How to Change the Default Port in Flask
-
Specify Port in
app.run()
Method to Change the Default Port in Flask - Use Command-Line Arguments to Change the Default Port in Flask
- Set Environment Variables to Change the Default Port in Flask
- Configure in Python File to Change the Default Port in Flask
- Conclusion
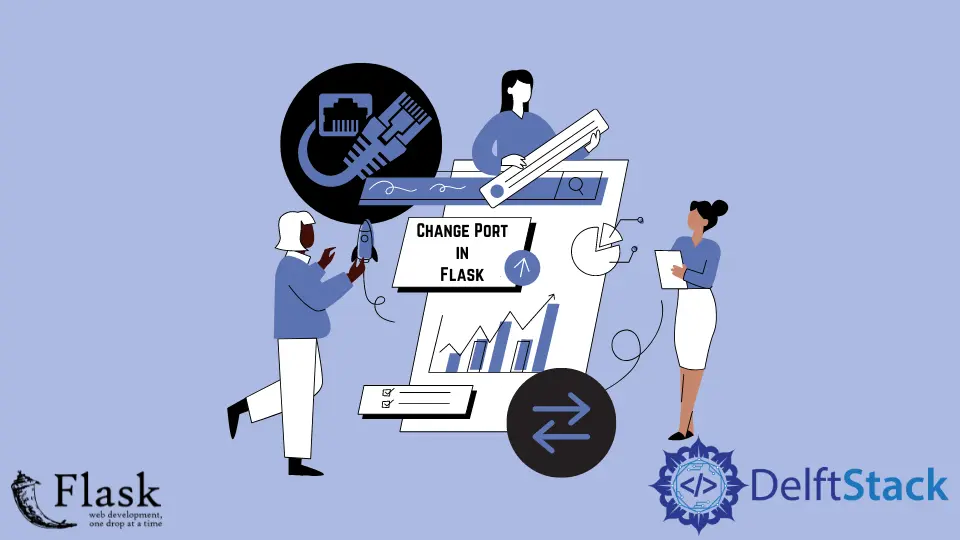
Flask, a lightweight and versatile web framework for Python, is often used for developing web applications. By default, Flask runs on port 5000
. However, there are scenarios where you may want to change this default port.
In this article, we’ll explore different methods to change the default port in Flask.
Specify Port in app.run()
Method to Change the Default Port in Flask
The most straightforward way to change the default port is by providing a port
argument to the app.run()
method.
The app.run()
method is used to run a Flask application. By default, it listens on port 5000
, but it can be customized to listen on a different port.
Syntax:
app.run(host=None, port=None, debug=True, options)
host
: This specifies the host that the application should listen on. By default, it’s set to'127.0.0.1'
, which means it will only accept connections from the local machine. To allow connections from any network interface, you can set it to'0.0.0.0'
.port
: This sets the port on which the application will run. The default is5000
.debug
: This is a Boolean value that enables or disables debug mode. When debug mode is enabled, the server will automatically restart after you make changes to your code. It’s very useful during development. The default value isFalse
.options
: This is an optional parameter that allows you to pass additional options to the underlying server. These options depend on the server you’re using (e.g., Werkzeug, Gunicorn, etc.).
Here’s an example of using the app.run()
method to change the default port in Flask.
from flask import Flask
app = Flask(__name__)
@app.route("/")
def hello_world():
return "Hello, World!"
if __name__ == "__main__":
app.run(port=8000)
Here’s a breakdown of the code:
The from flask import Flask
imports the Flask
class from the Flask package, allowing you to create a new Flask application.
app = Flask(__name__)
creates a new instance of the Flask application. The __name__
parameter is a special Python variable that is automatically set to the name of the current module. Flask uses it to determine the root path of the application.
Next, @app.route('/')
is a decorator that defines a route for your application. In this case, it specifies that the function hello_world()
should be called when a request is made to the root URL '/'
.
def hello_world()
is the function that will be called when a request is made to the root URL. It returns the string 'Hello, World!'
.
if __name__ == '__main__':
is a conditional statement that ensures that the development server only starts if the script is executed directly (not imported by another script). This is a common practice in Flask applications.
Lastly, app.run(port=8000)
runs the Flask application. Since you’ve specified the port
parameter as 8000
, the application will listen for incoming requests on port 8000
.
Once the application is running, you should be able to access it by visiting http://localhost:8000
in your web browser. You should see the message "Hello, World!"
displayed on the page.
Output:
Use Command-Line Arguments to Change the Default Port in Flask
Python’s argparse
module is a standard library for parsing command-line arguments. It provides a user-friendly interface for specifying and handling arguments.
Let’s see how we can use argparse
to change the default port in your Flask application.
Here’s an example (app.py
):
from flask import Flask
import argparse
app = Flask(__name__)
@app.route("/")
def hello():
return "Hello, World!"
if __name__ == "__main__":
parser = argparse.ArgumentParser(
description="Run the Flask application with a custom port."
)
parser.add_argument(
"--port", type=int, default=5000, help="Port number (default is 5000)"
)
args = parser.parse_args()
app.run(debug=True, port=args.port)
Here’s a summary of the code:
The code begins by importing the Flask
class from the Flask package and the argparse
module.
Then, parser = argparse.ArgumentParser(description=' ')
creates an instance of the ArgumentParser
class from argparse
. It sets the description that will be displayed when the script is run with the --help
option.
parser.add_argument('--port', type=int, default=5000, help='Port number (default is 5000)')
adds an argument to the parser. In this case, it defines a --port
option that expects an integer. If no port is provided, it defaults to 5000
. The help
parameter describes what the argument does.
The args = parser.parse_args()
line parses the command-line arguments provided when the script is run. It extracts the values of the arguments defined earlier.
app.run(debug=True, port=args.port)
runs the Flask application. The debug=True
argument enables debug mode, which automatically restarts the server when changes are made to the code. The port=args.port
argument sets the port on which the application will run. This value is obtained from the command-line arguments.
To run your Flask application with a custom port, open a terminal/command prompt and use the following command:
python app.py --port 5001
This command will run your Flask application on port 5001
. If you don’t specify a port, it will default to 5000
.
Output:
By using command-line arguments, you can easily change the port without modifying the code of your Flask application.
Set Environment Variables to Change the Default Port in Flask
You can change the port of a Flask application using environment variables. This allows you to configure these settings dynamically without modifying your code.
First, you need to modify your Flask application code to read the port from environment variables.
Here’s an example (app.py
):
import os
from flask import Flask
app = Flask(__name__)
@app.route("/")
def hello():
return "Hello, World!"
if __name__ == "__main__":
# Get the PORT environment variable or use the default value (5000)
port = int(os.environ.get("PORT", 5000))
app.run(debug=True, port=port)
In this example, we’re using os.environ.get()
to retrieve the value of the PORT
environment variable. If the PORT
environment variable is not set, it will default to port 5000
.
The port=port
argument sets the port on which the application will run. This value is obtained from the environment variable or defaults to 5000
.
Before running the application, we need to set the PORT
environment variable.
Linux/Unix/macOS:
Open your terminal and set the PORT
environment variable.
export PORT=5001
Windows:
Open a Command Prompt and set the PORT
environment variable.
set PORT=5001
Regardless of your operating system, you can now run your Flask application.
python app.py
Your Flask application will start and run on the port specified by the PORT
environment variable (in this example, it will run on port 5001
).
Output:
If you use this method, please take note of the following:
- Set the
PORT
environment variable to the desired port before running your Flask application. This way, you can easily change the port without modifying the code. - If you’re using an IDE or a development environment like VS Code, there might be specific settings within the IDE to configure the environment variables. Consult the documentation of your specific development environment if you encounter any issues.
- If the specified port is already in use by another application, you’ll need to choose a different one.
Configure in Python File to Change the Default Port in Flask
You can configure the port in a Python configuration file. This approach allows you to centralize your configuration options in one file (config.py
).
If you ever need to change the port, you can do so in config.py
without modifying your main application code. This separation makes it easier to manage configuration settings, especially in larger applications.
Create a file named config.py
and define a class with configuration options. In this case, we’ll set the FLASK_RUN_PORT
variable to the desired port, such as 8000
.
class Config:
FLASK_RUN_PORT = 8000
In your main Flask application file (app.py
), import the Flask framework, create an instance of the Flask application, and load configuration settings from the Config
class defined in the config.py
file.
from flask import Flask
app = Flask(__name__)
app.config.from_object("config.Config")
@app.route("/")
def hello_world():
return "Hello, World!"
In this example, we created two Python files: config.py
to store configuration variables and app.py
to import Flask and set the app.config.from_object()
to point to the configuration class.
Your Flask application will now run on the port specified in config.py
.
Output:
Conclusion
In conclusion, Flask typically runs on port 5000
by default. However, there are various methods to change this default port.
One approach is to specify the port directly in the app.run()
method within your Flask application code. Another method involves using the command-line interface to pass a custom port when running the application.
Additionally, you can utilize environment variables to dynamically configure the port without modifying your code. Finally, you can centralize your configuration options in a Python file (config.py
), allowing easy management of settings, particularly in larger applications.
By implementing these methods, you can seamlessly adapt the port to suit your specific needs in Flask development.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn