PHP cURL File Upload
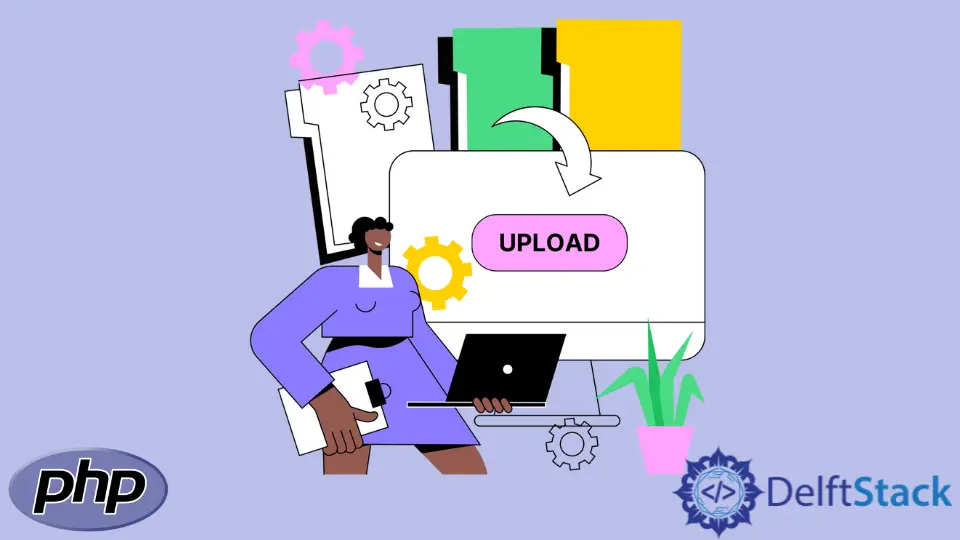
This tutorial will guide how to send an image file to the server with cURL and the CURLFile
class.
The idea is to post the image file from one page to another on a different page.
- The
Index.php
: First, we send the fileimage
to theindex.php
. Secondly, we redirect the file to thecurl.php
. - The
Curl.php
: After that, we move it to the upload folder usingcURLFile class
.
the cURLFile
Upload Method in PHP
For example, we are posting an image to the index.php
page, and then with the help of curl to the curl.php
page.
We do it by using the curl and CURLFile
class features. Let us see the function parameters in a working syntax.
//create curl file
if (function_exists('curl_file_create')) {
//determine file path
$tmp_file = curl_file_create($file_name_with_full_path);
} else { //
$tmp_file = '@' . realpath($file_name_with_full_path);
}
//store data in an array index
$data = array('extra_info' => '123456789','file_contents'=> $tmp_file);
$init = curl_init();
//function parameteres
curl_setopt($init, CURLOPT_URL,$url);
curl_setopt($init, CURLOPT_POST,1);
curl_setopt($init, CURLOPT_POSTFIELDS, $data);
$res=curl_exec ($init);
curl_close ($init);
The above example can depend on user-defined conditions since the URL can be to any server location.
However, we will now create a working example that you can use to move the files between the servers. It will allow you to clarify how to use the curl file class.
<!DOCTYPE html>
<html>
<body>
<form action="index.php" method="post" enctype="multipart/form-data" align="center">
<input type="file" name="image" />
<input type="submit" value= "Submit">
</form>
</body>
</html>
Normally, the HTML form collects the input file name and sent with the $_POST or $_REQUEST
variable in php.
However, with the help of curl file functionality, we can redirect it to a different server.
curl.php
for this tutorial instead of a different server since we are on the localhost, but it can be on any remote server.PHP cURL File Upload Working Example
index.php
:
<?php
if (isset($_FILES['image']['tmp_name'])){
//create a handler for curl function
$curl = curl_init(); //initialzie cURL session
//The CURLFile class
$cfile = new CURLFile($_FILES['image']['tmp_name'], $_FILES['image']['type'], $_FILES['image']['name']);
//use array to post data to different server or within localhost
$data = array ("myimage"=>$cfile);
//this url can be directed to any server location
//for the sake of this demo, we are using localhost directory to upload images in the upload folder
//Sets an option on the given cURL session handle
curl_setopt($curl, CURLOPT_URL, "http://localhost:7882/curl/curl.php");
curl_setopt($curl, CURLOPT_POST, true);
curl_setopt($curl, CURLOPT_POSTFIELDS, $data);
//assign execute curl to a response varible
$result = curl_exec($curl);
//check if the response varible is true
if($result == true){
echo "Your image has been uploaded successfully";
}
else {
// curl error returns a string comprising of error for the current session
echo "Error!" . curl_error($curl);
}
}
?>
Output:
The output.gif
image shows the file directory and how the file is moved from index.php
to the curl.php
. Then moved to the upload folder.
Curl.php
code:
<?php
//determine the array index
if(isset($_FILES['myimage']['tmp_name'])){
//set the path in the path variable
$path = "uploads/" . $_FILES['myimage']['name'];
//move the file to the path
move_uploaded_file($_FILES['myimage']['tmp_name'], $path);
?>
Conclusion
We have shown both the files and the output of how we executed the CURLFile
class from one server to another.
Users can use this method in various situations that require file uploading on different server locations.
Sarwan Soomro is a freelance software engineer and an expert technical writer who loves writing and coding. He has 5 years of web development and 3 years of professional writing experience, and an MSs in computer science. In addition, he has numerous professional qualifications in the cloud, database, desktop, and online technologies. And has developed multi-technology programming guides for beginners and published many tech articles.
LinkedIn