How to Save NumPy Array as Image in Python
-
Use the
Image.fromarray()
Function to Save a NumPy Array as an Image -
Use the
imageio.imwrite()
Function to Save a NumPy Array as an Image -
Use the
matplotlib.pyplot.imsave()
Function to Save a NumPy Array as an Image -
Use the
cv2.imwrite()
Function to Save a NumPy Array as an Image -
Use the
io.imsave()
Function to Save a NumPy Array as an Image - Conclusion
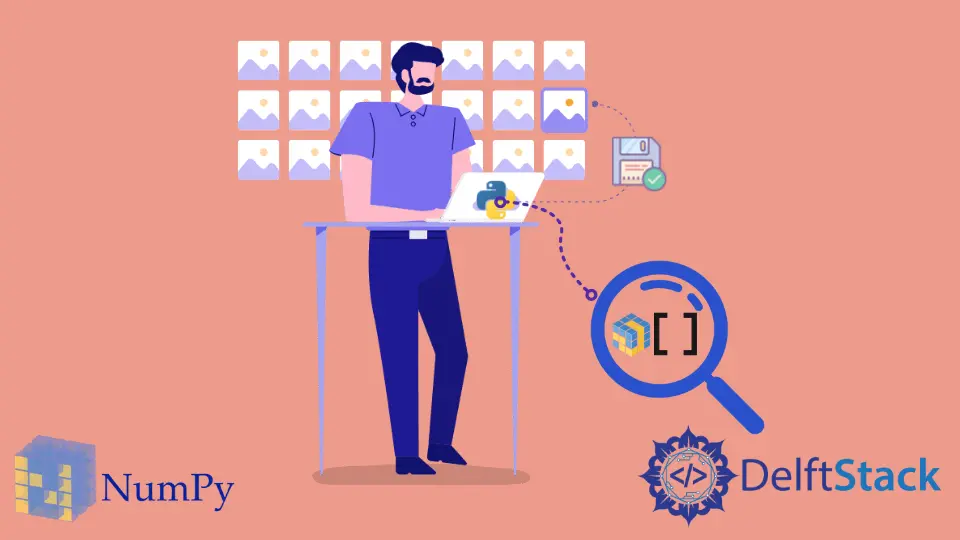
NumPy is a powerful library for numerical computing in Python, and it’s often used in various data science and image processing tasks. Sometimes, you may want to save a NumPy array as an image for further analysis, visualization, or sharing with others.
Fortunately, Python offers several methods to accomplish this task, and the choice depends on your specific requirements and the libraries you have available in your environment. In this article, we will explore different methods to save a NumPy array as an image in Python.
Use the Image.fromarray()
Function to Save a NumPy Array as an Image
The Image.fromarray()
function is part of the Pillow
library (PIL) in Python, and it is designed to create a Pillow Image
object from a NumPy array. This is especially useful when working with image data in numerical form, which is common in scientific and machine-learning applications.
Function Signature:
Image.fromarray(obj, mode=None)
-
obj
: This is the NumPy array or any other array-like object that you want to convert into aPillow Image
. -
mode
: This parameter specifies the color mode of the image. It should be a string that defines the color mode, such asL
for grayscale,RGB
for RGB,RGBA
for RGB with transparency, and others.If not provided,
Pillow
will try to infer the mode from the input data. However, it’s a good practice to specify the mode explicitly to avoid any ambiguity.
Now, before you start, ensure that you have NumPy and Pillow
installed. If not, you can install them using pip
:
pip install numpy pillow
Now, you can import the required libraries:
from PIL import Image
import numpy as np
The first step is to create a NumPy array that you want to save as an image. You can generate this array or load it from your data source.
For the sake of this example, let’s create a simple grayscale image array:
image_data = np.array([
[0, 128, 255],
[64, 192, 32],
[100, 50, 150]
], dtype=np.uint8)
In this example, we create a 3x3 NumPy array called image_data
, which represents a grayscale image. This array is defined with pixel intensity values ranging from 0
to 255
, and the data type is explicitly set to np.uint8
to ensure it’s interpreted as an 8-bit unsigned integer.
The Image.fromarray()
function allows you to convert the NumPy array to a Pillow Image
object. You can specify the image mode and provide the NumPy array:
image = Image.fromarray(image_data, mode="L")
The mode
parameter should match the color format of your image data.
Now that you have the image in the Pillow Image
format, you can easily save it in various image formats, such as PNG
, JPEG
, or BMP
:
image.save("output.png")
You can replace "output.png"
with your desired file name and format.
Here’s the complete example of saving a NumPy array as a grayscale image using Image.fromarray()
:
from PIL import Image
import numpy as np
image_data = np.array([
[0, 128, 255],
[64, 192, 32],
[100, 50, 150]
], dtype=np.uint8)
image = Image.fromarray(image_data, mode="L")
image.save("output.png")
This code snippet creates a simple grayscale image represented by a NumPy array and saves it as output.png
.
Let’s have another example of saving a NumPy array as an RGB image using Image.fromarray()
:
import numpy as np
from PIL import Image
image_data = np.array([
[(255, 0, 0), (0, 255, 0), (0, 0, 255)],
[(0, 0, 0), (128, 128, 128), (255, 255, 255)]
], dtype=np.uint8)
image = Image.fromarray(image_data, mode="RGB")
image.save("output.png")
Here, the mode
parameter is set to "RGB"
to specify that the NumPy array represents an RGB color image.
Use the imageio.imwrite()
Function to Save a NumPy Array as an Image
When you need to save a NumPy array as an image in Python, imageio.imwrite()
is also a straightforward and effective function to accomplish this task.
The imageio.imwrite()
function is used to write image data to a file in various formats. It is part of the imageio
library, which is a popular Python library for reading and writing images in multiple formats.
Function Signature:
imageio.imwrite(uri, im, format=None, **kwargs)
uri
: This is the file path or URI where the image file will be saved. It should include the file extension to specify the desired image format (e.g.,output.png
).im
: The image data that you want to save. This can be a NumPy array or any other image data format supported byimageio
.format
: The format parameter allows you to explicitly specify the image format if you don’t want to rely on the file extension. For example, you can usePNG
orJPEG
.**kwargs
: This parameter allows you to pass additional keyword arguments specific to the image format you are saving. Different image formats may have different options, and**kwargs
allows you to customize the output accordingly.
Before you get started, make sure you have NumPy and imageio
installed. If not, you can install them using pip
:
pip install numpy imageio
Now, you can import the required libraries:
import imageio
import numpy as np
First, create a NumPy array representing the image data you want to save. You can generate this array or load it from your data source.
For the sake of this example, we’ll create a simple grayscale image array:
image_data = np.array([
[0, 128, 255],
[64, 192, 32],
[100, 50, 150]
], dtype=np.uint8)
Here, image_data
is a 3x3 NumPy array representing a grayscale image with pixel intensity values ranging from 0
to 255
.
Then, use the imageio.imwrite()
function to save the NumPy array as an image. You can specify the file name, the NumPy array, and the desired format (e.g., png
, jpg
, or bmp
).
imageio.imwrite("output.png", image_data)
You can replace "output.png"
with your preferred file name and format.
Here’s the complete example to save a NumPy array as a grayscale image using imageio
:
import imageio
import numpy as np
image_data = np.array([
[0, 128, 255],
[64, 192, 32],
[100, 50, 150]
], dtype=np.uint8)
imageio.imwrite("output.png", image_data)
This code snippet generates a grayscale image represented by a NumPy array and saves it as output.png
.
You can also explicitly specify the image format using the format
parameter:
imageio.imwrite("output.jpg", image_data, format="JPEG")
In this case, we’ve specified "JPEG"
as the image format, even though the file extension is .jpg
.
Use the matplotlib.pyplot.imsave()
Function to Save a NumPy Array as an Image
The matplotlib.pyplot.imsave()
function provides a convenient way to save a NumPy array as an image in various formats. It is part of the Matplotlib library, which is primarily used for data visualization but can also handle image-related tasks.
Function Signature:
matplotlib.pyplot.imsave(fname, arr, **kwargs)
fname
: This parameter specifies the file name or path where the image file will be saved. You should include the file extension to specify the desired image format (e.g.,output.png
).arr
: The image data you want to save. This can be a NumPy array or any other image data format.**kwargs
: The**kwargs
parameter allows you to pass additional keyword arguments that control various aspects of the image-saving process. These arguments can be specific to the image format you are working with.
Before you get started, ensure you have Matplotlib installed. If you don’t have it, you can install it using pip
:
pip install matplotlib
Now, you can import the required libraries:
import matplotlib.pyplot as plt
import numpy as np
First, create a NumPy array that represents the image data you want to save. You can generate this array or load it from your data source.
For this example, we’ll create a simple grayscale image array:
image_data = np.array([
[0, 128, 255],
[64, 192, 32],
[100, 50, 150]
], dtype=np.uint8)
The image_data
array is a 3x3 NumPy array representing a grayscale image.
The matplotlib.pyplot.imsave()
function is easy to use and allows you to save a NumPy array as an image in various formats. You can specify the file name, the NumPy array, and the desired format.
plt.imsave("output.png", image_data, cmap='gray')
Replace "output.png"
with your desired file name and format.
Here’s a complete example of saving a NumPy array as a grayscale image using matplotlib.pyplot.imsave()
:
import matplotlib.pyplot as plt
import numpy as np
image_data = np.array([
[0, 128, 255],
[64, 192, 32],
[100, 50, 150]
], dtype=np.uint8)
plt.imsave("output.png", image_data, cmap='gray')
This code generates a grayscale image represented by a NumPy array and saves it as output.png
.
Note that you can control the appearance of the image by specifying the colormap using the cmap
keyword argument:
plt.imsave("colored_output.png", image_data, cmap='viridis')
Here, we use the viridis
colormap for a different appearance.
Matplotlib provides numerous customization options for images, and these can be specified as keyword arguments using **kwargs
. For example, you can control the DPI (dots per inch) of the image using the dpi
keyword argument:
plt.imsave("custom_dpi_output.png", image_data, dpi=300)
In this case, we’ve set the DPI to 300
.
Additionally, Matplotlib can handle various image formats, and by default, it tries to infer the format from the file extension. However, you can explicitly specify the format using the format
keyword argument if needed.
It supports several common image formats, including PNG
, JPEG
, BMP
, and more.
Use the cv2.imwrite()
Function to Save a NumPy Array as an Image
OpenCV provides various functions for working with images, including saving NumPy arrays as image files using the cv2.imwrite()
function.
Function Signature:
cv2.imwrite(filename, img, params=None)
filename
: This parameter specifies the file name or path where the image file will be saved. You should include the file extension to specify the desired image format (e.g.,output.png
).img
: The image data you want to save. This can be a NumPy array, an OpenCV image object, or any other image data format supported by OpenCV.params
: Theparams
parameter allows you to pass additional parameters specific to the image format you are saving. These parameters can be used to control various aspects of the saved image, such as compression quality or metadata.
Before you get started, ensure you have OpenCV installed. If you don’t have it, you can install it using pip
:
pip install opencv-python
Now, you can import the required libraries:
import cv2
import numpy as np
First, create a NumPy array representing the image data you want to save. You can generate this array or load it from your data source.
For this example, we’ll create a simple grayscale image array:
image_data = np.array([
[0, 128, 255],
[64, 192, 32],
[100, 50, 150]
], dtype=np.uint8)
Here, image_data
is a 3x3 NumPy array representing a grayscale image.
The cv2.imwrite()
function in OpenCV is simple to use and allows you to save a NumPy array as an image in various formats. You need to specify the file name and the NumPy array you want to save:
cv2.imwrite("output.png", image_data)
Replace "output.png"
with your desired file name and format.
Here’s a complete example of saving a NumPy array as a grayscale image using cv2.imwrite()
:
import cv2
import numpy as np
image_data = np.array([
[0, 128, 255],
[64, 192, 32],
[100, 50, 150]
], dtype=np.uint8)
cv2.imwrite("output.png", image_data)
This code generates a grayscale image represented by a NumPy array and saves it as output.png
.
You can also explicitly specify the other image formats using the file extension in the filename. For example, if you want to save the image in JPEG format:
cv2.imwrite("output.jpg", image_data)
In this case, we include the .jpg
file extension in the filename.
Use the io.imsave()
Function to Save a NumPy Array as an Image
The io.imsave()
function is part of the SciPy library and is used for saving image data in various formats. It’s particularly useful when you want to save NumPy arrays as images, which is a common task in scientific and computational applications.
Function Signature:
scipy.io.imsave(name, arr, plugin=None, **plugin_args)
name
: This parameter specifies the file name (including the path) where the image will be saved. You should include the file extension to indicate the desired image format (e.g.,output.png
).arr
: The image data to be saved. This can be a NumPy array, a NumPy structured array, or any other image-like array.plugin
: Theplugin
parameter allows you to specify the format in which the image should be saved. If not provided, SciPy will try to infer the format from the file extension.**plugin_args
: Additional keyword arguments that are specific to the chosen plugin. These parameters allow customization based on the image format you are working with.
Before you begin, make sure you have SciPy installed. If it’s not already installed, you can do so with pip
:
pip install scipy
Now, you can import the required libraries:
from scipy import io
import numpy as np
Start by creating a NumPy array that represents the image data you want to save. You can generate this array or load it from your data source.
For this example, we’ll create a simple grayscale image array:
image_data = np.array([
[0, 128, 255],
[64, 192, 32],
[100, 50, 150]
], dtype=np.uint8)
Here, image_data
is a 3x3 NumPy array representing a grayscale image.
Using the io.imsave()
function allows you to save the NumPy array as an image. You need to specify the file name, the NumPy array, and the desired format (e.g., png
, jpg
, or bmp
):
io.imsave("output.png", image_data)
You can replace "output.png"
with your desired file name and format.
Here’s a complete example of saving a NumPy array as a grayscale image using io.imsave()
:
from scipy import io
import numpy as np
image_data = np.array([
[0, 128, 255],
[64, 192, 32],
[100, 50, 150]
], dtype=np.uint8)
io.imsave("output.png", image_data)
This code generates a grayscale image represented by a NumPy array and saves it as output.png
.
Conclusion
In conclusion, the method you choose for saving a NumPy array as an image in Python depends on your specific requirements and the libraries available in your environment. Whether you need basic image saving or advanced image processing, Python offers a wide range of options to suit your needs.
Explore these methods and select the one that best fits your image-saving task.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn