How to Convert String to Float in NumPy
-
Convert String to Float in NumPy Using the
astype()
Method -
Convert String to Float in NumPy Using the
asarray()
Method -
Convert String to Float in NumPy Using the
asfarray()
Method
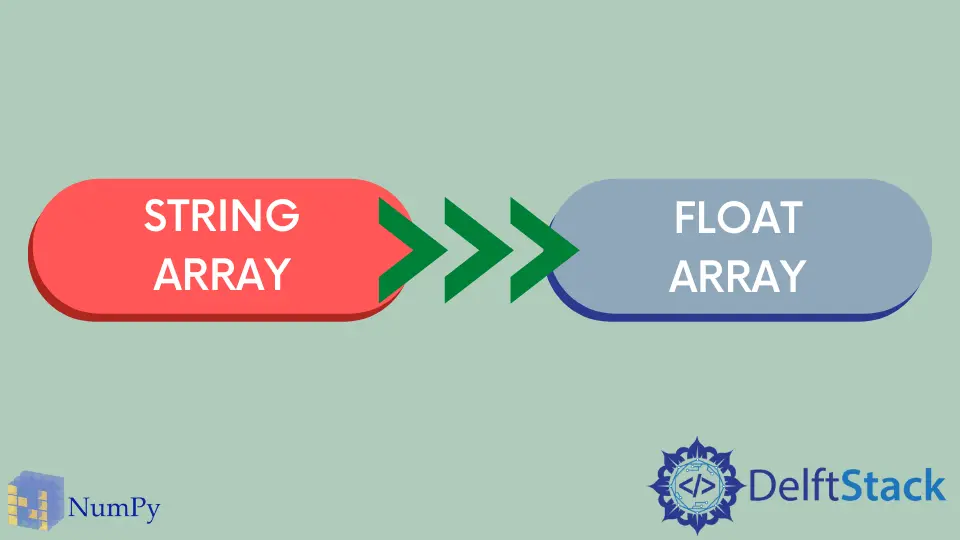
Conversions between numerical data and string data are a bit difficult to handle. But in Python, all this is almost effortless because Python has many inbuilt functionalities specially crafted to drive conversions like so.
Python even has a bunch of external libraries to handle these conversions with improved performance and speeds. NumPy is one such library.
NumPy is a Python library that is all about multidimensional arrays and matrices and all sorts of mathematical and logical computations that we can perform on them. This article will introduce how to convert a NumPy string array to a NumPy float array using NumPy itself.
Convert String to Float in NumPy Using the astype()
Method
astype
is an in-build class function for objects of type ndarray
. This method returns a copy of the ndarray
and casts it into a specified datatype.
The syntax of the astype()
method is below.
astype(dtype, order, casting, subok, copy)
It has the following parameters.
dtype
- It is the datatype to which the NumPy array will be cast to,order
- This is an optional parameter. This parameter controls the memory layout of the resulting array or the output.casting
- This is an optional parameter. This parameter controls the type of casting or conversion that will take place. By default, its value isunsafe
.subok
- This is an optional boolean parameter. It decides whether the output array will be of typenparray
orndarray
’s sub-classes.copy
- This is an optional boolean parameter. It decides if the output should be a newly allocated array or not.
You can use this method to get the conversion done. Refer to the following code snippet.
import numpy as np
stringArray = np.array(
["1.000", "1.235", "0.000125", "2", "55", "-12.35", "0", "-0.00025"]
)
floatArray = stringArray.astype(float)
print(stringArray)
print(floatArray)
Output:
['1.000' '1.235' '0.000125' '2' '55' '-12.35' '0' '-0.00025']
[ 1.000e+00 1.235e+00 1.250e-04 2.000e+00 5.500e+01 -1.235e+01
0.000e+00 -2.500e-04]
To learn more about this method, refer to its official documentation
Convert String to Float in NumPy Using the asarray()
Method
asarray()
is a NumPy function that converts the input array to a NumPy array of a specified type. The input array can be a Python’s list, tuples, tuples of lists, list of tuples, lists of lists, tuples of tuples, and a NumPy array itself.
The syntax of the asarray()
method is below.
asarray(a, dtype, order, like)
It has the following parameters.
a
- It is the input array that will be converted.dtype
- It refers to the datatype of the converted array. By default, this function automatically infers the data type from the input array.order
- This is an optional parameter. This parameter decides the memory layout of the converted array.like
- This is an optional boolean parameter. It controls the definition of the newly created array.
To learn more about this method, refer to the official documents
import numpy as np
stringArray = np.array(
["1.000", "1.235", "0.000125", "2", "55", "-12.35", "0", "-0.00025"]
)
floatArray = np.asarray(stringArray, dtype=float)
print(stringArray)
print(floatArray)
Output:
['1.000' '1.235' '0.000125' '2' '55' '-12.35' '0' '-0.00025']
[ 1.000e+00 1.235e+00 1.250e-04 2.000e+00 5.500e+01 -1.235e+01
0.000e+00 -2.500e-04]
Convert String to Float in NumPy Using the asfarray()
Method
Lastly, it is the asfarray()
. You might have already guessed what this method does. It converts the input array to a NumPy of type float.
The syntax of the asfarray()
method is below.
asfarray(a, dtype)
This method has only two parameters.
a
- It is the input array that will convert to a float array.dtype
- It is an optional parameter and refers to the data type of the output array. It can bestr
or one of the float datatypes. If its value is one of the integer datatypes, it is automatically replaced with datatypefloat64
.
import numpy as np
stringArray = np.array(
["1.000", "1.235", "0.000125", "2", "55", "-12.35", "0", "-0.00025"]
)
floatArray = np.asfarray(stringArray, dtype=float)
print(stringArray)
print(floatArray)
Output:
['1.000' '1.235' '0.000125' '2' '55' '-12.35' '0' '-0.00025']
[ 1.000e+00 1.235e+00 1.250e-04 2.000e+00 5.500e+01 -1.235e+01
0.000e+00 -2.500e-04]
Refer to the official documents to learn more.