How to Get Query Parameters Using Flask
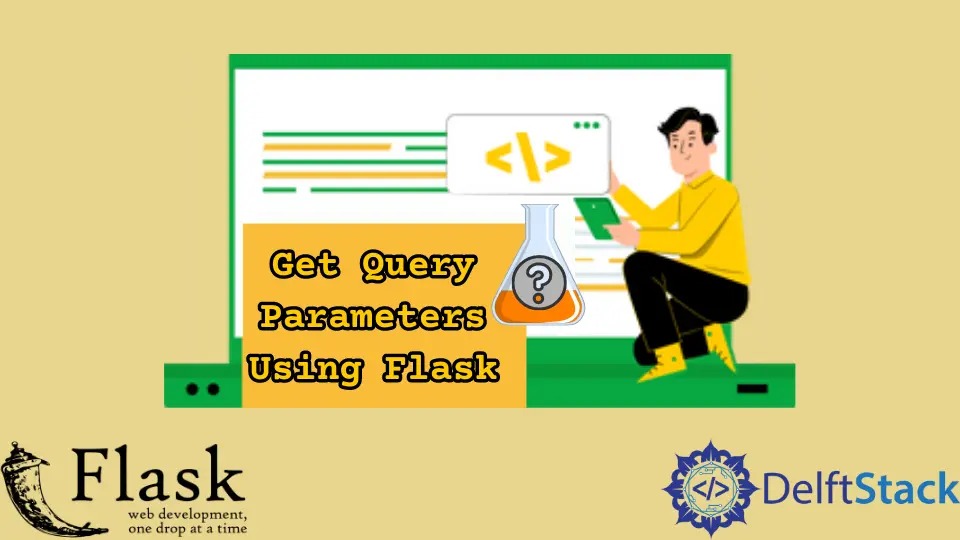
We will learn, with this explanation, how to get the parameters from the URL with the help of the request query string in Flask.
Extract the Parameter With the Help of Request Query String in Flask
Generally, we build a clean URL with our parameters in Flask, but sometimes we want a traditional query string for whatever reason. Our query strings are easy to work with; they are just separated by &
and the value on the right side.
Now we will show you exactly how to extract those, and we can use them somewhere in our application, so let’s get started. We will set up our Flask app, and we will import the Flask
class from the Flask module, and we also need to import the request variable and instantiate an object with the Flask
class.
from flask import Flask, request
We will define the main block, and inside this block, we will use the run()
method to run our app. Then we will put debug
equal to True
.
if __name__ == "__main__":
app.run(debug=True)
Now we will create a new route and call params
for this route, and the function will be named Get_Query_Params()
. We will return some strings to get the parameters out of the query string.
We will need to use the requests variable, define two arguments and access the query parameter. To get them, we only need to call requests.args
, a dictionary with all the parameters in the query string.
The first one will be called VAR1
, and the second one will be called VAR2
and store the dictionary values using the requests.args['key']
key. We are returning these dictionary items to display them on the page.
@app.route("/params")
def Get_Query_Params():
VAR1 = request.args["var1"]
VAR2 = request.args["var2"]
return f"Student name is: {VAR!} and Father nmae is: {VAR2}"
Let’s start the server, pass the parameter inside the route, and hit Enter to see the parameter displayed.
Now we can see we easily extract these values from the query string.
We will take one more example to access all the query parameters. We will create a variable called var1
, which will store the request.args
; this is a dictionary containing all the keys and values we provide as our query parameters.
Now we will apply a for
loop over the dictionary items and be able to access and print these keys and values.
@app.route("/")
def Get_Query_Params():
var1 = request.args
for key, value in var1.items():
print(key, value)
Using the keys, we can also access specific query parameters using if-else statements to check those actual query parameters exist. For now, the way we use to access the key is using var1.get()
instead of using request.args['key']
because get()
is the method that helps us to access the key.
if "nationality" in var1.keys():
print(var1.get("nationality"))
return f'Age is {var1.get("age")} and nationality is {var1.get("nationality")}'
Now we save and start the server, go to the browser, and provide the key-value pair inside the route. When we run this page, then we can see the parameters displayed, as well as we can see in the console where keys and values are printed.
It is very similar to getting the data from a form; if someone submits a form, we can extract it from an endpoint.
Full Python Code:
from flask import Flask, request
app = Flask(__name__)
# @app.route('/params')
# def Get_Query_Params():
# VAR1=request.args['var1']
# VAR2=request.args['var2']
# return f'Student name is: {VAR1} and Father nmae is: {VAR2}'
@app.route("/")
def Get_Query_Params():
var1 = request.args
for key, value in var1.items():
print(key, value)
if "nationality" in var1.keys():
print(var1.get("nationality"))
return f'Age is {var1.get("age")} and nationality is {var1.get("nationality")}'
if __name__ == "__main__":
app.run(debug=True)
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn