How to Download a File Using Flask
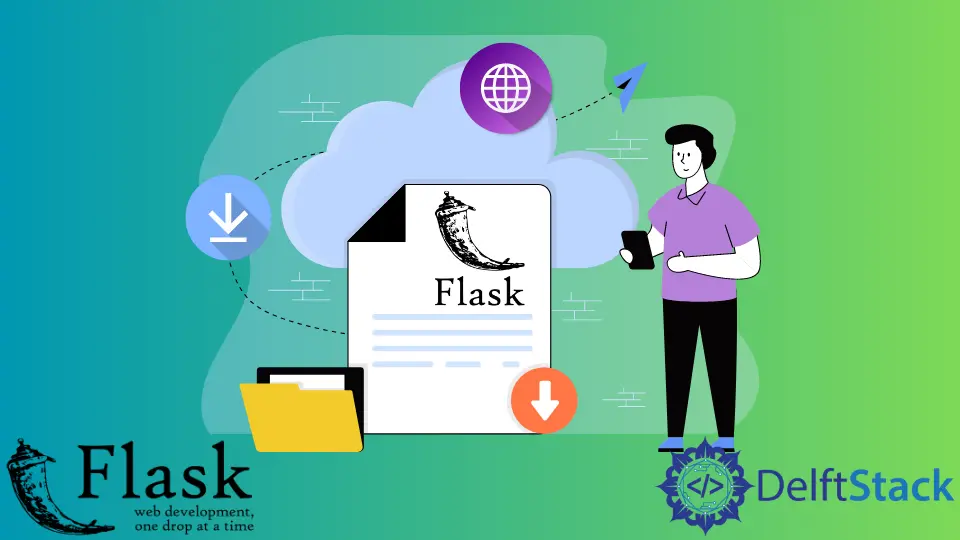
We will learn, with this explanation, how to make a downloadable link to download a file as an attachment using the send_file
function in Flask.
Download a File as an Attachment With the Help of the send_file
Function in Flask
In the Flask Framework, we can make a file downloader to download all kinds of files like PDF, JPEG, MP3, or other files, but the logic remains constant. Now we only need to go to our text editor and create a simple app.py
file inside the same directory where our PNG file is located.
Now we also need to create a templates folder, and inside this, we will create an index.html
file, and that’s it. Now, first of all, we need to import the Flask library.
You only need some required libraries and the send_file
library:
from flask import Flask, render_template, send_file
We need to create an app
variable and initialize the Flask application using the __name__
attribute. Now we will initialize our main function, and we only need to call the run
function inside this block and pass it debug
equals to True
, so it will restart the application whenever we make changes.
app = Flask(__name__)
if __name__ == "__main__":
app.run(debug=True)
We must create a route and a function to load the HTML file. For this, we will create a home route that is /
, and when we hit this route, it will call a function that would be Main_Page()
.
This function returns render_template()
; inside this, we will pass the index.html
file, which will load the template stored inside the index.html
file.
@app.route("/")
def Main_Page():
return render_template("index.html")
Now we will write a basic template that will hold the downloaded file.
We will use just a few tags, the first will be a <h2>
tag that will hold the heading, and the second one is a <p>
tag that is a paragraph tag. Inside this tag, we will use the anchor tag, and the href
attribute will be equal to a property which is url_for
, and pass it its name.
<h2>Downloqad this file</h2>
<p> <a href="{{url_for('Download_File')}}">Download</a></p>
If we run this application and open localhost 5000
, it will tell us that it could not build a URL for the endpoint Download_File
.
This syntax url_for('Download_File')
is looking for a download file, so we only need to make a get request to do this.
Now we will create another route, /download
. And we will create a function with the same name we specified inside the url_for()
property.
Now we will need to specify the file’s path, so we will create a variable that would be PATH
and store the path inside this variable.
We will return the file using the send_file()
function, which takes two parameters. The first will be a path, and the second will be as_attachment
equals to True
because we want to make a downloadable file as an attachment.
@app.route("/download")
def Download_File():
PATH = "Flask-logo.png"
send_file(PATH, as_attachment=True)
If we save and refresh the page, we will see that it is converted to a hyperlink, and we can download the file by clicking on this link.
Complete Python Code:
from flask import Flask, render_template, send_file
app = Flask(__name__)
@app.route("/")
def Main_Page():
return render_template("index.html")
@app.route("/download")
def Download_File():
PATH = "Flask-logo.png"
return send_file(PATH, as_attachment=True)
if __name__ == "__main__":
app.run(debug=True)
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn