How to Sum of Columns of a Matrix in NumPy
- Understanding NumPy Arrays
- Method 1: Using the NumPy sum() Function
- Method 2: Using the NumPy apply_along_axis() Function
- Method 3: Using List Comprehensions
- Conclusion
- FAQ
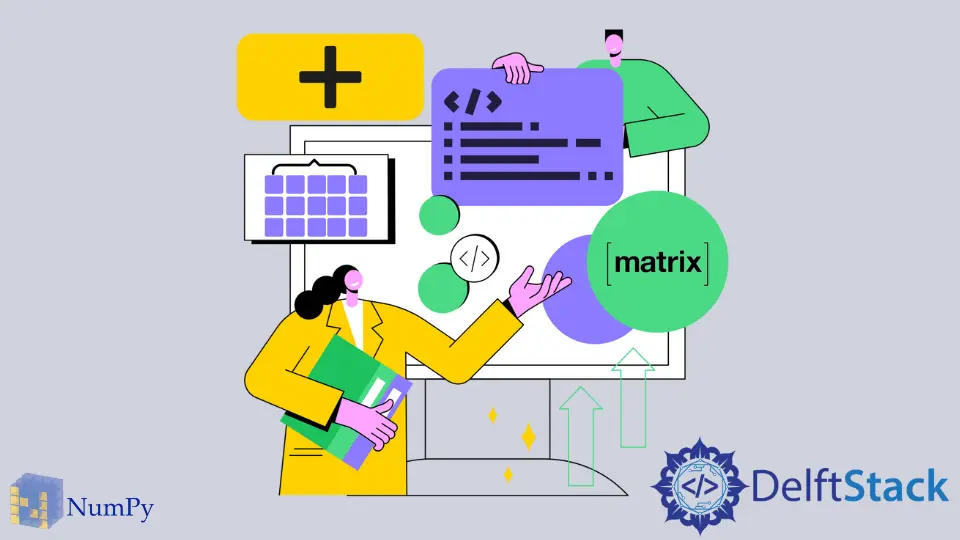
When working with numerical data in Python, NumPy stands out as a powerful library that simplifies many mathematical operations. One common task is calculating the sum of columns in a matrix. Whether you’re analyzing data, performing calculations, or simply manipulating arrays, knowing how to sum columns efficiently can save you time and effort.
In this tutorial, we’ll delve into the various methods to achieve this using NumPy, providing clear examples and explanations to ensure you grasp the concepts. By the end, you’ll be equipped with the knowledge to handle matrix operations with confidence.
Understanding NumPy Arrays
Before we dive into summing columns, it’s essential to understand what NumPy arrays are. NumPy is a library that provides support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays. An array can be one-dimensional (like a list) or multi-dimensional (like a matrix).
To sum the columns of a matrix, we first need to create a NumPy array. Here’s how you can do that:
import numpy as np
matrix = np.array([[1, 2, 3],
[4, 5, 6],
[7, 8, 9]])
In this example, we create a 3x3 matrix. Now that we have our matrix, let’s explore different methods to sum its columns.
Method 1: Using the NumPy sum() Function
One of the simplest ways to sum the columns of a matrix in NumPy is by using the sum()
function. This function can take an axis parameter, which allows you to specify whether you want to sum across rows or columns. By setting axis=0
, you can sum the columns.
column_sum = np.sum(matrix, axis=0)
Output:
[12 15 18]
When we call np.sum(matrix, axis=0)
, NumPy calculates the sum of each column. The result is an array where each element corresponds to the sum of the respective column in the original matrix. In our example, the first column sums to 12 (1+4+7), the second column to 15 (2+5+8), and the third column to 18 (3+6+9). This method is efficient and straightforward, making it a go-to choice for many users.
Method 2: Using the NumPy apply_along_axis() Function
Another way to sum the columns of a matrix is by using the apply_along_axis()
function. This function applies a specified function along a given axis of the array. In this case, we can use the sum
function.
column_sum_apply = np.apply_along_axis(np.sum, axis=0, arr=matrix)
Output:
[12 15 18]
Here, np.apply_along_axis(np.sum, axis=0, arr=matrix)
effectively achieves the same result as the previous method. It applies the sum function along the specified axis (in this case, columns). This method offers flexibility, as you can replace np.sum
with any other function you want to apply, making it a versatile tool for various operations.
Method 3: Using List Comprehensions
If you prefer a more manual approach, you can use Python’s list comprehensions to sum the columns. While this method is less efficient than the built-in NumPy functions, it can be useful for understanding how the summation works.
column_sum_list = [sum(matrix[:, i]) for i in range(matrix.shape[1])]
Output:
[12, 15, 18]
In this example, we use a list comprehension that iterates through each column index (from 0 to the number of columns). The expression matrix[:, i]
selects all rows for the i-th column, and sum()
calculates the total for that column. The result is a list containing the sums of each column. While this method demonstrates the underlying logic, it’s generally advisable to use NumPy’s built-in functions for performance and simplicity.
Conclusion
Summing the columns of a matrix in NumPy is a fundamental operation that can be accomplished through various methods. Whether you choose to use the straightforward sum()
function, the flexible apply_along_axis()
method, or the manual list comprehension approach, each has its advantages. As you continue to work with NumPy, mastering these techniques will enhance your ability to manipulate and analyze data efficiently. Remember, the choice of method often depends on the specific context of your task, so feel free to experiment with each.
FAQ
-
How do I install NumPy?
You can install NumPy using pip with the command pip install numpy. -
Can I sum rows instead of columns?
Yes, you can sum rows by setting the axis parameter to 1 in the sum() function. -
What is the output type of the sum() function?
The output of the sum() function is a NumPy array containing the sums of the specified axis. -
Are there performance differences between methods?
Yes, using built-in NumPy functions is generally faster and more efficient than manual methods like list comprehensions.
- Can I sum columns in a multi-dimensional array?
Yes, you can sum columns in higher-dimensional arrays by specifying the appropriate axis.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn