PHP Dynamic Table Generation
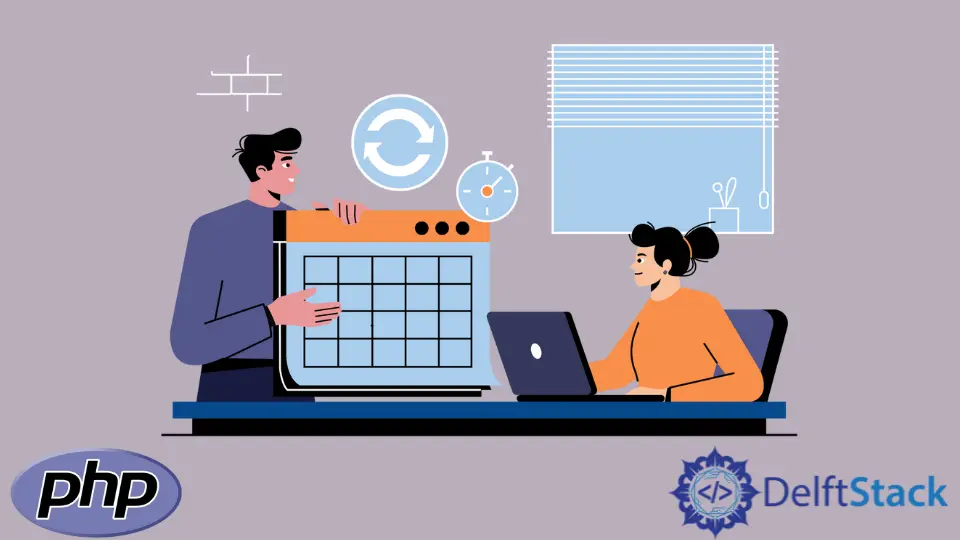
Normally, we use HTML for tables. However, we need our sites to dynamically extract fields from the database and incorporate the content in the HTML table dynamically.
This tutorial teaches two simple methods to generate a dynamic table in php instead.
Generate A Dynamic Table Using while
Loop in PHP
We use a while
loop and if
statement to generate these tables for the first example.
First, we set an incrementing variable $dynamictable
then start the while
loop. Each table row will contain 20 table cells <td>
and a total of 20 rows.
We also use line breaks and ending tags dynamically.
<!DOCTYPE html>
<body>
<div align="center">
<form action="table.php" method="post">
<input type="submit" value="Generate Dynamic Table" name="table">
<table border="2">
We use a form text field to use the isset()
function. When a user clicks on Generate Dynamic Table
using while
loop or for
loop.
The isset()
function is set to true
, and the code executes. It is unnecessary but will allow you to control your webpage more dynamically.
<?php
if(isset($_POST['table'])){
//initize incrementing varibale
$dynamictable = 0;
//start while loop
while ($dynamictable < 200)
{
// set modulus operator to divide table rows automatically
if($dynamictable%20 == 0)
{
//table row starts
echo "<tr>".PHP_EOL;
}
echo "<td>".$dynamictable."</td>".PHP_EOL;
//each time while loop increments table cell
$dynamictable++;
if($dynamictable%20 == 0)
{
//table row closes
echo "</tr>".PHP_EOL;
}
} // while loop closes
} // if isset condition closes
?>
Output:
Generate Dynamic Table Using for
Loop in PHP
Although there are multiple ways to generate a dynamic table with a for
loop, this simple code will be helpful to do most tasks.
We also use mt_rand
, assigning a unique number to each table cell.
<?php
//set table row and colums limit
$R = 10;
$C = 20;
echo "<table border=1>";
//start for loop and set rows limit
if(isset($_POST['forlooptable'])){
for($i=1;$i<=$R;$i++)
{
echo "<tr>";
//start for loop to set cols limit
for($j=1;$j<=$C;$j++){
//we are generating random numbers in each table cell
echo '<td>' . mt_rand($i, $i*100) . mt_rand($j, $j*100) . '</td>'; //mt_rand function randomly generates a number
}
echo "</tr>";
}
echo "</table>";
}
?>
</table>
</form>
</div>
</body>
</html>
Sarwan Soomro is a freelance software engineer and an expert technical writer who loves writing and coding. He has 5 years of web development and 3 years of professional writing experience, and an MSs in computer science. In addition, he has numerous professional qualifications in the cloud, database, desktop, and online technologies. And has developed multi-technology programming guides for beginners and published many tech articles.
LinkedIn