NumPy Mask 2d Array
- Create Mask or 2d Boolean Array With NumPy in Python
- Create Mask With Python Logical Operators
- Create Mask With NumPy Logical Function
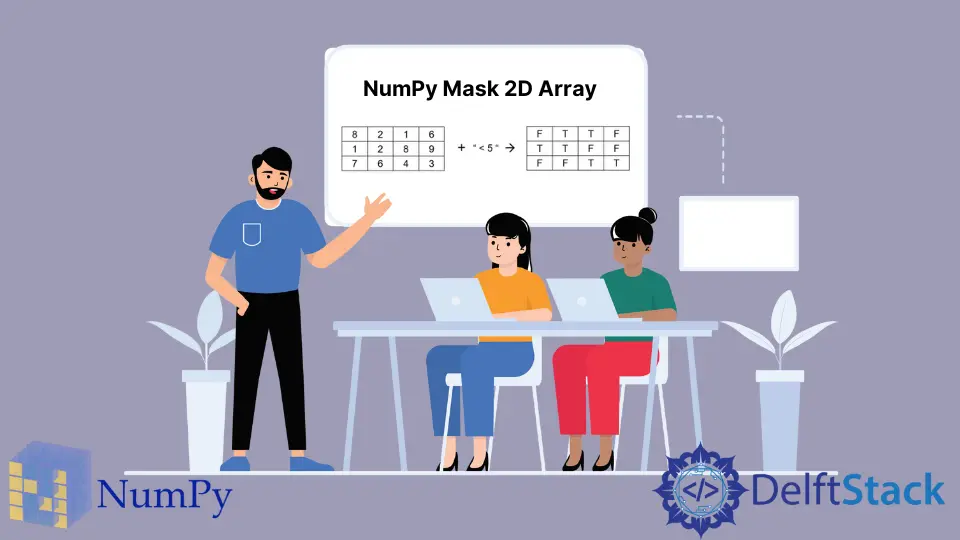
We go to learn with this explanation about what is the mask or Boolean array. We also learn how to create a 2d mask with logical P1ython operators and NumPy logical function in python.
Create Mask or 2d Boolean Array With NumPy in Python
We start with some array where we apply some condition, and then we generate a mask
or a Boolean array. For example, let’s think about just an array of integers shown below, and then we apply this condition, which is less than five.
The resulting Boolean array would be the same shape as the input array, and it would just be an element-by-element application of the condition. In this case, 8 is less than 5, so that is false, 2 is less than 5, that is true, 1 is less than 5 true, and so on.
Sometimes it represents mask by 0-1, and the false represents 0 and 1 represents true.
Create Mask With Python Logical Operators
We’ll jump into the code by importing numpy
and create a variable called My_2DArray
, which is populated with a Python 2d list using a numpy
array.
import numpy as np
My_2DArray = np.array([[-12, -31, 5], [7, 0, -9]])
print(My_2DArray)
Output:
[[-12 -31 5]
[ 7 0 -9]]
Let’s have an example to demonstrate mask
. We are creating a new variable called zero_mod_array
, which takes the values where My_2DArray
is divisible by 7 using the %
operator.
We select all elements in the array where the remainder after division by 7 equals zero.
Code:
import numpy as np
My_2DArray = np.array([[-12, -31, 5], [7, 0, -9]])
zero_mod_array = 0 == (My_2DArray % 7)
print(zero_mod_array)
After executing, we see that we have created an array of Boolean values. If we look at the first element of an array that is -12 is not divisible by 7, and the next one is also not, but the first element in the second list is 7, which is divisible by 7, and the second element 0 is also divisible by 7.
Therefore, both elements are True
except for other elements because all elements are not divisible by 7, which is why we got False
values on their positions.
Output:
[[False False False]
[ True True False]]
In this following example, we are creating a variable called Sun_array
, and these would be essential elements of Boolean mask arrays. We are taking My_2DArray
and indexing it with the results we created in zero_mod_array
.
Code:
import numpy as np
My_2DArray = np.array([[-12, -31, 5], [7, 0, -9]])
zero_mod_array = 0 == (My_2DArray % 7)
Sun_array = My_2DArray[zero_mod_array]
print(Sun_array)
Output:
[7 0]
Let’s use Sun_array
as a mask array to index itself, selecting the elements within Sun_array
that are greater than 0 and creating a new array that only contains the positive elements.
Sun_array[Sun_array > 0]
In our Sun_array
only one element is greater than 7 array([7])
. If we examine the Sun_array
, we will see that the values are unchanged; it is still an array([7, 0])
.
Create Mask With NumPy Logical Function
Let’s examine an alternative method to accomplish the same task. In a particular way, we will use NumPy logical operators.
First, we will create a variable called mod_test
, which will assign the results of the remainder operator as we did above.
We will do something similar and create another variable called positive_test
, and this time we will assign the values where My_2DArray
is greater than zero, which means it will indicate the Boolean values after applying the condition on every element of My_2DArray
.
We’ll create another variable called combined_test
and which uses the logical_and()
function and it takes mod_test
and positive_test
as arguments.
Code:
import numpy as np
My_2DArray = np.array([[-12, -31, 5], [7, 0, -9]])
mod_test = 0 == (My_2DArray % 7)
positive_test = My_2DArray > 0
combined_test = np.logical_and(mod_test, positive_test)
print(combined_test)
After execution, we see the perfect Boolean values, and it contains only one value that corresponds to the array.
Output:
[[False False False]
[ True False False]]
We can use combined_test
to index our original array and get the same value we achieved above.
Code:
import numpy as np
My_2DArray = np.array([[-12, -31, 5], [7, 0, -9]])
mod_test = 0 == (My_2DArray % 7)
positive_test = My_2DArray > 0
combined_test = np.logical_and(mod_test, positive_test)
print(My_2DArray[combined_test])
Output:
[7]
This is how we can mask the NumPy 2d array using two techniques to achieve the same result.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn