How to Achieve Multithreading in PHP
-
Use the
Parallel
Parallel Concurrency Extension to Achieve Multithreading in PHP -
Use the
popen()
Function to Achieve Multithreading in PHP
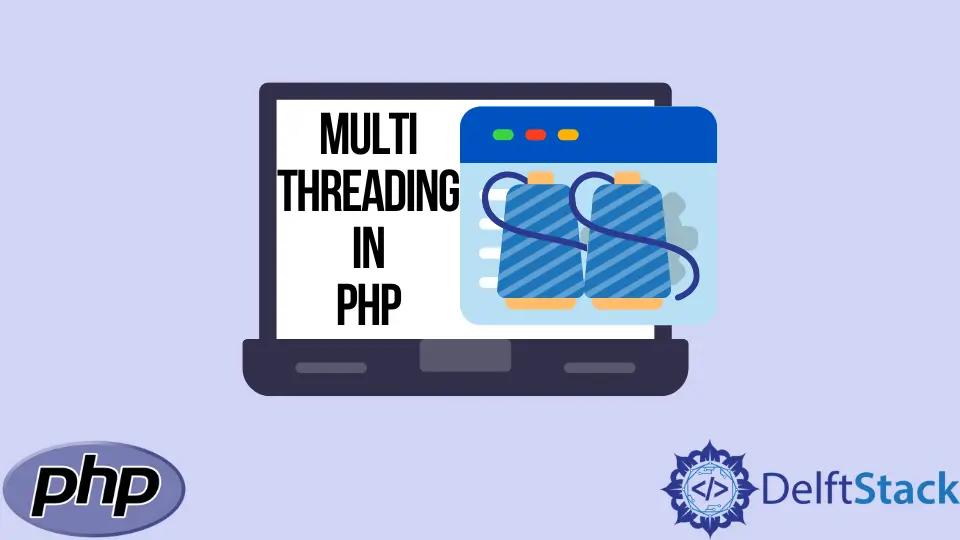
Multithreading is a form of program execution, where a single process creates several threads, and they execute simultaneously. This tutorial will discuss multithreading in PHP and demonstrate how to achieve it.
Use the Parallel
Parallel Concurrency Extension to Achieve Multithreading in PHP
Using the Parallel
parallel concurrency extension, we can achieve multithreading in PHP.
The extension provides a interpreter thread parallel\Runtime
. We can create an object from the parallel\Runtime()
class and thus create a thread.
The class provides a method run()
that schedules the tasks to run parallelly. We can pass Closure
as the parameter to the run
method.
The parameter is generally called task
, and we can also specify an array as the method’s second parameter. The content of the array is passed to the task.
There are some requirements before downloading the Parallel
parallel concurrency extension. The PHP version should be 8.0, and Zend Thread Safe (ZTS) should be enabled.
The <pthread.h>
header is another requirement. We can download the extension from pecl
as follows.
pecl install parallel
We can test the parallel execution of the program using the for
loop.
For example, we can run a loop inside the run()
method and another loop outside the method. In such conditions, the code execution will be parallel.
For example, create an object $rt
of the parallel\Runtime
class and then invoke the run()
method with the object. Inside the run()
method, write an anonymous function.
First, write a for
loop to print the +
sign fifty times inside the function. Next, outside the run()
method, write another for
loop to print the -
sign fifty times.
As the loop inside the run()
method runs in a separate thread, the loop outside the run()
method will execute concurrently. As a result, the -
and +
sign are printed simultaneously, as shown in the output section below.
Therefore, we can use the parallel concurrency extension to achieve multithreading in PHP.
Example Code:
$rt = new \parallel\Runtime();
$rt->run(function(){
for ($i = 0; $i < 50; $i++)
echo "+";
});
for ($i = 0; $i < 50; $i++) {
echo "-";
}
Output:
-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+
Use the popen()
Function to Achieve Multithreading in PHP
We can use the popen()
function to open parallel processes in PHP.
The function forks the process, and as a result, parallel processing is achieved. There is no sharing of the resources by the processes.
In this way, we can achieve multithreading in PHP. The popen()
function creates a pipe to the forked process.
We can loop over the popen()
function and create several processes to achieve multithreading. The popen()
function takes command
as the first parameter and mode
as the second parameter.
The mode can be either r
or w
.
For example, create a for
loop that loops five times. Inside the loop, create another for
loop that loops over five times.
Inside the child loop, create an array $process
that stores the popen()
function. Set the PHP file message.php
and the r
mode as the first and second parameters.
Next, create another child loop and use the pclose()
function to close the $process
.
Here, the five processes execute parallelly in the first child loop. The processes are terminated in the second child loop with the pclose()
function.
This is how we can achieve multithreading using the popen()
function in PHP.
Example Code:
for ($i=0; $i<5; $i++) {
for ($j=0; $j<5; $j++) {
$process[$j] = popen('message.php', 'r');
}
for ($j=0; $j<5; ++$j) {
pclose($process[$j]);
}
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook