How to Display an Image in Flask App
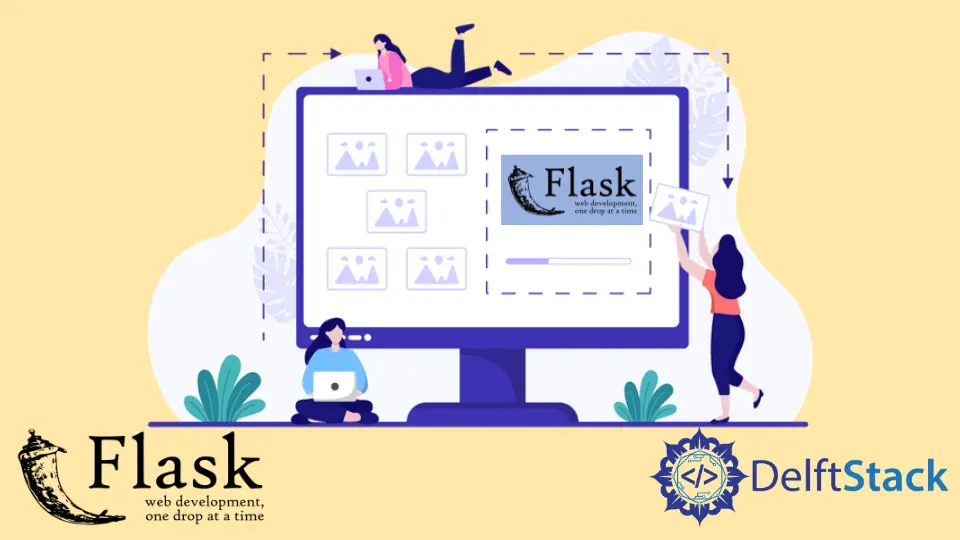
We will learn, with this explanation, how we can add an image to a web page and how we can upload or display multiple images in the Flask app.
Display an Image in the Flask App
In this section, we will be uploading an image on our web page, and the first step is to create two initial directories, static
and templates
. We will create a new directory that will hold our images inside the static
folder.
The next step is to get the address of this IMG
folder we created inside the static
folder. So we have to import the os
library and use it to save the IMG
folder path in a new variable called IMG_FOLDER
.
IMG_FOLDER = os.path.join("static", "IMG")
We will need to pass this address to the application configuration upload folder. So we have to go inside UPLOAD_FOLDER
and assign the address where we want to look at the images.
app.config["UPLOAD_FOLDER"] = IMG_FOLDER
In the next step, we will join the path using UPLOAD_FOLDER
with an image name we want to display on the web page. We will store this address in the Flask_Logo
variable inside the Display_IMG()
function.
Flask_Logo = os.path.join(app.config["UPLOAD_FOLDER"], "flask-logo.png")
Next, we will need to define an argument inside the render_template()
and pass the Flask_Logo
variable to this as a value.
from flask import Flask, render_template
import os
app = Flask(__name__)
IMG_FOLDER = os.path.join("static", "IMG")
app.config["UPLOAD_FOLDER"] = IMG_FOLDER
@app.route("/")
def Display_IMG():
Flask_Logo = os.path.join(app.config["UPLOAD_FOLDER"], "flask-logo.png")
return render_template("index.html", user_image=Flask_Logo)
if __name__ == "__main__":
app.run(debug=True)
Now we will need to go to the templates
folder and create an index.html
file, and inside this file, we will write basic HTML code. We will only pass the user_image
variable in the img
tag we defined in the render_template()
.
This is the source code used in the HTML file for this example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Hello Worl!</title>
</head>
<body>
<h1>Hello World!</h1>
<center><h3>Flask Logo</h3><img src="{{ user_image }}" alt="Mike" height="240px" width="300px"></center>
</body>
</html>
When we run this app, we shall see the image displayed properly.
Display Multiple Images in the Flask App
Now we will be adding multiple images from the IMG
folder and showing you how you can display all of them, so let’s start by making a list of the images. We will create this list inside the function that will hold all assets of a directory using the listdir()
function, and the list name will be IMG_LIST
.
IMG_LIST = os.listdir("static/IMG")
Now we will create a loop for all the images, and we will use list comprehension and store it in the same variable called IMG_LIST
.
from flask import Flask, render_template
import os
app = Flask(__name__)
IMG_FOLDER = os.path.join("static", "IMG")
app.config["UPLOAD_FOLDER"] = IMG_FOLDER
@app.route("/")
def Display_IMG():
IMG_LIST = os.listdir("static/IMG")
IMG_LIST = ["IMG/" + i for i in IMG_LIST]
return render_template("index.html", imagelist=IMG_LIST)
if __name__ == "__main__":
app.run(debug=True)
The next step is to make some changes to the HTML file. Instead of passing them one by one, we will display them through the looping using an imagelist
variable that is a list of multiple images.
Now we will put an img
tag, and inside this tag, we will use the url_for
tag to get static files. url_for()
will take two attributes: one will be static
, and the second will be the filename
, and we’ll pass it an item i
that we are iterating through the loop.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Hello Worl!</title>
</head>
<body>
<h1>The Multiple Images</h1>
{% for i in imagelist %}
<img src = "{{ url_for('static', filename=i)}}">
{% endfor %}
</body>
</html>
When we run the server and go to the browser, we can see the multiple images displayed here.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn