How to Find the First Index of Element in NumPy Array
-
Using NumPy’s
np.where()
Function -
Using
list.index()
Method - Using a Loop for Custom Logic
- Conclusion
- FAQ
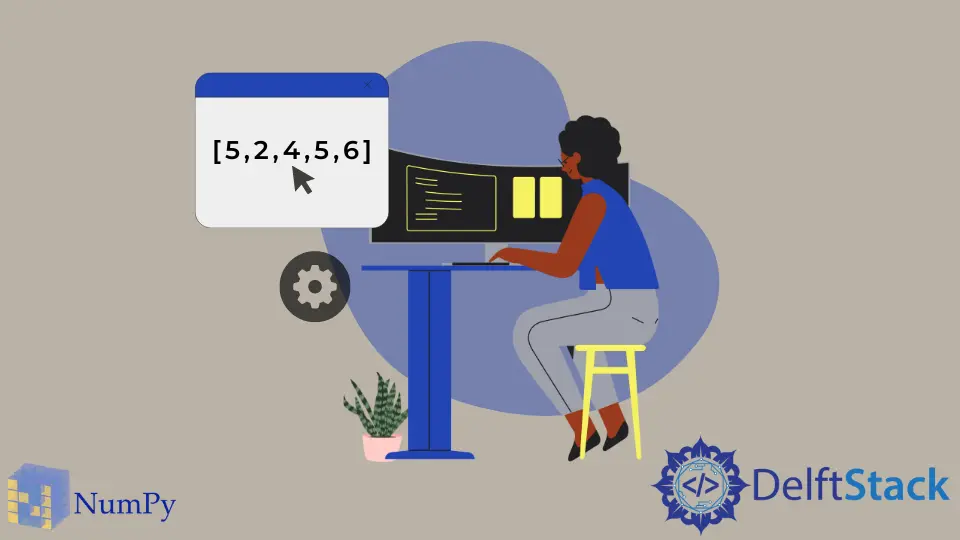
Finding the index of an element in a NumPy array can be a common task in data analysis and scientific computing. Whether you’re working with large datasets or performing complex calculations, knowing how to efficiently locate elements is crucial.
In this tutorial, we will explore various methods to find the first index of an element in a NumPy array using Python. We’ll cover straightforward techniques that allow you to quickly retrieve the desired index, making your coding experience smoother and more efficient. Let’s dive into the world of NumPy and discover how to effectively find indices in your arrays.
Using NumPy’s np.where()
Function
One of the simplest and most effective methods to find the first index of an element in a NumPy array is by utilizing the np.where()
function. This function returns the indices of elements that satisfy a given condition. To find the first occurrence, you can simply access the first element of the result.
Here’s a quick example:
import numpy as np
array = np.array([10, 20, 30, 40, 50, 20])
element_to_find = 20
index = np.where(array == element_to_find)[0][0]
Output:
1
In this example, we first import the NumPy library and create an array containing some integers. We then specify the element we want to find, which is 20
. The np.where()
function checks the condition array == element_to_find
and returns the indices where this condition is true. By accessing the first element of the returned array with [0][0]
, we obtain the first index of 20
, which in this case is 1
.
This method is efficient and easy to understand, making it a popular choice for many Python developers. However, it’s important to note that if the element is not found in the array, this approach will raise an IndexError
. To handle such cases gracefully, you can add a condition to check if the result is empty before trying to access the index.
Using list.index()
Method
If you prefer working with Python lists, you can convert your NumPy array to a list and then use the built-in list.index()
method to find the index of the first occurrence of an element. This method is straightforward and works well for smaller datasets.
Here’s how to implement this:
import numpy as np
array = np.array([10, 20, 30, 40, 50, 20])
element_to_find = 20
index = list(array).index(element_to_find)
Output:
1
In this code snippet, we first convert the NumPy array into a standard Python list using the list()
function. We then call the index()
method on this list, passing in the element we want to find. The index()
method will return the first index of the specified element. In our example, the index of 20
is 1
.
While this method is simple and effective, it may not be the best choice for large arrays due to the overhead of converting the array to a list. Additionally, if the element is not found, this method will raise a ValueError
. Therefore, it’s wise to ensure that the element exists in the array before attempting to find its index.
Using a Loop for Custom Logic
For those who prefer a more manual approach or need to implement custom logic, using a loop to iterate through the elements of the array can be a good solution. This method provides flexibility and can be tailored to specific requirements.
Here’s an example:
import numpy as np
array = np.array([10, 20, 30, 40, 50, 20])
element_to_find = 20
index = -1
for i in range(len(array)):
if array[i] == element_to_find:
index = i
break
Output:
1
In this example, we loop through each index of the array using a for
loop. We check if the current element matches the element_to_find
. If it does, we assign the current index to the index
variable and break out of the loop. This way, we ensure that we capture the first occurrence of the element.
Using a loop can be beneficial when you need to implement additional conditions or when working with complex data structures. However, this approach may be less efficient than the previous methods for large arrays, as it involves checking each element until a match is found.
Conclusion
Finding the first index of an element in a NumPy array can be accomplished through various methods, each with its own advantages and trade-offs. Whether you choose to use the np.where()
function for its simplicity, the list.index()
method for its straightforwardness, or a manual loop for more control, the key is to select the method that best suits your needs. By mastering these techniques, you’ll enhance your data manipulation skills and streamline your coding process in Python. Happy coding!
FAQ
-
How do I find the index of an element that does not exist in the array?
You can handle this by checking if the result fromnp.where()
is empty or using a try-except block around thelist.index()
method to catch theValueError
. -
Can I find multiple occurrences of an element in a NumPy array?
Yes, you can usenp.where()
without accessing the first element to get all indices where the element occurs. -
Is it faster to use NumPy methods compared to Python lists?
Generally, yes. NumPy is optimized for performance with large datasets, making it faster than standard Python lists for numerical operations. -
What should I do if I need to find the last index of an element?
You can usenp.where()
and then access the last element of the result by using[-1]
instead of[0]
. -
Are there any alternatives to NumPy for finding indices in arrays?
Other libraries like Pandas can also be used for similar tasks, especially when dealing with tabular data.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn