How to Compare Two Arrays in Python
-
Compare Two Arrays in Python Using the
numpy.array_equal()
Method -
Compare Two Arrays in Python Using the
numpy.allclose()
Method -
Compare Two Arrays in Python Using the
numpy.array_equiv()
Method -
Compare Two Arrays in Python Using the
==
Operator andnumpy.all()
Method
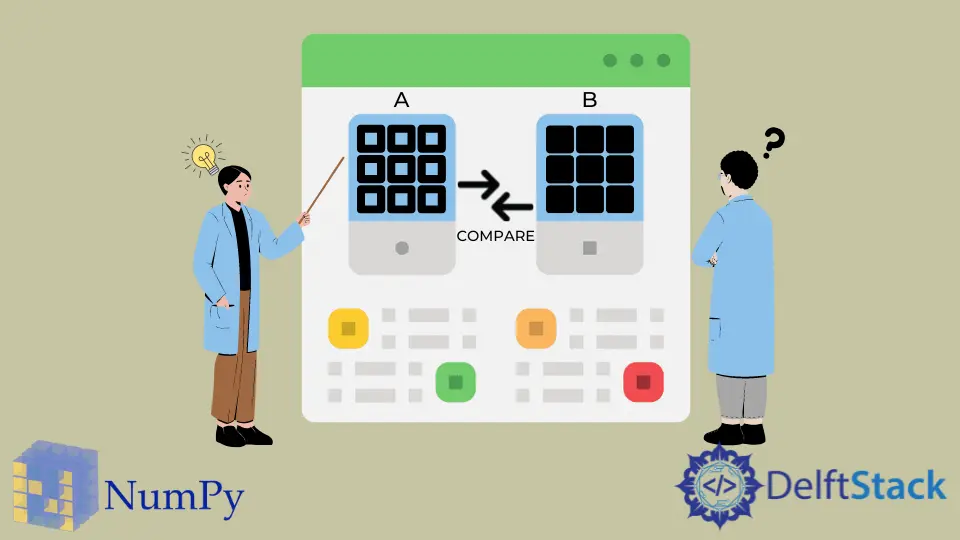
In this tutorial, we will look into various methods to compare two arrays in Python and check if they are equal or not. The two arrays will only be equal when their dimensions and values are the same. If the two arrays have the same values, but their sequence is not the same, then the arrays will not be considered equal.
We can check if the two arrays are equal or not in Python using the following methods:
Compare Two Arrays in Python Using the numpy.array_equal()
Method
The numpy.array_equal(a1, a2, equal_nan=False)
takes two arrays a1
and a2
as input and returns True
if both arrays have the same shape and elements, and the method returns False
otherwise. The default value of the equal_nan=
keyword argument is False
and must be set True
if we want the method to consider two NaN
values as equal.
The below example code demonstrates how to use the numpy.array_equal()
method to compare two arrays in Python.
import numpy as np
a1 = np.array([1, 2, 4, 6, 7])
a2 = np.array([1, 3, 4, 5, 7])
print(np.array_equal(a1, a1))
print(np.array_equal(a1, a2))
Output:
True
False
Compare Two Arrays in Python Using the numpy.allclose()
Method
The numpy.allclose(a1, a2, rtol=1e-05, atol=1e-08, equal_nan=False)
method takes array a1
and a2
as input and returns True
if the each element of a1
is equal to corresponding element of a2
, or their difference is within the tolerance value.
The value of tolerance is calculated using the a2
, rtol
, and atol
arguments.
atol + rtol * absolute(a2)
The numpy.allclose()
method is useful in calculations where we want to check whether the final arrays are equal to the expected array or not. We can use the numpy.allclose()
method to compare two arrays in Python in the following way:
import numpy as np
a1 = np.array([1, 2, 4, 6, 7])
a2 = np.array([1, 3, 4, 5, 7])
a3 = np.array([1, 3, 4.00001, 5, 7])
print(np.allclose(a1, a2))
print(np.allclose(a3, a2))
Output:
False
True
As shown in the above example code, the difference of 0.00001
between the two values is ignored by default. We can change the values of atol
and rtol
to increase tolerance value.
Compare Two Arrays in Python Using the numpy.array_equiv()
Method
The numpy.array_equiv(a1, a2)
method takes array a1
and a2
as input and returns True
if both arrays’ shape and elements are the same; otherwise, returns False
.
We can pass both arrays to the numpy.array_equiv()
method to compare them in Python. The below example code demonstrates how to use the numpy.array_equal()
method to check if the two arrays are equal in Python.
import numpy as np
a1 = np.array([1, 2, 4, 6, 7])
a2 = np.array([1, 3, 4, 5, 7])
a3 = np.array([1, 3, 4.00001, 5, 7])
print(np.array_equiv(a1, a2))
print(np.array_equiv(a3, a2))
Output:
False
False
Compare Two Arrays in Python Using the ==
Operator and numpy.all()
Method
The ==
operator when used with the arrays, returns the array with the shape equivalent to both arrays, the returned array contains True
at an index if the elements of both arrays are equal in that index, and the array will otherwise contain False
at that index.
As we want to compare the two arrays instead of each element, we can use the numpy.all()
method with the ==
operator. The numpy.all()
method returns True
if all the input array elements along the given axis are True
; otherwise, it returns False
.
True
if both arrays are empty or one array has a length of 1
. And also will raise an error if the shape of both arrays is not the same; that is why the methods mentioned above must be preferred.The below example code demonstrates how to use the ==
operator and numpy.all()
method to compare the two arrays in Python.
import numpy as np
a1 = np.array([1, 2, 4, 6, 7])
a2 = np.array([1, 3, 4, 5, 7])
a3 = np.array([1, 3, 4.00001, 5, 7])
print((a1 == a2).all())
print((a3 == a2).all())
Output:
False
False