How to Transpose a 1D Array in NumPy
- Understanding 1D Arrays in NumPy
- Reshaping a 1D Array to 2D
- Using the New Axis Method
- Conclusion
- FAQ
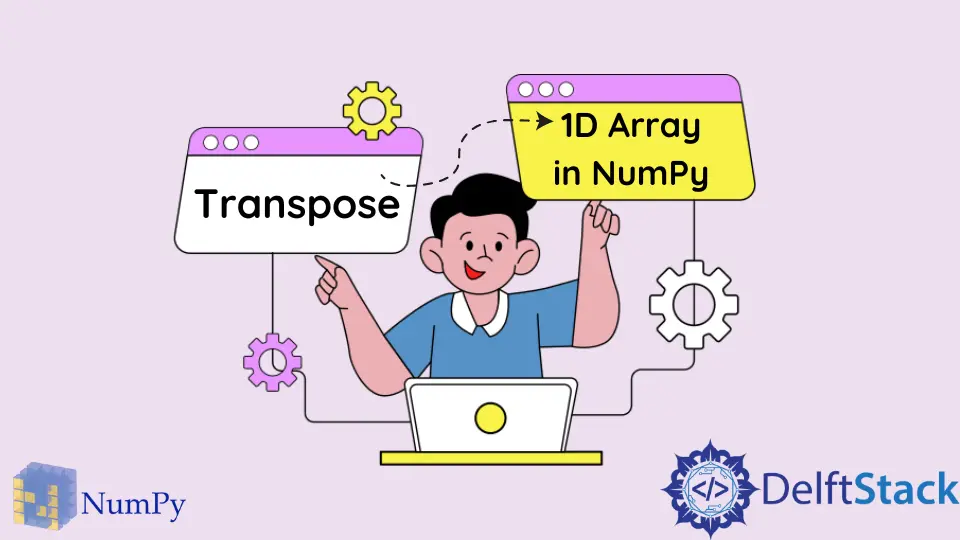
Transposing a 1D array in NumPy can be a bit of a misnomer since a true transpose operation typically applies to 2D arrays. However, it’s essential to understand how to manipulate arrays effectively in Python, especially when working with data structures.
In this article, we will explore how to handle 1D arrays in NumPy, focusing on reshaping and altering their dimensions. If you’re looking to dive into array manipulation, you’ve come to the right place. We’ll cover practical methods to reshape your data, making it easier to perform mathematical operations or prepare it for machine learning tasks. Let’s get started!
Understanding 1D Arrays in NumPy
Before we dive into transposing, it’s crucial to grasp what a 1D array is. In NumPy, a 1D array is essentially a linear collection of elements. Think of it like a list of numbers. You can create a 1D array using the numpy.array()
function. Here’s how you can do that:
import numpy as np
array_1d = np.array([1, 2, 3, 4, 5])
print(array_1d)
Output:
[1 2 3 4 5]
This simple command creates a 1D array containing five elements. While you can’t technically transpose a 1D array, you can reshape it into a 2D format, which is often what users are looking to achieve when they mention transposing.
Reshaping a 1D Array to 2D
The first method we will discuss is reshaping a 1D array into a 2D array. This is often the desired outcome when users think of transposing. You can use the reshape()
method to convert a 1D array into a 2D array. Here’s how it works:
array_2d = array_1d.reshape(1, -1) # Reshape to 1 row and n columns
print(array_2d)
Output:
[[1 2 3 4 5]]
In this example, we used reshape(1, -1)
, which means we want one row and as many columns as needed to accommodate the five elements in our original 1D array. The -1
acts as a placeholder, allowing NumPy to automatically calculate the necessary number of columns.
You can also reshape it into a column format:
array_2d_column = array_1d.reshape(-1, 1) # Reshape to n rows and 1 column
print(array_2d_column)
Output:
[[1]
[2]
[3]
[4]
[5]]
Here, we have transformed our 1D array into a 2D array with five rows and one column. This transformation is particularly useful when preparing data for operations that require 2D input, such as matrix multiplication or feeding data into machine learning models.
Using the New Axis Method
Another effective way to achieve a similar outcome is by using NumPy’s newaxis
function. This method allows you to add a new axis to your existing array, effectively reshaping it without needing to specify dimensions explicitly. Here’s how you can do it:
array_2d_newaxis = array_1d[np.newaxis, :]
print(array_2d_newaxis)
Output:
[[1 2 3 4 5]]
In this case, np.newaxis
is used to add a new axis to the array. The result is a 2D array with one row, similar to what we achieved using the reshape()
method.
You can also create a column vector using newaxis
:
array_2d_column_newaxis = array_1d[:, np.newaxis]
print(array_2d_column_newaxis)
Output:
[[1]
[2]
[3]
[4]
[5]]
This method is particularly handy when you want to maintain the original data structure while altering its shape. It’s a clean and efficient way to manipulate your data, especially in data science and machine learning applications.
Conclusion
In this article, we explored various methods to manipulate a 1D NumPy array, focusing on reshaping it into a 2D format. While you can’t technically transpose a 1D array, you can achieve similar results by reshaping or adding new axes. Whether you’re preparing data for analysis or machine learning, understanding these techniques will significantly enhance your data manipulation skills in Python. Keep experimenting with NumPy, and you’ll uncover even more powerful features that can streamline your data processing tasks.
FAQ
-
What is a 1D array in NumPy?
A 1D array in NumPy is a linear collection of elements, similar to a list in Python. -
Can you transpose a 1D array?
No, you cannot transpose a 1D array. However, you can reshape it into a 2D array. -
How do I convert a 1D array to a 2D array?
You can use thereshape()
method ornp.newaxis
to convert a 1D array to a 2D array. -
What is the purpose of reshaping an array?
Reshaping an array allows you to manipulate its dimensions, which is often necessary for mathematical operations or machine learning tasks. -
Is there a performance difference between reshape and newaxis?
Generally, both methods are efficient, butnewaxis
may be more readable for adding a new dimension without specifying the shape explicitly.