How to Convert Float Array to Int Array in NumPy
- Understanding NumPy Arrays
-
Method 1: Using the
astype()
Function - Method 2: Using the numpy.floor() Function
- Method 3: Using the numpy.ceil() Function
- Method 4: Using the numpy.round() Function
- Conclusion
- FAQ
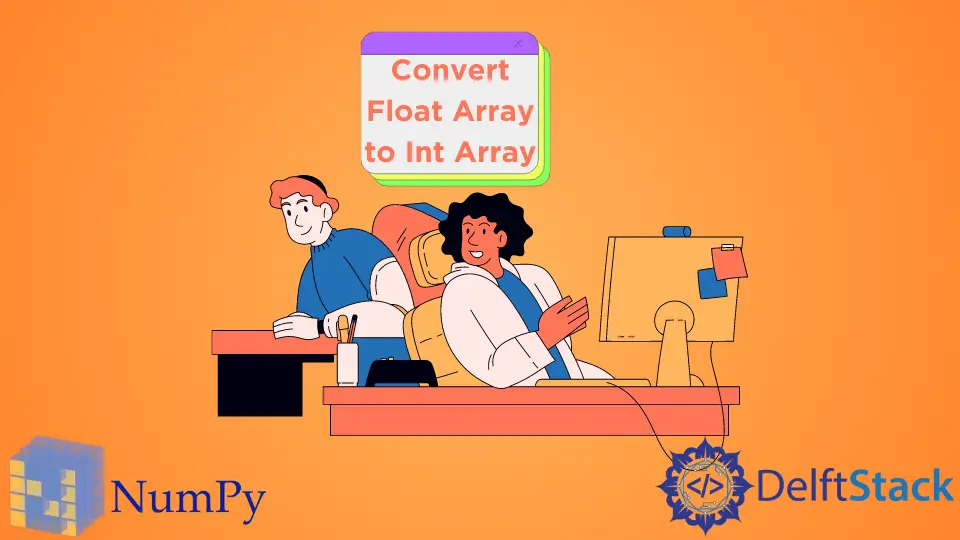
Converting a float array to an integer array in NumPy is a common task that many Python developers encounter. Whether you’re working on data analysis, machine learning, or scientific computing, understanding how to manipulate data types effectively can streamline your workflows.
In this article, we will explore the process of converting a 2D float NumPy array into a 2D integer NumPy array. We will discuss various methods, providing clear code examples and detailed explanations for each approach. By the end of this guide, you’ll be equipped with the knowledge to handle float-to-int conversions seamlessly, enhancing your data manipulation skills in Python.
Understanding NumPy Arrays
NumPy is a powerful library for numerical computing in Python, providing support for multi-dimensional arrays and a collection of mathematical functions to operate on these arrays. When working with data, you may find that your numerical values are stored as floats, but for certain applications, you might need them as integers. This conversion is not only straightforward but also essential for ensuring compatibility with functions that require integer inputs.
Method 1: Using the astype()
Function
One of the simplest ways to convert a float array to an integer array is by using the astype()
method. This method allows you to specify the desired data type for the conversion. Here’s how you can do it:
import numpy as np
float_array = np.array([[1.2, 2.5, 3.8], [4.1, 5.6, 6.7]])
int_array = float_array.astype(int)
print(int_array)
Output:
[[1 2 3]
[4 5 6]]
The astype(int)
function converts each float in the array to its corresponding integer value. It effectively truncates the decimal part, which is important to note. In the example above, 1.2
becomes 1
, 2.5
becomes 2
, and so forth. This method is highly efficient and is often the preferred way to handle data type conversions in NumPy due to its simplicity and speed.
Method 2: Using the numpy.floor() Function
Another approach to converting float arrays to integers involves using the numpy.floor()
function. This function rounds each element down to the nearest integer. Here’s how it works:
import numpy as np
float_array = np.array([[1.9, 2.1, 3.7], [4.8, 5.3, 6.9]])
int_array = np.floor(float_array).astype(int)
print(int_array)
Output:
[[1 2 3]
[4 5 6]]
In this example, the numpy.floor()
function is applied to the float array, which rounds each value down to the nearest whole number before converting it to an integer with astype(int)
. This method can be particularly useful when you want to ensure that you are rounding down, rather than simply truncating. It’s a great option when dealing with datasets where rounding behavior is critical.
Method 3: Using the numpy.ceil() Function
If your goal is to round up instead of down, you can use the numpy.ceil()
function. This function rounds each element to the smallest integer greater than or equal to the input value. Here’s an example:
import numpy as np
float_array = np.array([[1.2, 2.5, 3.8], [4.1, 5.6, 6.7]])
int_array = np.ceil(float_array).astype(int)
print(int_array)
Output:
[[2 3 4]
[5 6 7]]
In this code snippet, numpy.ceil()
rounds each float value up to the nearest integer. For instance, 1.2
becomes 2
, 2.5
becomes 3
, and so on. This method is particularly useful in scenarios where you want to ensure that your values are not underestimated, which can be crucial for certain calculations or algorithms.
Method 4: Using the numpy.round() Function
The numpy.round()
function offers yet another way to convert floats to integers by rounding to the nearest integer. This method can be particularly handy when you want a more balanced approach to rounding. Here’s how you can implement it:
import numpy as np
float_array = np.array([[1.4, 2.6, 3.2], [4.5, 5.1, 6.9]])
int_array = np.round(float_array).astype(int)
print(int_array)
Output:
[[1 3 3]
[4 5 7]]
In this example, numpy.round()
rounds each element to the nearest integer. If the decimal part is exactly 0.5, it rounds to the nearest even integer. This behavior is known as “bankers’ rounding.” For instance, 1.4
rounds down to 1
, while 2.6
rounds up to 3
. This method is particularly useful in statistical applications where rounding errors can accumulate.
Conclusion
Converting a float array to an integer array in NumPy is a fundamental task that can significantly impact your data processing and analysis workflows. Whether you choose to use astype()
, floor()
, ceil()
, or round()
, each method has its unique advantages depending on your specific needs. By mastering these techniques, you can enhance your data manipulation skills and ensure that your numerical data is in the correct format for your applications. With the right understanding of these methods, you’ll be well-equipped to handle any float-to-int conversion challenges that come your way.
FAQ
-
What is the difference between astype() and numpy.floor()?
astype() converts the data type without rounding, while numpy.floor() rounds down to the nearest integer before converting. -
Can I convert a 1D float array to an int array using the same methods?
Yes, all the methods discussed can be applied to 1D arrays as well. -
What happens to negative float values when using ceil() or floor()?
ceil() will round negative values up towards zero, while floor() will round them down away from zero. -
Is there a performance difference between these methods?
Generally, astype() is the fastest, but the performance can vary based on the size of the array and the method used. -
Can I convert a float array to other data types using astype()?
Yes, astype() can convert arrays to various data types, including float, int, and complex numbers.