How to Check NumPy Version in Python
-
Use the
__version__
Function to Find the Version of NumPy Module -
Use the
importlib.metadata
Module to Find the Version of the NumPy Module -
Use the
pkg_resources
Module to Find the Version of NumPy Module -
Use of
pip
Commands to Find the Version of the NumPy Module
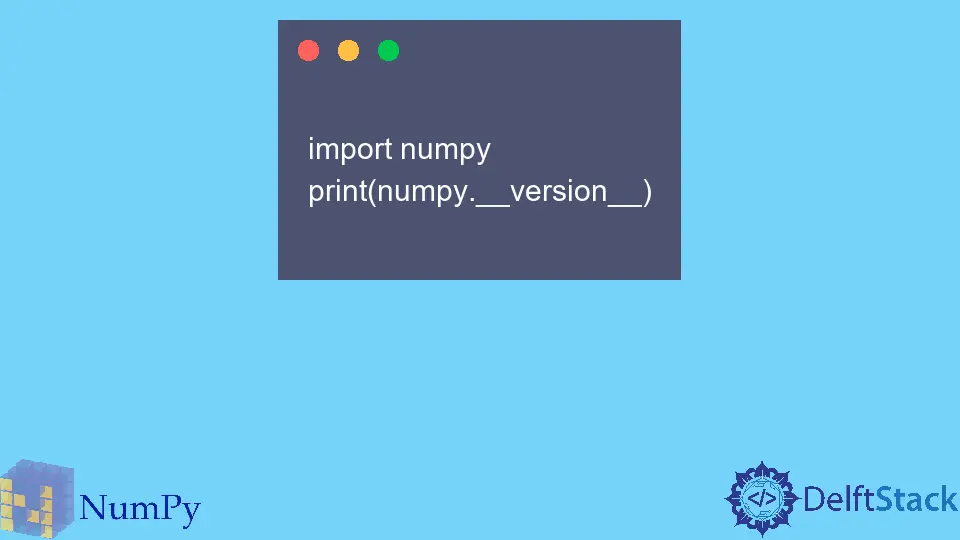
The numpy module is used to work with arrays in Python. It has functions and classes which can also be used to perform mathematical and logical operations on these objects.
In this tutorial, we will discuss how to check the version of numpy module.
Use the __version__
Function to Find the Version of NumPy Module
Usually, most of the modules have the __version__
method associated with them, which can return its version. This method is available in the numpy module.
For example,
import numpy
print(numpy.__version__)
Output:
1.16.5
Alternatively, we can also use the version.version
method in a similar way.
import numpy
print(numpy.version.version)
Output:
1.16.5
Use the importlib.metadata
Module to Find the Version of the NumPy Module
In Python v3.8 and above, we have the importlib.metadata
module, which has the version()
function. This function will return the version of the specified module.
For example,
from importlib_metadata import version
print(version("numpy"))
Output:
1.16.5
We can also use the import_metadata
module for older versions of Python.
Use the pkg_resources
Module to Find the Version of NumPy Module
Below Python 3.8, we can use the get_distribution.version()
method from the pkg_resources
module to find the version of the numpy module. Note that the string that you pass to the get_distribution
method should correspond to the PyPI entry.
For example,
import pkg_resources
print(pkg_resources.get_distribution("numpy").version)
Output:
1.16.5
Use of pip
Commands to Find the Version of the NumPy Module
We can use many pip
commands to stay up to date. Alternatively, we can use the pip show
command to find out details about a specific package that includes its version. For the numpy module, we will use the following command.
pip show numpy
Note that pip
should be updated for this.
Another pip
command which we can use is the pip list
. It shows all the installed packages along with their versions. We can check the version as below.
pip list
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn