How to Filter Elements in a NumPy Array
-
Filter Elements Using the
fromiter()
Method in NumPy - Filter Elements Using Boolean Mask Slicing Method in NumPy
-
Filter Elements Using the
where()
Method in NumPy
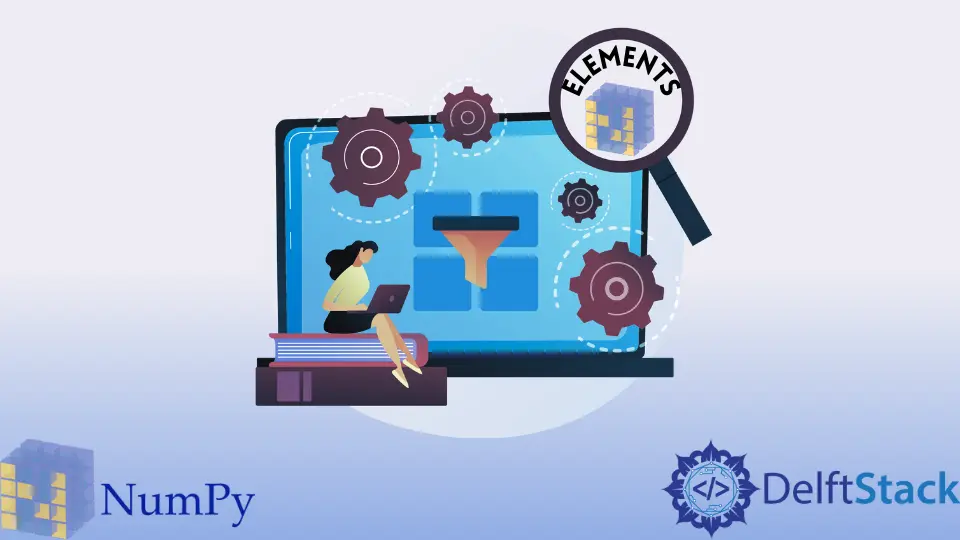
Often, we need values from an array in a specific, usually in either an ascending or descending order. Sometimes, we also have to search elements from an array and retrieve them or filter some values based on some conditions.
This article will introduce how to filter values from a NumPy array.
Filter Elements Using the fromiter()
Method in NumPy
fromiter()
creates a new one-dimensional array from an iterable object which is passed as an argument. We can apply conditions to the input array elements and further give that new array to this function to get the desired elements in a NumPy array.
The syntax of the fromiter()
method is below.
fromiter(iterable, dtype, count, like)
It has the following parameters.
iterable
- An iterable object that the function will iterate over.dtype
- This parameter refers to the data type of the returned array.count
- This is an optional integer parameter, and it refers to the number of elements that will be read in the iterable object. The default value of this parameter is-1
, which means that all the elements will be read.like
- This is an optional boolean parameter. It controls the definition of the returned array.
The way to filter elements using the fromiter()
method in NumPy is as follows.
import numpy as np
myArray = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9])
newArray = np.fromiter(
(element for element in myArray if element < 6), dtype=myArray.dtype
)
print(myArray)
print(newArray)
Output:
[1 2 3 4 5 6 7 8 9]
[1 2 3 4 5]
First, we initialize a NumPy Array from which we wish to filter the elements. Then we iterate over the whole array and filter out the values that are less than 6
. Then we cast this new array into a NumPy Array with the same data type as that of the original array.
To learn more about this method, refer to its official documentation
Filter Elements Using Boolean Mask Slicing Method in NumPy
This method is a bit weird but works like a charm in NumPy. We have to mention the condition inside the square or box brackets []
after the array. Then NumPy will filter out the elements based on the condition and return a new filtered array.
This concept might not be clear and even seem tricky to some, but don’t worry. We have some examples below to explain it a little better.
import numpy as np
myArray = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9])
newArray1 = myArray[myArray < 6] # Line 1
newArray2 = myArray[myArray % 2 == 0] # Line 2
newArray3 = myArray[myArray % 2 != 0] # Line 3
newArray4 = myArray[np.logical_and(myArray > 1, myArray < 5)] # Line 4
newArray5 = myArray[np.logical_or(myArray % 2 == 0, myArray < 5)] # Line 5
print(myArray)
print(newArray1)
print(newArray2)
print(newArray3)
print(newArray4)
print(newArray5)
Output:
[1 2 3 4 5 6 7 8 9]
[1 2 3 4 5]
[2 4 6 8]
[1 3 5 7 9]
[2 3 4]
[1 2 3 4 6 8]
As mentioned above, we add some conditions between the square brackets, and the target array was filtered based on those conditions. The variable storing the array, which is in this case myArray
, represents a single element of the array inside the square brackets.
To apply multiple conditions and use logical operators, we use two NumPy methods, namely, logical_and()
and logical_or()
for the logic and
or or
respectively.
myArray < 6
- It filters the values that are less than 6myArray % 2 == 0
- It filters the values that are divisible by 2myArray % 2 != 0
- It filters the values that are not divisible by 2np.logical_and(myArray > 1, myArray < 5)
- It filters the values greater than one and less than five.np.logical_or(myArray % 2 == 0, myArray < 5)
- It filters the values that are either divisible by two or less than five.
Filter Elements Using the where()
Method in NumPy
Here is the last method, which uses the where()
method from the NumPy library. It filters the elements from the target array based on a condition and returns the indexes of the filtered elements.
You can also use this method to change the values of elements that satisfy the condition.
The syntax of the where()
method is shown below.
where(condition, x, y)
It has the following parameters.
condition
- It is the boolean condition for which each element of the array is checked.x
- It is a value given to elements satisfying the condition or a computation carried on the satisfying elements.y
- It is a value given to elements not satisfying the condition or a computation carried on the unsatisfying elements.
Let’s see how to use this function to filter out the elements.
import numpy as np
myArray = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9])
newArray1 = myArray[np.where(myArray < 7)[0]]
newArray2 = myArray[np.where(myArray % 2 == 0)[0]]
newArray3 = myArray[np.where(myArray % 2 != 0)[0]]
print(myArray)
print(newArray1)
print(newArray2)
print(newArray3)
Output:
[1 2 3 4 5 6 7 8 9]
[1 2 3 4 5 6]
[2 4 6 8]
[1 3 5 7 9]
In the above snippet, all the elements that satisfy the condition were returned as an array.
The where()
function returns a tuple of NumPy arrays. So we only consider the first array, which is our answer.
As I mentioned above, you can also assign custom values and perform custom actions over elements when they satisfy the specified condition and when they not.
Below is an example of that.
import numpy as np
myArray = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9])
newArray1 = np.where(myArray < 7, 5, -1)
newArray2 = np.where(myArray % 2 == 0, myArray ** 2, 0)
newArray3 = np.where(myArray % 2 != 0, myArray, -1)
newArray4 = np.where(myArray % 2 != 0, myArray, myArray)
newArray5 = np.where(myArray % 2 != 0, 0, myArray)
print(myArray)
print(newArray1)
print(newArray2)
print(newArray3)
print(newArray4)
print(newArray5)
Output:
[1 2 3 4 5 6 7 8 9]
[ 5 5 5 5 5 5 -1 -1 -1]
[ 0 4 0 16 0 36 0 64 0]
[ 1 -1 3 -1 5 -1 7 -1 9]
[1 2 3 4 5 6 7 8 9]
[0 2 0 4 0 6 0 8 0]
Have a look at the output. See how the elements are changing based on conditions and based on values and calculations you provided to manipulate the elements to the where()
function.