How to Create Route in Flask App
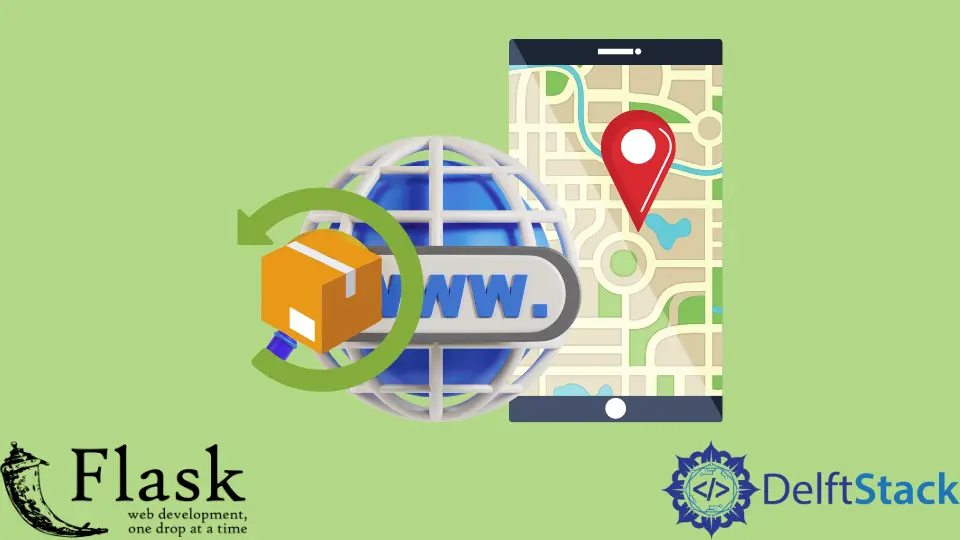
We will learn how we can create a route in Flask and how we get a response when we call the route in Flask.
Create a Page With the Help of app.route()
Decorator in Flask
The way that we can route is through the use of a decorator, so what is a decorator? Well, whenever we see an @
symbol with a function something like this @app.route('/')
, that is a decorator.
It is a way that you can wrap up an existing Python function and modify its behavior. We route or map a URL to a return value, and whenever a user requests a URL, the server’s response will be whatever function’s return value.
We will show you some examples that make a lot more sense, so let’s create a route.
@app.route('/fund-api')
Now we will define a function called Fund_Func()
so whenever the user goes to the page that is usually going to be our URL like /fund-api
, then the function will return all content that is wrapped under this function. We are going to return HTML <h2>
tag.
def Fund_Func():
return "<h2>Your fund is returned</h2>"
When we save and run this server, we can visit our index page and go ahead and write fund-api
inside the URL, which means we request the server to give the Fund_Func
page and check if the request is valid or not. If the route is valid, then it will run that function that exists inside this route.
There are better ways to include HTML using render_template()
. Another thing that we do is use variables inside the route.
Let’s say we are setting up our web page, and inside this webpage, we have a profile section, and we want to know which user is using its profile.
We will need to join the variable to be part of this URL, and depending on that variable, the content changes. Now we are going to create a new route, and inside the route, we will add a variable called candidate_name
and put it between angle brackets.
Now we will pass a parameter inside the function we are using inside the route, then we can return this variable with whatever we want to display on the screen. We will pass this variable from the URL.
@app.route("/fund-user-api/<candidate_name>")
def Fund_Func_Var(candidate_name):
return "<h2>Hi %s,your fund is returned</h2>" % candidate_name
When we pass the variable value with the URL, it will return the response with a parameter we passed, and if we do not pass the parameter value, it will raise an error because this is also part of the URL.
Complete Python code:
from flask import Flask
app = Flask(__name__)
@app.route("/")
def index():
return "The main page"
@app.route("/fund-api")
def Fund_Func():
return "<h2>Your fund is returned</h2>"
@app.route("/fund-user-api/<candidate_name>")
def Fund_Func_Var(candidate_name):
return "<h2>Hi %s,your fund is returned</h2>" % candidate_name
if __name__ == "__main__":
app.run(debug=True)
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn