Flask Project Structure
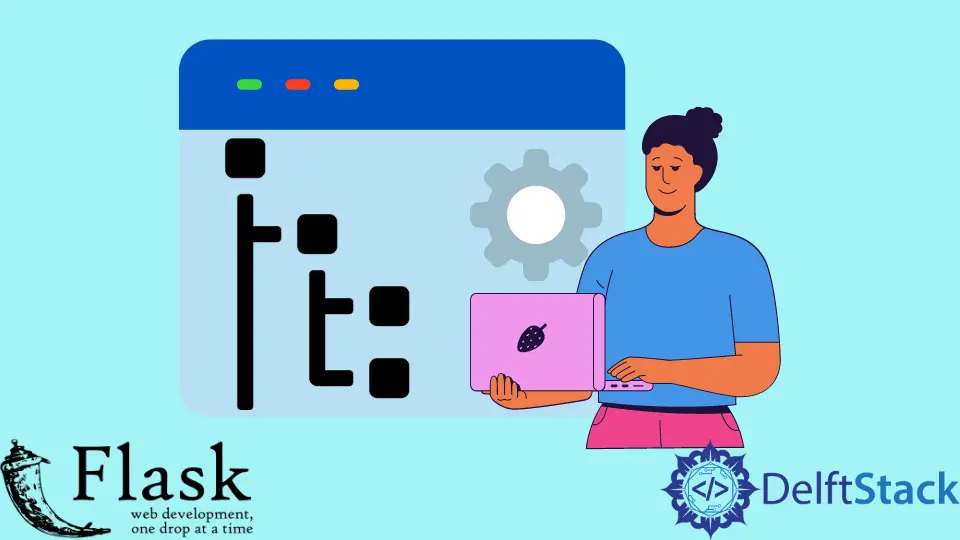
We will learn, with this explanation, how to Structure a big Flask application with the help of the Blueprint Approach in Flask and Python.
Structure a Big Flask Application With the Help of the Blueprint Approach in Flask and Python
We know that the Flask documentation has written about the Flask blueprint. Still, it is sometimes difficult for beginners to understand how to structure the Flask application by just reading the Flask documentation.
So we would like to break it down using simple words and explain how to structure the Flask application using the Flask Blueprint
class.
To start working, we will create a virtual environment and install a Flask using the following commands. After creating the virtual environment, we will activate this and install the Flask package.
python -m venv our_venv
.\our_venv\Scripts\Activate.ps1
pip install flask
The blueprint enables us to create different instances of the Flask application and reuse some of the code in the different packages so that we do not always have to write them from scratch.
First, we will create a directory called app
; inside the root
directory, we will create a file called run.py
. Inside the app directory, we will make this app directory to make a package in Python and create the __init__.py
file.
Now we will go ahead, and inside the __init__.py
file, we will import Flask and define the function create_app()
that will create the application. Now we will make the instance of Flask
and store it to the app
variable like this app=Flask(__name__)
and then return this object.
Now we have to import the create_app
function from the app, and we would like to run the application when we run this run.py
file. To do this, we will use the following syntax.
When creating an application, we will create different components that will work together to create the application; we can make that inside the app
directory. Now inside the app
directory, we create a directory called main
, and inside this main
directory, we will create the __init__.py
file, which will make it a package.
Now we will create a routes.py
file, and inside this file, we will import the Blueprint
class from the Flask module and give our blueprint a name something called main
, and pass it __name__
.
Basically, this is how we can create the simple Flask application, like as we instantiate the Flask application using the Flask
class.
main = Blueprint("main", __name__)
Now we will create a route for the routing page, define the Main_Page()
function, and then return the page.
Now inside the __init__.py
file that is inside of the app
directory, we need to register the blueprint, so we will import main
from the app.main.routes
where we have created the Blueprint instance. Then we will next register the blueprint, that is, main
using the app.register_blueprint()
.
In the same way, if we add the templates in the normal Flask application, we could create a directory called templates
, and then we can generate HTML files inside this directory. We can also control the configuration of the Flask application, which is very simple.
Inside the root
directory, we can create a config.py
file, and inside this file, we can create a class called Config
, which will have TESTING
and then DEBUG
set to False
.
We can also create another class here called Development
, which would inherit from Config
and overwrite DEBUG
to True
. We will define one more class called TESTING
which will also inherit from Config
; it will have the TESTING
property equal to True
.
You could have many configurations, but we only want to make this simple so that you can understand how to configure the blueprints application using configurations.
Now we are going to create a variable called config
that will be a dictionary, and this dictionary will have Development
and TESTING
classes as a value of those keys.
class Config(object):
TESTING = False
DEBUG = False
class Development(Config):
DEBUG = True
class TESTING(Config):
TESTING = True
config = {"development": Development, "testing": TESTING}
We will go back inside the __init__.py
file and import the config
dictionary from the Config
file. We will need to pass app_config
inside the create_app()
function and pass development to this argument.
Now we will need to pass the Flask app using the app.config.from_object(config[app_config])
and write this code inside the function block. Now we will install the dotenv
package that is useful to set up .env
files and variables because they can be used across the entire application and easily switch up.
pip install python-dotenv
Now we will also create a .env
file, and inside of this file, we will set the Flask environment from production to development. We only need to write this like the code FLASK_ENV=development
and save it.
We can create as many packages as we want, so we can now go inside the app directory and create an auth
package, and instead of this package, you can have your logins and your logout, and much more. We can also create a static
folder and keep your static asserts inside this directory as in the normal Flask app.
Now we will run this run.py
file we created initially. We can see the environment is currently in development mode rather than production, and the app is working properly.
This Blueprint Approach helps us to structure the application to make it much neater and compose a more extensive application.
The code of the run.py
file:
from app import create_app
if __name__ == "__main__":
create_app().run(debug=True)
The code of the __init__.py
file:
from flask import Flask
from config import config
def create_app(app_config="development"):
app = Flask(__name__)
app.config.from_object(config[app_config])
from app.main.routes import main
app.register_blueprint(main)
return app
The code of the routes.py
file:
from flask import Blueprint
main = Blueprint("main", __name__)
@main.route("/", methods=["GET"])
def Main_Page():
return "Hi there!"
Now our Flask project structure looks like this:
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn