Imaginary Numbers in NumPy Arrays
- Understanding NumPy and Imaginary Numbers
- Creating a NumPy Array with Imaginary Numbers
- Performing Mathematical Operations on Imaginary Numbers
- Accessing Real and Imaginary Parts of Complex Numbers
- Conclusion
- FAQ
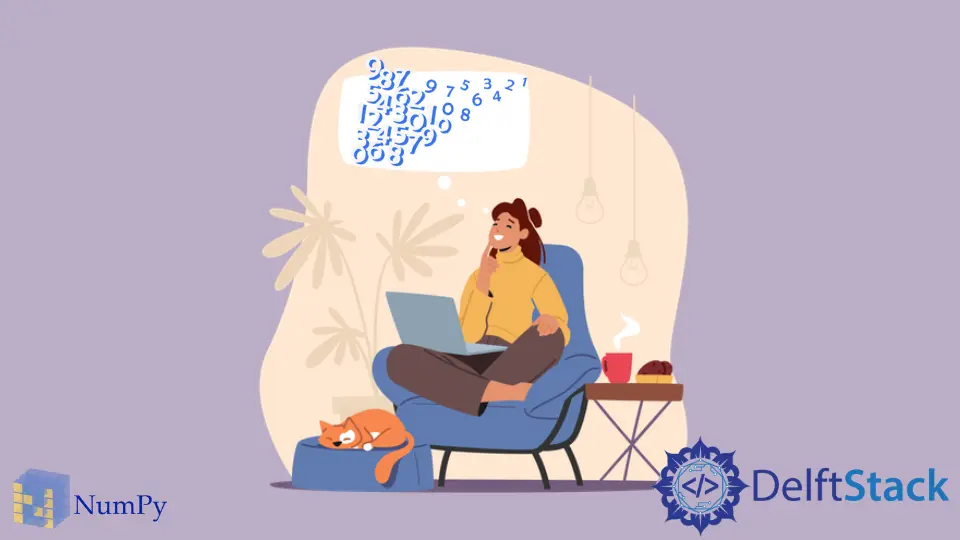
When diving into the world of numerical computing, you might encounter various types of numbers, including real and imaginary numbers. Imaginary numbers play a crucial role in complex mathematics and engineering applications. If you’re using Python, the NumPy library is your go-to tool for handling arrays, including those that contain imaginary numbers.
This tutorial will guide you through the process of storing and manipulating imaginary numbers in NumPy arrays. By the end, you’ll have a solid understanding of how to work with these fascinating numbers in your numerical computations.
Understanding NumPy and Imaginary Numbers
Before we jump into the practical aspects, let’s clarify what imaginary numbers are. An imaginary number is defined as a number that can be expressed as a real number multiplied by the imaginary unit, denoted as ‘i’, where i² = -1. In Python, you can easily create imaginary numbers by appending ‘j’ to a real number. NumPy extends this capability, allowing you to store and manipulate arrays of complex numbers efficiently.
Creating a NumPy Array with Imaginary Numbers
Creating a NumPy array that includes imaginary numbers is straightforward. You can directly specify complex numbers in the array. Here’s how you can do it:
import numpy as np
# Create an array with imaginary numbers
imaginary_array = np.array([1 + 2j, 3 + 4j, 5 + 6j])
print(imaginary_array)
Output:
[1.+2.j 3.+4.j 5.+6.j]
In this example, we import NumPy and create an array named imaginary_array
that contains three complex numbers. Each number consists of a real part and an imaginary part. The output confirms that the array holds the complex numbers as expected.
When creating a NumPy array with imaginary numbers, it’s essential to use the ‘j’ notation for the imaginary part. NumPy recognizes this and treats the elements as complex numbers, allowing for various mathematical operations.
Performing Mathematical Operations on Imaginary Numbers
Once you have your imaginary numbers stored in a NumPy array, you can perform a variety of mathematical operations. For instance, you can add, subtract, multiply, and divide complex numbers. Here’s an example that demonstrates some basic arithmetic operations:
# Define another array with imaginary numbers
another_array = np.array([2 + 3j, 4 + 5j, 6 + 7j])
# Perform operations
addition = imaginary_array + another_array
subtraction = imaginary_array - another_array
multiplication = imaginary_array * another_array
division = imaginary_array / another_array
print("Addition:", addition)
print("Subtraction:", subtraction)
print("Multiplication:", multiplication)
print("Division:", division)
Output:
Addition: [3.+5.j 7.+9.j 11.+13.j]
Subtraction: [-1.-1.j -1.-1.j -1.-1.j]
Multiplication: [-4.+9.j -8.+43.j -12.+67.j]
Division: [0.61538462+0.07692308j 0.71428571+0.14285714j 0.83333333+0.16666667j]
In this code snippet, we define another array called another_array
, which also contains complex numbers. We then perform addition, subtraction, multiplication, and division between the two arrays. The results show how NumPy seamlessly handles these operations with complex numbers. Each operation yields a new array, demonstrating the flexibility of NumPy for numerical computations involving imaginary numbers.
Accessing Real and Imaginary Parts of Complex Numbers
One of the powerful features of NumPy is its ability to easily access the real and imaginary parts of complex numbers stored in an array. This can be particularly useful when you need to analyze or manipulate these components separately. Here’s how you can do that:
# Access real and imaginary parts
real_parts = imaginary_array.real
imaginary_parts = imaginary_array.imag
print("Real Parts:", real_parts)
print("Imaginary Parts:", imaginary_parts)
Output:
Real Parts: [1. 3. 5.]
Imaginary Parts: [2. 4. 6.]
In this example, we use the .real
and .imag
attributes of the NumPy array to extract the real and imaginary components, respectively. The output confirms that we successfully retrieved the real parts (1, 3, 5) and the imaginary parts (2, 4, 6) of the complex numbers. This functionality is particularly valuable for applications that require separate handling of real and imaginary components, such as signal processing or control systems.
Conclusion
In this tutorial, we explored how to store and manipulate imaginary numbers in NumPy arrays. We learned how to create arrays with complex numbers, perform mathematical operations, and access their real and imaginary components. NumPy’s capabilities make it an excellent choice for anyone looking to work with complex numbers in Python. Whether you’re tackling engineering problems or delving into complex analysis, understanding how to handle imaginary numbers in NumPy will undoubtedly enhance your computational skills.
FAQ
-
how do I create a NumPy array with complex numbers?
You can create a NumPy array with complex numbers by using the ‘j’ notation for the imaginary part, like this:np.array([1 + 2j, 3 + 4j])
. -
can I perform arithmetic operations on complex numbers in NumPy?
Yes, NumPy allows you to perform various arithmetic operations such as addition, subtraction, multiplication, and division on complex numbers stored in arrays. -
how can I access the real and imaginary parts of complex numbers in a NumPy array?
You can access the real parts using the.real
attribute and the imaginary parts using the.imag
attribute of the NumPy array. -
what are some applications of imaginary numbers in real-world scenarios?
Imaginary numbers are widely used in fields such as electrical engineering, control systems, and signal processing, where they help analyze waveforms and oscillations. -
is it possible to visualize complex numbers using NumPy?
Yes, you can visualize complex numbers using libraries like Matplotlib in combination with NumPy to create plots that represent complex data.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn