How to Log Errors Using Flask
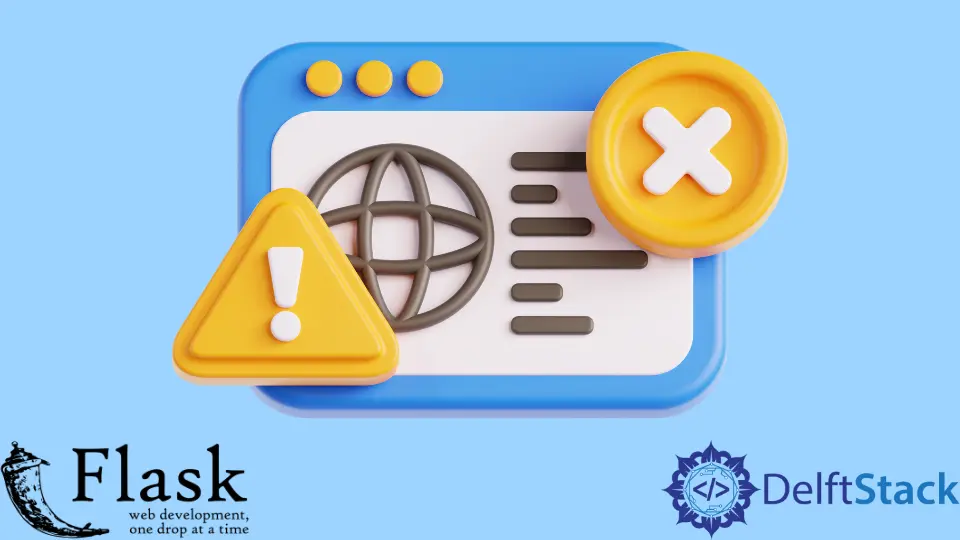
We will learn, with this explanation, about error handling, specifically logging errors. By the end of this article, you should know how to log errors to a file and where to go to check out other ways to log errors in Flask.
Log Errors With the Help of the Logging Module in Flask
Typically a production app will not be in debug mode, so the only way we can use the logging
module is to know there is an error. In this case, it will be sent to a file, so we can view it later, debug it, and fix the issue.
This is not enabled by default; we have to add a logging feature to our Flask app. That is why we will show you a really basic Flask app running, which is not in debug mode.
from flask import Flask
app = Flask(__name__)
@app.route("/")
def Error_Los():
return "The page without error"
if __name__ == "__main__":
app.run()
The first thing to use is the logging
module; we will need to import this. It is a built-in Python module, so we do not need to install it if we use Python 3.
We will import two things from the logging
module: FileHandler
and WARNING
.
FileHandler
is a way to log errors to a file. The WARNING
is the level at that we want to trigger the log; it could be something like an error where it is more serious.
But a warning is nice when we log into a file because we can log as much as we want.
from logging import FileHandler, WARNING
Now we will need to instantiate the FileHandler
, and we need to pass the file name where the error will be saved.
F_H = FileHandler("errorlogs.txt")
Now we will need to set the level of the file handler using setLevel()
; it is a method on the file FileHandler
class, so we pass the WARNING
level, which we imported from the logging
module.
Now we will need to use logger to our Flask app, and then we use addHandler
, and inside it is the file handler object we created above.
F_H.setLevel(WARNING)
app.logger.addHandler(F_H)
If we run this app, an errorlogs.txt
file will be created. And one thing you should note is that it only logs applications but not HTTP errors because it is not the type of error you are looking for; it is not an application.
Now we will try to return 1/0
, which will fail. We will run the server again and restart the page.
We will get an internal server error which means it is some error that HTTP cannot point to, but if we look in the log file, we see the error details.
def Error_Los():
return 1 / 0
Output:
If you want to learn more about the other handlers for error logging, visit the official documents from here.
Complete Python Code:
from flask import Flask
from logging import FileHandler, WARNING
app = Flask(__name__)
F_H = FileHandler("errorlogs.txt")
F_H.setLevel(WARNING)
app.logger.addHandler(F_H)
@app.route("/")
def Error_Los():
return 1 / 0
if __name__ == "__main__":
app.run()
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn