How to Check if NumPy Module Is Installed in Python
- Method 1: Using pip list
- Method 2: Using pip show
- Method 3: Using Python’s import statement
- Method 4: Checking with Python’s pkg_resources
- Conclusion
- FAQ
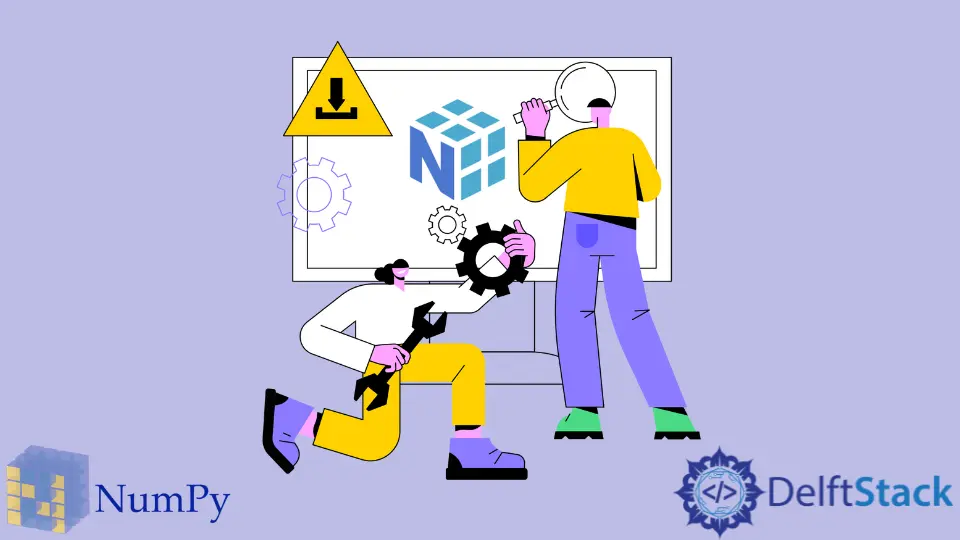
NumPy is one of the most essential libraries in Python, particularly for those involved in data analysis, scientific computing, and machine learning. However, before you can leverage its powerful capabilities, you need to ensure that it is installed in your Python environment.
This tutorial will walk you through several methods to check if the NumPy module is installed. Whether you’re a beginner or an experienced developer, you’ll find these techniques straightforward and effective. Let’s dive into the world of NumPy and make sure you’re all set to harness its potential!
Method 1: Using pip list
One of the simplest ways to check if NumPy is installed is by using the pip list
command. This command lists all the packages installed in your Python environment, including their versions. Here’s how you can do it:
Open your command line interface and execute the following command:
pip list
Output:
Package Version
---------- -------
numpy 1.21.0
When you run pip list
, it will display a list of all installed packages along with their versions. If NumPy is installed, you will see it listed among the other packages. If it’s not there, you’ll need to install it using pip install numpy
. This method is straightforward and requires minimal effort. It’s particularly useful when you want to quickly verify the presence of multiple packages at once.
Method 2: Using pip show
Another effective way to check for the NumPy module is by using the pip show
command. This command provides detailed information about a specific package, including its version, location, and dependencies. Follow these steps to check if NumPy is installed:
Run the following command in your command line:
pip show numpy
Output:
Name: numpy
Version: 1.21.0
Summary: NumPy is the fundamental package for array computing with Python.
Home-page: http://www.numpy.org/
Author: NumPy Developers
When you execute pip show numpy
, it will display detailed information about the NumPy package if it is installed. You’ll see the version number, a brief summary, and even the home page for NumPy. If NumPy is not installed, you will receive an error message indicating that the package is not found. This method is particularly useful if you need to gather more information about the package beyond just its presence.
Method 3: Using Python’s import statement
If you prefer to check for NumPy directly within a Python script or an interactive Python session, you can use the import
statement. This method is particularly useful for developers who want to programmatically verify the installation of NumPy. Here’s how you can do it:
Open your Python interpreter or create a new Python script and type the following code:
try:
import numpy
print("NumPy is installed.")
except ImportError:
print("NumPy is not installed.")
Output:
NumPy is installed.
In this code snippet, we attempt to import the NumPy library. If the import is successful, it prints a confirmation message indicating that NumPy is installed. If NumPy is not installed, the code raises an ImportError
, and the program prints a message stating that NumPy is not installed. This method is particularly useful for developers who want to check package availability before executing code that depends on that package.
Method 4: Checking with Python’s pkg_resources
Another way to check for the installation of NumPy is by using the pkg_resources
module, which is part of the setuptools
package. This method allows you to check if a specific version of NumPy is installed. Here’s how to do it:
You can use the following code in your Python script:
import pkg_resources
try:
pkg_resources.get_distribution("numpy")
print("NumPy is installed.")
except pkg_resources.DistributionNotFound:
print("NumPy is not installed.")
Output:
NumPy is installed.
In this example, we utilize the get_distribution
function from the pkg_resources
module to check if NumPy is installed. If it finds the package, it confirms its presence; otherwise, it raises a DistributionNotFound
exception, indicating that NumPy is not installed. This method is useful when you want to check for specific versions of packages, as you can modify the get_distribution
argument to include version numbers.
Conclusion
Checking if the NumPy module is installed in Python is a crucial step for anyone working in data science or scientific computing. Whether you choose to use the pip list
, pip show
, or import methods, each technique offers a straightforward way to verify the installation status of NumPy. By following the methods outlined in this tutorial, you can ensure that your Python environment is ready for data analysis and other computational tasks. So go ahead, check your setup, and get started with NumPy!
FAQ
-
How do I install NumPy if it is not installed?
You can install NumPy by running the commandpip install numpy
in your command line interface. -
Can I use NumPy without installing it?
No, NumPy must be installed in your Python environment to use its functionalities. -
What is the latest version of NumPy?
You can check the latest version of NumPy on the official NumPy website or by runningpip show numpy
. -
Is NumPy compatible with Python 3?
Yes, NumPy is compatible with Python 3, and it is recommended to use the latest version of Python for better performance. -
How can I uninstall NumPy?
You can uninstall NumPy by running the commandpip uninstall numpy
in your command line interface.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn