How to Convert Datetime64 to Datetime.Datetime or Timestamp in NumPy
- the Problem
-
Convert
datetime.datetime
tonp.datetime64
andpd.Timestamp
-
Convert
np.datetime64
todatetime.datetime
andpd.Timestamp
-
Convert
Timestamp
todatetime
anddatetime64
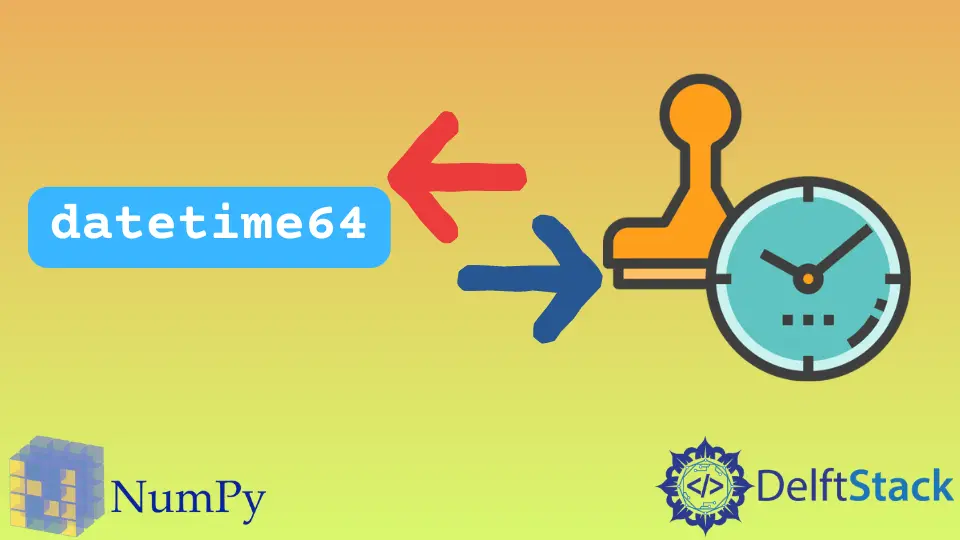
This article aims to demonstrate how to convert data between numPy.datetim64
, datetime.datetime
and Timestamp
.
the Problem
When dealing with data - be it ordered or unordered, coming across date and time is a fairly common occurrence. Depending on the situation, the time and date must be dealt with in a suitable manner to ensure that the processed data is in a desirable format.
Code Example:
import datetime
import numpy as np
import pandas as pd
dt = datetime.datetime(2022, 5, 21)
ts = pd.DatetimeIndex([dt])[0]
dt64 = np.datetime64(dt)
In the code above, we have imported three modules named datetime
, numpy
, and pandas
, each providing their implementation for storing and processing dates (each has its own distinct use cases).
Using datetime.datetime()
, we create a datetime
instance and store it in a variable named dt
. As for pandas
, we used the method pd.DatetimeIndex()
, which creates an immutable array storing n
number of datetime64
instances and accessing the first member of the array returns a Timestamp
instance.
Coming to the pandas
module, we use the datetime64()
method to convert the datetime
instance and store it in a variable named dt64
.
It is easy to convert datetime
to Timestamp
and datetime64
instances. In this article, we will now see how we can convert np.datetime64
to datetime
and Timestamp
instances.
Convert datetime.datetime
to np.datetime64
and pd.Timestamp
Code Example:
import datetime
import numpy as np
import pandas as pd
dt = datetime.datetime(
year=2018, month=10, day=24, hour=4, minute=3, second=10, microsecond=7199
)
# convert datetime.datetime to np.datetim364
print(np.datetime64(dt))
# datetime.datetime to pd.Timestamp
print(pd.Timestamp(dt))
Output:
2018-10-24T04:03:10.007199
2018-10-24 04:03:10.007199
Convert datetime. datetime
to datetime64
and Timestamp
by passing the datetime
instance to the constructors of datetime64
and Timestamp
, which will be converted.
Convert np.datetime64
to datetime.datetime
and pd.Timestamp
Code Example:
import datetime
import numpy as np
import pandas as pd
# np.datetime64 to datetime.datetime
dt64 = np.datetime64("2017-10-24 05:34:20.123456")
unix_epoch = np.datetime64(0, "s")
one_second = np.timedelta64(1, "s")
seconds_since_epoch = (dt64 - unix_epoch) / one_second
print(datetime.datetime.utcfromtimestamp(seconds_since_epoch))
# np.datetime64 to Timestamp
print(pd.Timestamp(dt64))
Output:
2017-10-24 05:34:20.123456
2017-10-24 05:34:20.123456
To convert from np.datetime64
to datetime.datetime
, we must first convert the datetime64
data to seconds (in epoch format). After conversion, the seconds are passed to the datetime
method named utcfromtimestamp
, which reads the epoch time and converts it to an instance of datetime
.
As for np.datetime64
to Timestamp
, it is relatively simple, just pass the np.datetime64
instance to the Timestamp
constructor.
Convert Timestamp
to datetime
and datetime64
Code Example:
import datetime
import numpy as np
import pandas as pd
ts = pd.Timestamp("2017-10-24 04:24:33.654321")
# Timestamp to datetime.datetime
print(ts.to_pydatetime())
# Timestamp to np.datetime64
print(ts.to_datetime64())
Output:
2017-10-24 04:24:33.654321
2017-10-24T04:24:33.654321000
With the help of these functions, Timestamp
can be easily converted to datetime
and datetime64
with just a single method call. To convert from Timestamp
to datetime.datetime
, use the to_pydatetime()
method, It converts the Timestamp
instance to a datetime. datetime
instance.
As for conversion from Timestamp
to datetime
, use the to_datetime64()
method. It converts the Timestamp
instance to an np.datetime64
instance.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn