How to Post Request in Python Flask
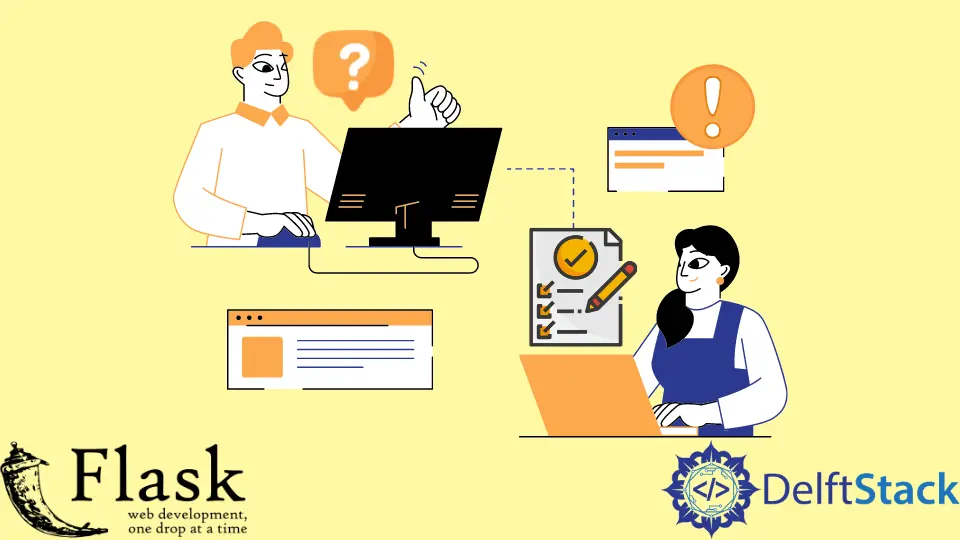
We will learn with this explanation about two basic HTTP methods (get
and post
), and we will also learn the differences between them and then go through a basic example of how we can send data from a basic form in the Flask.
Use Post
Request in Flask
We will explain the HTTP methods, get
and post
requests. You have probably heard both of these before; get
is the most common way of getting or sending information to a website or a client, depending on how this information is sent.
Post
is a way of doing this securely; get
essentially is an insecure way of getting information; it is the most commonly used. When we run our app and browse it then, we can see it is connected where we have the home page; if we go to the console where a statement pops up and says get
.
What does this mean? Essentially whenever we type something here is not secure, which means you know anyone can see it.
It is not secure information that will be sent to the server and returned us the actual web page using a get
method.
from flask import Flask
app = Flask(__name__)
@app.route("/")
def index():
return "Hi there, this is testing"
if __name__ == "__main__":
app.run(debug=True)
Output:
If we use the post
request, we can send secured information that is encrypted, and we won’t see it from either endpoint. It is not stored on the actual web server, so this is the difference between get
and post
.
We did not probably explain it in the best way, but through this article, we will understand the main differences between them and the basic way to think about the get
method that is used to send unsecured requests and post
requests is secured for sending data, it is usually used to send form data.
Let’s go through a basic example: we want to set up a few different pages in this app. We will write a script with a dialog box in which someone can type and send it using a button, and we do this while using the post
request.
We will set up a page using a method called LOGIN()
, give this a decorator at the top, and put "/login"
. We need to add another aspect we have not seen yet: the methods
.
We can use it on this login page, so by default, whenever you connect, or you go to one of the couple pages, then you go with a get
request which means we need to get that information, but it is not going to be secure that is why we have a different method that is post
method.
Inside the decorator, we need to use an argument called methods
, which takes a list, and then we put a post
and get
inside the list.
@app.route("/login", methods=["POST", "GET"])
The problem is how can we determine in this login
function whether we called the get
request or whether we call the post
request? We need to start importing the request
and using it with an if
statement to check whether we reach this page with a get
request or a post
request.
If we have a post
, we redirect the user and send the data to the user page, where we can display the data. We also use the Data
as a key of the form dictionary and access it from our login.html
file, where we have an attribute called name
.
If we have the get
request, we render the login page using the render_template()
method.
def LOGIN():
if request.method == "POST":
UER_DATA = request.form["DATA"]
return redirect(url_for("USER", usr=UER_DATA))
else:
return render_template("login.html")
Now we need to create one more page, and the function’s name would be USER()
, which will take a variable that is usr
. Instead of returning the template, we are going to return basic HTML.
@app.route("/<usr>")
def USER(usr):
return f"<h1>{usr}</h1>"
Now we need to build out this login page that would be a simple HTML form, so, inside the templates folder, we create a login.html
file; inside this file, we will use the Bootstrap starter template. Inside the body tag, we start creating the form.
We used the method
attribute, and in this case, our method is going to be post
because we are going to be posting information, not getting information, and usually, if you put get
in here, that means you are going to fill this form with information that you get from the server.
<h1>Hello, this is login page!</h1>
<form action="#" method="post">
<p>Name:</p>
<p><input type="text" name="DATA" /></p>
<p><input type="submit" value="submit"/></p>
</form>
Here is the complete source code of the app.py
file we explained above.
from flask import Flask, render_template, redirect, url_for, request
app = Flask(__name__)
@app.route("/")
def INDEX():
return render_template("index.html")
@app.route("/login", methods=["POST", "GET"])
def LOGIN():
if request.method == "POST":
UER_DATA = request.form["DATA"]
return redirect(url_for("USER", usr=UER_DATA))
else:
return render_template("login.html")
@app.route("/<usr>")
def USER(usr):
return f"<h1>{usr}</h1>"
if __name__ == "__main__":
app.run(debug=True)
Output:
Console output:
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn