a different request context structure, each thread will get its own servers.
When we use the request.args
out of the view function, it activates the proxy to see the request
object stored in the request context for this thread. And, of course, there is none of that because we are not running an application; that is why we are not receiving requests.
In this case, the Flask detects that we are asking to get a request
object out of a request context that does not exist. When we run our flask application and try to access the string query using request.args.get()
from the URL endpoint:
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn