How to Remove Elements From Array in NumPy
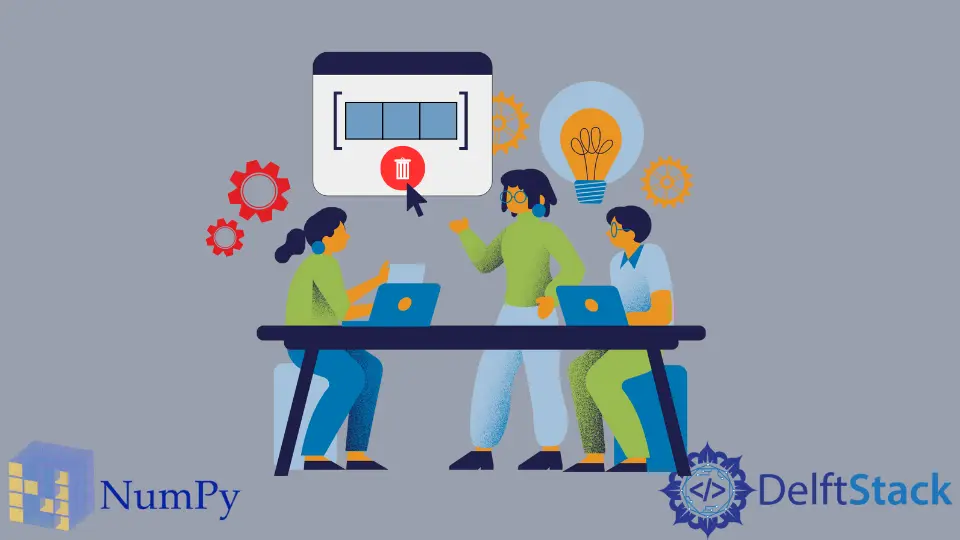
In this article, we will learn about two ways to remove elements from a NumPy array.
Remove Elements Using numpy.delete()
Function
Refer to the following code.
import numpy as np
myArray = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
indexes = [3, 5, 7]
modifiedArray = np.delete(myArray, indexes)
print(modifiedArray)
Output:
[ 1 2 3 5 7 9 10]
In the above code, we use the delete()
function of the NumPy
library. The delete()
function accepts three parameters, namely, arr
, obj
, and axis
and outputs a NumPy array. The arr
is the NumPy array we wish to delete elements from. obj
is a list of integer numbers. These numbers represent the indexes of the elements that should be deleted from the array. Lastly, the axis
is an optional argument. axis
refers to the axis along which the elements targetted by the obj
should be deleted. If a None
value is assigned to this parameter, arr
is flattened, and deletion is carried out on this flattened array.
As usual, if an index that lies outside the range of arr
is provided to this method, it throws an IndexError
exception.
import numpy as np
myArray = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
indexes = [3, 5, 7, 34]
modifiedArray = np.delete(myArray, indexes)
print(modifiedArray)
Output:
Traceback (most recent call last):
File "<string>", line 5, in <module>
File "<__array_function__ internals>", line 5, in delete
File "/path/to/library/numpy/lib/function_base.py", line 4480, in delete
keep[obj,] = False
IndexError: index 34 is out of bounds for axis 0 with size 10
To learn more about this function, refer to the official documentation of this function here
Here are two more examples for deletion in a multidimensional NumPy array.
import numpy as np
myArray = np.array([[1, 2, 3, 4, 5], [11, 12, 13, 14, 15], [21, 22, 23, 24, 25]])
modifiedArray = np.delete(myArray, [1, 2], 1)
print(modifiedArray)
Output:
[[ 1 4 5]
[11 14 15]
[21 24 25]]
Use None
as the value for parameter axis
.
import numpy as np
myArray = np.array([[1, 2, 3, 4, 5], [11, 12, 13, 14, 15], [21, 22, 23, 24, 25]])
modifiedArray = np.delete(myArray, [1, 2], None)
print(modifiedArray)
Output:
[ 1 4 5 11 12 13 14 15 21 22 23 24 25]
Remove Elements Using numpy.setdiff1d()
Function
This time we will use the setdiff1d()
function from NumPy
. This function accepts three parameters, ar1
, ar2
, and assume_unique
. ar1
and ar2
are two NumPy arrays. And assume_unique
is an optional boolean argument. Its default value is False
. When it’s True
, then the two input arrays are assumed to be unique, and this assumption can speed up the calculation time.
setdiff1d()
returns the unique values in ar1
that are not in ar2
.
Refer to the following code.
import numpy as np
myArray = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
indexes = [3, 5, 7]
modifiedArray = np.setdiff1d(myArray, indexes)
print(modifiedArray)
Output:
[ 1 2 4 6 8 9 10]
Unlike numpy.delete()
, both the arrays are NumPy arrays with actual elements in them but not indexes.
To learn more about this function, refer to the official documentation of this function here.