How to Submit Ajax in Python Flask
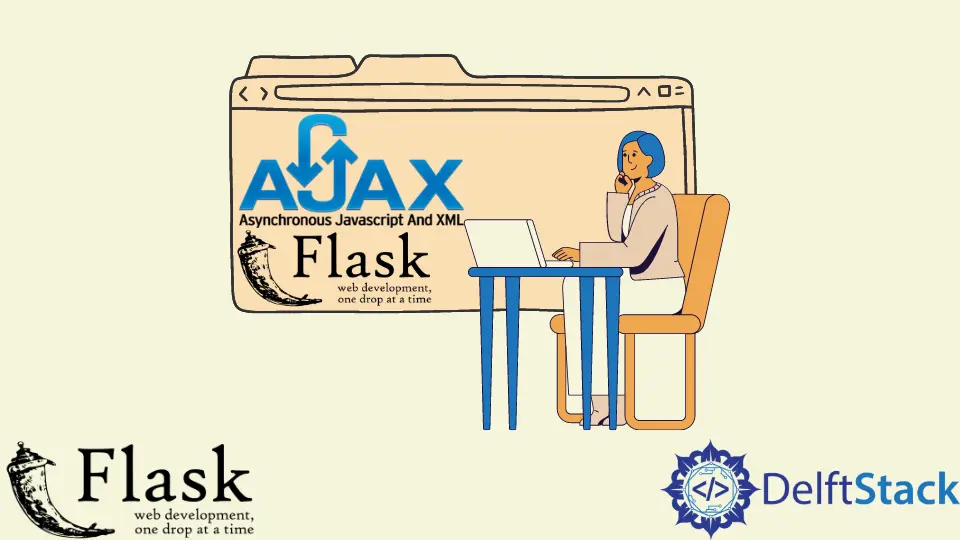
We will learn, with this explanation, how to submit an AJAX form with the help of jQuery and Flask, where jQuery handles the AJAX part and Flask accepts the AJAX request.
Submit an AJAX Form With the Help of jQuery and Flask
Now, we will create an app.py
file and get into actually writing the code. So the first thing we want to handle is server-side.
Now, we will add a route called post-data
that will take the data that has passed from the AJAX request, and we will only accept post requests. We will define a function called Post_DATA()
, and inside this function, we will need to get the data that will be passed from the form.
Typically, an AJAX will be in the form of a dictionary and the request object.
We will create the first object called Employee_Email
and store the request.form['Employee_Email']
, which means we are looking for data from the form field. Now, we will do the same thing with another object called Employee_Name
.
Employee_Email = request.form["Employee_Email"]
Employee_Name = request.form["name"]
Now, we will process this data, and if both Employee_Email
and Employee_Name
exist, we will create a New_EMP_Name
. What we are going to do is reverse the Employee_Name
using Employee_Name[::-1]
.
Now, we will return a JSON object that is New_EMP_Name
using the jsonify()
function, and if the block is not executed, then we will return a JSON object with the key error
and its value.
if Employee_Name and Employee_Email:
New_EMP_Name = Employee_Name[::-1]
return jsonify({"Employee_Name": New_EMP_Name})
return jsonify({"error": "Missing some data!"})
When this form gets submitted, we will need the event for submitting it; that is why we will handle it from jQuery.
Generally, in HTML, if you have a form and hit submit, it will try to post the data by itself. It is a built-in HTML feature; you do not need JavaScript to submit a form.
Since we are using jQuery to submit the data, we will need an event. The event
is the parameter in the anonymous function, and then event.preventDefault()
will be placed in the last to prevent the form from submitting the data twice.
$(document).ready(function() {
$('form').on('submit', function(event) {
event.preventDefault();
});
});
Within the function, we need to call ajax
using the dollar sign and construct the arguments; the data will contain two objects, Employee_Email
and Employee_Name
. We will trigger the data by the corresponding input id.
Now, we need to specify the type
of request, and it needs to be a POST
because, in our Flask code, we are only accepting post requests, and then the URL will be the route that is /post-data
.
$.ajax({
data:
{Employee_Name: $('#input_n').val(), Employee_Email: $('#input_e').val()},
type: 'POST',
url: '/post-data'
})
Now, we will create a done
function, and inside this function, we will create an if-else
statement to check and see if the error occurs or not. We are accessing the alert box id from the HTML file, and when an error occurs, this will select the alert for the error message and then hide the successful alert.
If the if
condition will not be true, then we go to the else
block, do the exact opposite, populate the success alert with the text data from this JSON object, and then we want to hide the error alert.
.done(function(data) {
if (data.error) {
$('#errorAlert').text(data.error).show();
$('#successAlert').hide();
} else {
$('#successAlert').text(data.Employee_Name).show();
$('#errorAlert').hide();
}
Now, let’s run the Flask app and navigate the app route. Inside the form, we will provide an email, then a name, and after submitting, we can see it returns the name in reverse order without reloading the page.
Complete Python Code:
from flask import Flask, request, jsonify, render_template
app = Flask(__name__)
@app.route("/")
def Main_Page():
return render_template("index.html")
@app.route("/post-data", methods=["POST"])
def Post_DATA():
Employee_Email = request.form["Employee_Email"]
Employee_Name = request.form["Employee_Name"]
if Employee_Name and Employee_Email:
New_EMP_Name = Employee_Name[::-1]
return jsonify({"Employee_Name": New_EMP_Name})
return jsonify({"error": "Missing some data!"})
if __name__ == "__main__":
app.run(debug=True)
Complete JavaScript Code:
$(document).ready(function() {
$('form').on('submit', function(event) {
$.ajax({
data: {
Employee_Name: $('#input_n').val(),
Employee_Email: $('#input_e').val()
},
type: 'POST',
url: '/post-data'
}).done(function(data) {
if (data.error) {
$('#errorAlert').text(data.error).show();
$('#successAlert').hide();
} else {
$('#successAlert').text(data.Employee_Name).show();
$('#errorAlert').hide();
}
});
event.preventDefault();
});
});
Complete HTML Code:
<!DOCTYPE html>
<html>
<head>
<title>Flask Form</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.1.0/jquery.min.js"></script>
<!-- Latest compiled and minified CSS -->
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css" integrity="sha384-1q8mTJOASx8j1Au+a5WDVnPi2lkFfwwEAa8hDDdjZlpLegxhjVME1fgjWPGmkzs7" crossorigin="anonymous">
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/js/bootstrap.min.js" integrity="sha384-0mSbJDEHialfmuBBQP6A4Qrprq5OVfW37PRR3j5ELqxss1yVqOtnepnHVP9aJ7xS" crossorigin="anonymous"></script>
<script src="{{ url_for('static', filename='index.js') }}"></script>
</head>
<body>
<div class="container">
<br><br><br><br>
<form class="form-inline">
<div class="form-group">
<label class="sr-only" for="input_e">Email address</label>
<input type="email" class="form-control" id="input_e" placeholder="Email">
</div>
<div class="form-group">
<label class="sr-only" for="input_n">Name</label>
<input type="text" class="form-control" id="input_n" placeholder="First Name">
</div>
<button type="submit" class="btn btn-default">Submit</button>
</form>
<br>
<div id="successAlert" class="alert alert-success" role="alert" style="display:none;"></div>
<div id="errorAlert" class="alert alert-danger" role="alert" style="display:none;"></div>
</div>
</body>
</html>
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn