How to Send Data in URL to View the Single Record of a Selected Product
- Select All Products From the Database Table
-
Use the
details.php?pid=(dynamic value)
to Send Data in URL - Use PODS Style MySQL to Send ID in URL
- Understand PODS to Send Data in URL
- Fetch Records Against the Selected Product ID (Pid)
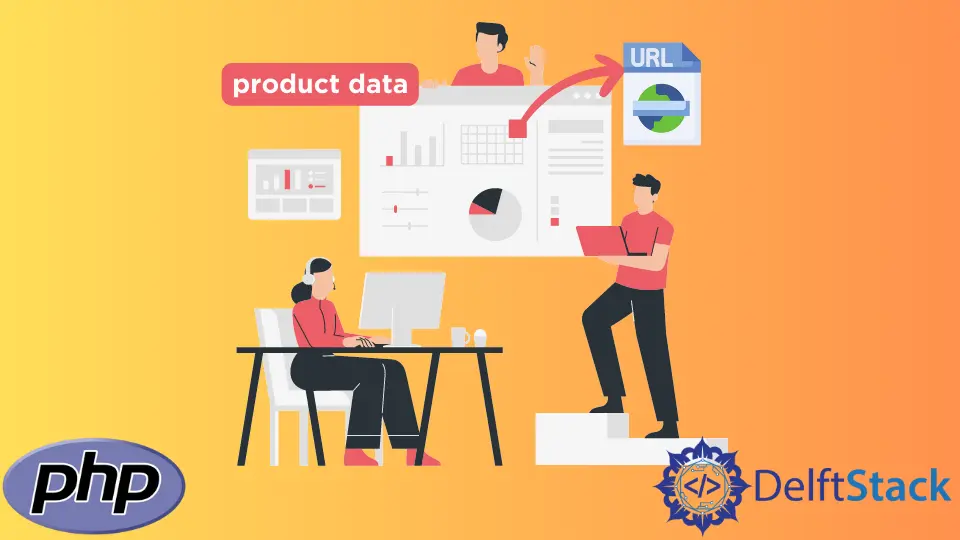
In this article, we will discuss how to send data in URL to view the single record of a selected product.
Select All Products From the Database Table
The code below explains how to fetch data from the MySQL database and create a myproducts.php
page.
<!DOCTYPE html>
<body align='center'>
<head>
<title> View product detail </title>
<link rel="stylesheet" href="style.css">
</head>
<form action="myproducts.php" method="post" align="center">
<input type="submit" name="select" value="Show all products"/>
</form>
<?php
//your database connection
//The database file is included in the tutorial directory
require_once ('db.php');
//On submit
if (isset($_POST['select']))
{
//execute the mysql_query(); query to select all records from the cart table
//You can also find the sql dump file for the tabe in the directory
$response = mysqli_query($connect, 'SELECT * FROM cart');
echo "<table border='2' align='center'>
<H2 align='center'> All Products </h2>
<tr>
<th>ID</th>
<td>Product Name</td>
<td>Price</td>
<td>Quantity</td>
<td>IP Rating</td>
</tr>";
//fetch records and store in the array using mysqli_fetch_array(); function
while ($fetch = mysqli_fetch_array($response));
{
echo "<tr>";
//echo array['index'] that contains product id which is a primary key
//You will use this id later to dynamically retrieve records for the selected id which is now printed in the hyper reference
//we are also using rawurlencode(); function for security which encodes your sensitive id information.
?><td><a href="details.php?pid=<?php echo rawurlencode($fetch['id']); ?> /">
<?php echo $fetch['id']; ?> </a> </td>
<?php
//fetch data from the cart table and create dynamic table in this while loop
echo "<td>" . $fetch['productname'] . "</td>";
echo "<td>" . $fetch['price'] . "</td>";
echo "<td>" . $fetch['quantity'] . "</td>";
echo "<td>" . $fetch['IPrating'] . "</td>";
echo "</tr>";
}
echo "</table>";
mysqli_close($connect); // close database connection
}
?>
</body>
</html>
Output:
Use the details.php?pid=(dynamic value)
to Send Data in URL
We can use any table property as your ID, including string. We can echo it inside the HTML element and then use $_GET['pid'];
method to retrieve it.
The rest is up to the requirements regarding how we would want to manipulate the dynamic ID.
<!DOCTYPE html>
<body align='center'>
<a href='myproducts.php'> Main Page </a>
<head>
<title> URL ID FETCH SINGLE RECORD FROM PRODUCTS </title>
<link rel="stylesheet" href="style.css">
</head>
Use PODS Style MySQL to Send ID in URL
From the myproducts.php
file, we learnt how to do something like:
<a href="details.php?pid=<?php echo rawurlencode($fetch['id']); ?> /">
<?php echo $fetch['id']; ?> </a>
This is the most common method used in PHP to make URLs dynamic and send ID(s).
Once we have created dynamic, hyper references, we are all set to use $_GET['id'];
and store it in a variable. That way, we can use any logic on this selected ID.
Since it comes from within the loop structure, it changes ID as the data gets fetched through mysqli_fetch_array();
.
Code (products.php
):
<?php
require "db.php";
//get your id from the URL
$id=$_GET['pid'];
$query="SELECT * FROM cart where id=?";
if($param = $connect->prepare($query))
{
$param->bind_param('i',$id);
$param->execute();
$response = $param->get_result();
$response->num_rows;
$field=$response->fetch_object();
}
?>
Understand PODS to Send Data in URL
The most important part to note here is using the PODS-styled database method.
Check the SQL query. It is different from the one we used in MySQL because it is PODS.
In a nutshell, we used bind_Param();
, a PHP function that connects a parameter to the identifier of a variable in a SQL query.
The get_result();
produced an array. As a result, each element correlates to one row of the query result.
The fetch_object();
returned the current row of a result set while the num_rows();
returned the number of rows in the result set.
<h3 align="center"> Selected product </h3>
<table align='center' border='2'>
<th> Id</th>
<th> Product Name</th>
<th> Price</th>
<th> Quantity</th>
<th> Other</th>
Fetch Records Against the Selected Product ID (Pid)
In the code below, we have used the PODS structure to fetch array indexes that contain data against product.php?pid='dynamic id'
.
<?php
echo "<tr ><td>$field->id</td>
<td>$field->productname</td>
<td>$field->price</td>
<td>$field->quantity</td>
<td>$field->IPrating</td></tr>
";
echo "</table>";
}else{
echo $connection->error;
}
?>
</body>
</html>
Output:
Sarwan Soomro is a freelance software engineer and an expert technical writer who loves writing and coding. He has 5 years of web development and 3 years of professional writing experience, and an MSs in computer science. In addition, he has numerous professional qualifications in the cloud, database, desktop, and online technologies. And has developed multi-technology programming guides for beginners and published many tech articles.
LinkedIn