How to Run Node Js and Apache on the Same Server
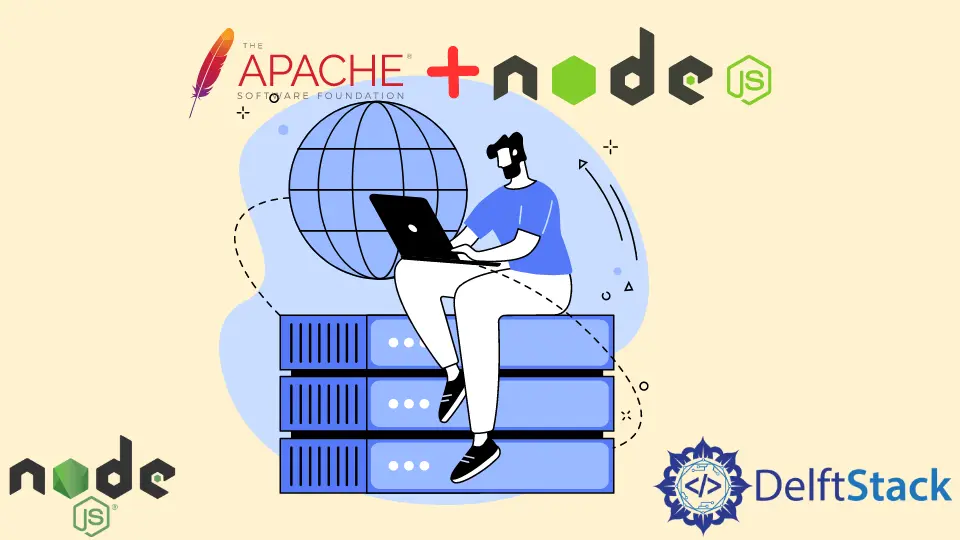
The Apache
is one of the most popular web servers that allow us to process client requests and serve content through HTTP. Apache is open source and easy to use alongside other technologies such as MYSQL and PHP.
The Apache server is configured to listen on port
by default. However, it can also listen on port 443
using the TLS configuration.
Node JS is a JavaScript runtime that creates server-side applications in JavaScript code. Node JS also enables us to create simple HTTP servers that we can use to serve static content through various HTTP
methods.
The HTTP
module in Node JS allows us to create a server that listens to a specific port number and responds to the client. Here is how we can create a simple server using the HTTP.createServer()
method provided by the HTTP
module.
const http = require('http');
const requestListener =
function(req, res) {
res.writeHead(200);
res.end('Hello, World!');
}
const server = http.createServer(requestListener);
server.listen(8080);
We have created a function that accepts the request and response object as parameters in the example above. The response object returns a 200 response
code and a simple payload with Hello world
text.
Finally, we have also created a server using the HTTP.createServer()
method that calls the requestListener
object when a request is made. Using the listen()
method, we can get the server to listen on port 8080
.
We cannot serve the same request since both the Node JS server and Apache are configured to run on different ports. Although we can change the port numbers and run on different ones, computers are engineered to have only one process listening on one port and not more.
However, we can opt to have the Apache server run as a reverse proxy server
and have the Node JS server solely responsible for serving client requests. A proxy server is an intermediary server used to direct requests to different servers.
A reverse proxy server
would usually sit behind a firewall and are commonly used for load balancing, providing acceleration through caching and compressing inbound data, and providing additional security using SSL encryption.
To get started, we will first create a simple Node JS server listening on port 8080
, such as the one we created earlier.
const http = require('http');
const requestListener =
function(req, res) {
res.writeHead(200);
res.end('Hello, World!');
}
const server = http.createServer(requestListener);
server.listen(8080);
I have used index.js
as the main file in my code. However, you may name it as you wish.
I will run the command below to get the server up and running.
node index.js
To view the response from the server, I’m going to navigate to http://localhost:8080/
.
We will use the ProxyPass
directive in the Apache httpd.conf
to pipe all requests on a particular URL to our Node.JS application.
This method allows us to map remote servers into a local server by making the local server act as a proxy. The local server in this context is referred to as a reverse proxy or gateway
.
However, we will first need to download and install XAMPP
from Apache Friends to get started. XAMPP
is a cross-platform web server solution that mostly consists of a MariaDB and Apache HTTP Server.
Now that XAMPP is installed open the XAMPP control panel and start the Apache service by clicking on Start. Then, click the Config button after the Admin button and select the Apache httpd.conf
option.
It will open a notepad document. The main Apache HTTP server configuration file contains configuration directives that give the server its instructions.
To map our Node JS server to the Apache server, we will add the URL /isaactonyloi http://localhost:8080/
and the ProxyPass directive at the beginning, just the server root URL as shown below. Do not forget to save.
Finally, we must enable two modules under the same document under the Dynamic shared
object.
We will enable two objects, namely proxy_http_module modules/mod_proxy_http.so
and proxy_module modules/mod_proxy.so
by simply removing the comment symbol #
and having them appear shown below.
You may find that the proxy_module modules/mod_proxy.
is already enabled. For the changes to take effect, we need to save them and restart our Apache server by clicking the Stop button and clicking the Start button again in the control panel.
Now to access the response from the Node JS server using the Apache HTTP server as a proxy visit, use the URL http://localhost/isaactonyloi
. The response should appear as shown below.
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedIn