How to Get the Current Full URL in PHP
-
Use
http_build_url()
to Get Current Url in PHP -
Use
$_SERVER[]
to Get Current Url in PHP -
Use
$_SERVER['HTTPS']
to Get Current Url in PHP - Get the Current URL Including Parameters in PHP
- Conclusion
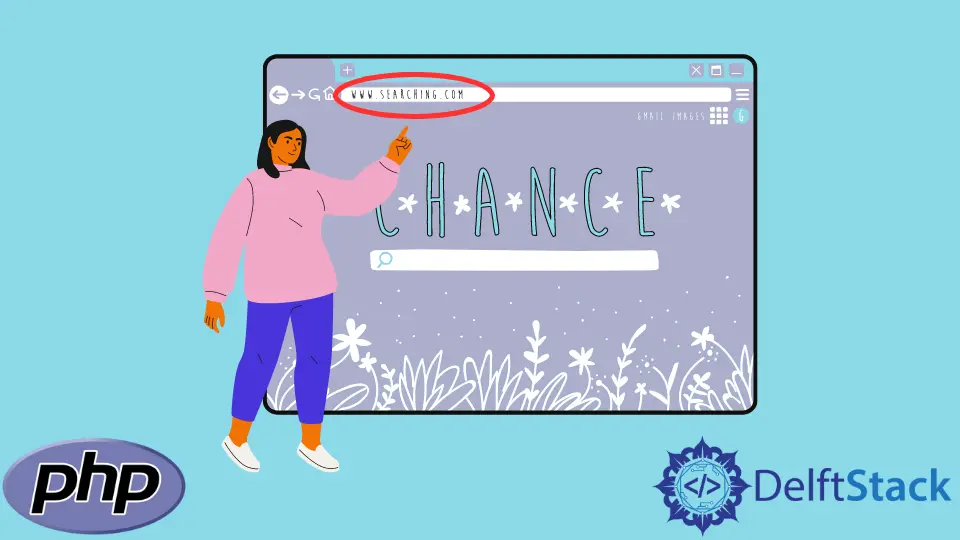
The tutorial introduces how to get the current full URL in the PHP programming language. There can be many use cases for getting the full URL of PHP. We have to render out the contents of the webpage based on query params. We may need to redirect or refresh the page as a whole. Hence, a full URL can be of essential value when it comes to web programming.
Here, we are going to discuss different ways to get the full URL in PHP programming.
Generally, there are few steps that we must know to get the complete URL of the currently running page, which are given below:
- We need to create a PHP variable that will store the URL in string format.
- We need to check if servers have HTTPS enabled. If it has, then we need to append
HTTPS
to the URL string. If HTTPS is not enabled, we need to appendHTTP
to the URL string. - We also need to append the
://
symbol to the URL. - We need to append the HTTP_HOST name of the server. HTTP_HOST name is the host that we have requested, e.g.
www.google.com
,www.exampledomain.com
, etc. - We need to append the REQUEST_URI to the URL string. REQUEST_URI is the resource which we have requested, e.g.
/index.php
, etc.
Now, we discuss some of the most efficient methods to get the current full URL in the PHP programming eco-system:
Use http_build_url()
to Get Current Url in PHP
This solution is pretty simple. We can use the PHP built-in function named http_build_url()
. This method works for both HTTP and HTTPs.
$link = http_build_url();
This function is used to build a URL. It accepts all together four parameters:
url
: This parameter represents the URL in the form of a string or associative array. It is returns value similar to parse_url().parts
: This parameter is the same as the first argument.flags
: It is a bitmask of binary HTTP_URL constants.new_url
: If this parameter is set, it will be filled with the parts of the composed URL likeparse_url()
returns.
Overall, this method returns the new URL as a string on success else False
on failure.
Use $_SERVER[]
to Get Current Url in PHP
Another way is by using the global variable named $_SERVER[]
. The $_SERVER
is a built-in variable of PHP that is used to get the current page URL. It is also a superglobal variable. Hence, it is always available in all scope.
We simply pass the parameter REQUEST_URI
to the variable as shown in the example below:
Example:
$link = "http://$_SERVER[HTTP_HOST]$_SERVER[REQUEST_URI]";
$_SERVER[HTTP_HOST]
represent hostname or domain name like https://www.delftstack.com/$_SERVER[REQUEST_URI]
represent URI like/dashboard/phpinfo.php
Use $_SERVER['HTTPS']
to Get Current Url in PHP
Here is another explicit hard code format to get the current URL in PHP.
The Global variable $_SERVER['HTTPS']
represents the status of the HTTPS. Hence, we can use it inside isset()
function to check if HTTPS exists or not. This will help us know if HTTPS is enabled in a URL or not. Meanwhile, by checking the value of $_SERVER['HTTPS']
, we can decide if or not to append “https” to the URL.
Both examples show the detection of HTTPS. The example below is the long format:
$link = (isset($_SERVER['HTTPS']) && $_SERVER['HTTPS'] === 'on' ? "https" : "http") . "://$_SERVER[HTTP_HOST]$_SERVER[REQUEST_URI]";
Or you can use the short format as shown in the example below:
$link = $_SERVER['REQUEST_SCHEME'] . '://' . $_SERVER['HTTP_HOST'].$_SERVER['REQUEST_URI']
Get the Current URL Including Parameters in PHP
In order to get the overall URL including the path, parameters, and query strings, we can use http_build_url()
as shown in the example below:
$all_in_address_bar = http_build_url();
Conclusion
This tutorial demonstrated some of the most efficient ways to get the current URL in PHP programming. The examples will surely help you understand the format, mechanism, and syntaxes used. The most used technique to get the current URL is by using the super global variable $_SERVER
. Using this, you have full control over the syntax format. However, the technique is a bit complex compared to the other ones. Now, it is up to you to decide which method of getting the current URL suits the best based on your PHP programming scenario. There can be different use-cases and efficiency in using a particular one. The best option might be to try each one of them and go with the most flexible and efficient one for your programming scenario.