How to Run Shell Scripts in PHP and Open Shell File
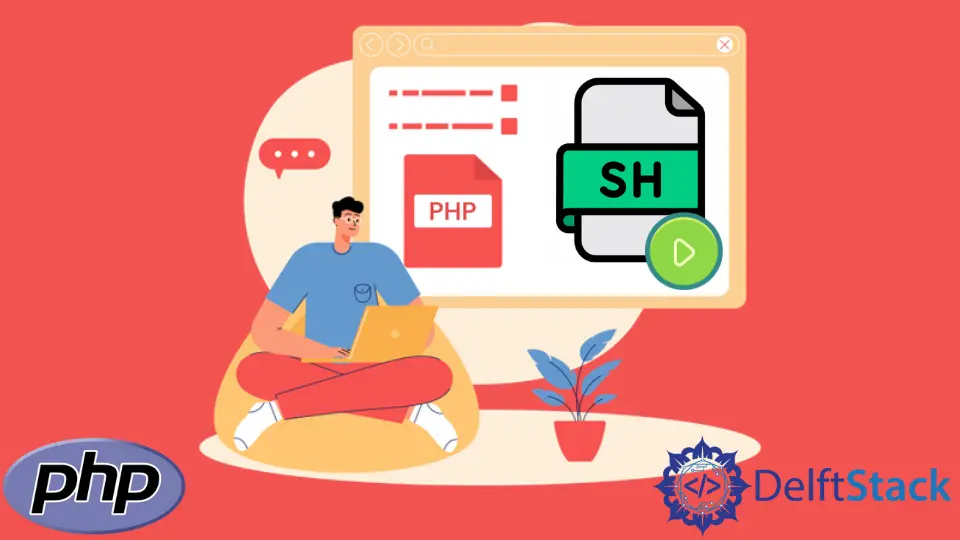
PHP allows us to use the shell_exec();
function to deal with shell files. However, if your OS is Windows, you should consider using popen()
and pclose()
functions because the pipes are executed in text mode, which usually keeps it from binary outputs.
We will implement two scripts in our shell.php
file. First we will open the .sh
file using shell_exec();
function.
Then, we will use shell_exec()
to open the cmd interface and run a few windows commands.
Run Shell File in Text Mode Using shell_exec()
Function syntax and parameters: shell_exec(string $cmd);
. This function returns shell output in the string format.
We have created a demo demo.sh
file in this tutorial.
Code (demo.sh
):
#!/bin/sh
echo "Hello world";
echo "This is a shell .sh file for demo";
// your shell commands go here
You can create a .sh
file using any text editor and save it with a .sh
file extension. After that, please run the following PHP script (shell.php
) to open it in Notepad because it will throw string format in text mode.
<!DOCTYPE html>
<head>
<title>
Run Shell File in PHP and open CLI (shell) to run shell scripts
</title>
</head>
</head>
<form action="shell.php" method ="post" align="center">
<input type="submit" value="Open .sh file using shell_exec() in PHP" name="openshellfile" />
<input type="submit" value="Run cmd/shell on Windows using shell_exec() in PHP" name="opencmd" />
</form>
</body>
</html>
<?php
// When user submits the form
if(isset($_POST['openshellfile'])){
echo $res=shell_exec('PATH to the file/demo.sh');
}
?>
Output:
Use shell_exec()
to Return Binary Format in CLI
The shell_exec()
function can be used for a variety of functions. Our method is an excellent way to use shell_exec()
without running a function in the background.
You do not need to use popen()
or pclose()
either.
<?php
// Ping facebook cmd (shell)
// open cmd shell
if (isset($_POST['opencmd']))
{
//You do not need to use popen() or pclose() either to run this shell command
// you can add any command here, it will work!
//For example, you can control your Windows (CLI)
//You will only change the command after cmd.ex /k "Your Command"
$open = shell_exec('start cmd.exe /k ping "facebook.com"');
echo $open;
//function shell($open) {
//check your php version
}
?>
Although we could have run any command using our script, we only pinged facebook.com
. For example, if you want to open a calculator through this function, type calc
instead of ping "facebook.com"
.
Before adding the above code in your PHP script, you first need to change the input field’s name in the HTML. Then add the above code in your shell.php
file that we previously ran.
The scope of the command can be anything, and you can type any windows command and assign it to the $open
variable. The script will run your command directly into the Windows shell (CLI in this example).
Output:
We executed a cmd command to ping facebook.com
using shell_exec();
in the code above. However, you can type any command in the function parameter, and it will work.
Sarwan Soomro is a freelance software engineer and an expert technical writer who loves writing and coding. He has 5 years of web development and 3 years of professional writing experience, and an MSs in computer science. In addition, he has numerous professional qualifications in the cloud, database, desktop, and online technologies. And has developed multi-technology programming guides for beginners and published many tech articles.
LinkedIn