How to Render HTML Files in Node.js
- Internally Render HTML File in Node.js
-
Use the
readFile()
Method to Render HTML Files in Node.js -
Use the
fs.createReadStream()
Method to Render HTML Files in Node.js - Conclusion
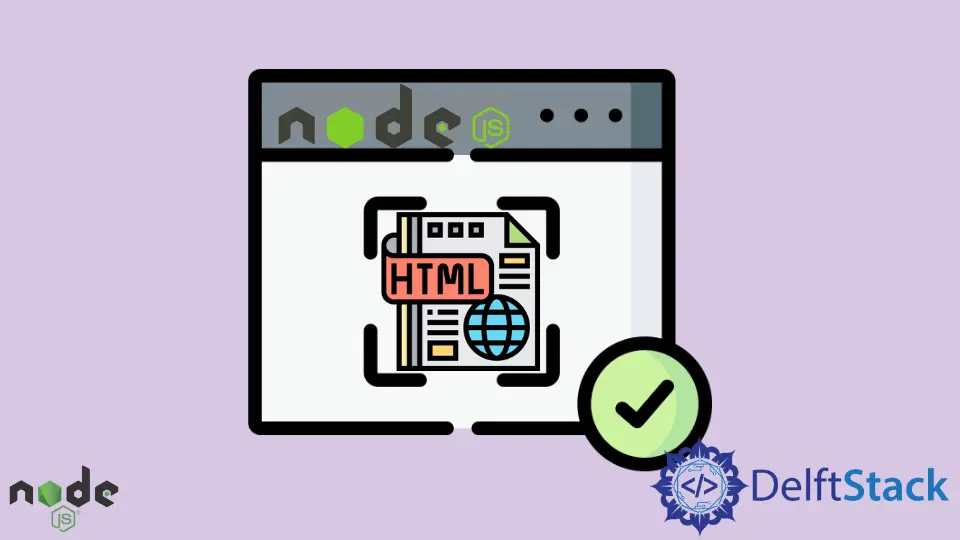
In this article, we will create a simple Node.js server and explore different methods that we can use to render an HTML page.
Internally Render HTML File in Node.js
Node.js is an open-source JavaScript runtime that allows us to use JavaScript on the server.
Using Node.js, we can do the following.
- Create, read, open, close, and delete files on the server.
- Add, modify and delete data in the database.
- Create web applications, video streaming apps, chat applications that render content dynamically.
To build fully-fledged web applications, we have to create server-static files on the client-side (includes JavaScript, CSS, images, and HTML files).
You must have Node.js pre-installed in your system. We will create a simple HTTP server instance using the common http.createServer()
function.
This function accepts an options argument and a callback function that takes in the request and response objects as parameters. We will send a response containing the headers and some content using the response object.
In this case, we will return a simple HTML tag to the user when a request is made to the server.
Finally, we will also use the server.listen()
method to make the server listen on port 8080
for the incoming requests. You can change the port number if another process uses this one.
const http = require('http');
const port = 8080;
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/html');
res.end('<h1>Hello, User!</h1> <h3> Welcome Home</h3>\n');
});
server.listen(port, () => {
console.log(`Server is running on port number::${port}`);
});
If we start the server and visit http://localhost:8080/
, the response below shows that the HTML file has been successfully rendered.
Although this method is precise, it is not the best way of serving HTML files in Node.js because when building real applications, we usually have very large HTML files that are best stored in an external file.
Use the readFile()
Method to Render HTML Files in Node.js
We can start by creating a file in our root directory that will contain an HTML file (see the code below).
<!DOCTYPE html>
<html lang="en">
<head>
<title>simple page</title>
</head>
<body>
<h1>Hello, User!</h1>
<h3> Welcome Home !</h3>
</body>
</html>
We will name it index.html
and write out the HTML file. Here is how the file structure should look after creating the index.html
file.
We will use the fs
module to read this file into the main file index.js
and render it to the user. Since fs
is an inbuilt method, we need to include it in the main file using the require()
function.
The fs
module provides the fs.ReadFile()
method that we can use to read files by simply passing in the path, encoding and a callback function that will be called with the file and handle the error (if any) as the parameters.
We will still use the http.createServer()
function to create the request and response objects. We will attach the HTML file to the response object, as shown here.
let http = require('http');
let fs = require('fs');
let port = 8080;
const server = http.createServer((request, response) => {
response.writeHead(200, {'Content-Type': 'text/html'});
fs.readFile('./index.html', null, function(error, data) {
if (error) {
response.writeHead(404);
respone.write('Whoops! File not found!');
} else {
response.write(data);
}
response.end();
});
});
server.listen(port, () => {
console.log(`Server is listening on port number: ${port}`);
});
If we start the server and visit http://localhost:8080/
, the response below shows that the HTML file has been successfully rendered.
We have explored creating a simple Node.js server with a simple HTML page in this example. Alternatively, we can use readable streams in Node.js provided by the stream module instead of the traditional fs
module.
Use the fs.createReadStream()
Method to Render HTML Files in Node.js
Node.js streams are both memory efficient and time-efficient.
Unlike the traditional way of reading a file where the whole file must be read before processing it, Node.js streams allow us to read content piece by piece without keeping it in memory.
The fs
module provides us with the fs.createReadStream()
function that accepts the path parameter that holds the path to our HTML file in the root directory.
The second parameter is an optional parameter that holds a string object. This function allows us to open a readable stream, which we can then pipe from the source to the destination using the pipe()
method.
Here is how we can implement this.
const http = require('http');
const fs = require('fs');
const port = 8080;
const server = http.createServer((req, res) => {
if (req.url === '/') {
const htmlFile = 'index.html';
res.statusCode = 200;
res.setHeader('Content-Type', 'text/html');
fs.createReadStream(htmlFile).pipe(res);
}
});
server.listen(port, () => {
console.log(`The serer is running at port number::${port}`);
});
If we start the server and visit http://localhost:8080/
, the response below shows that the HTML file has been successfully rendered.
Conclusion
In conclusion, if you’re looking to develop web applications, at some point, you may probably have to learn a Node.js framework. Express.js is one of the most commonly used frameworks.
Express.js is a minimalist framework that provides robust features for developing web and mobile applications. Express.js offers the best solutions for serving static files using the express.static()
middleware function.
This function accepts root and options parameters, where root specifies the root directory from which we can serve the static files such as HTML and CSS.
Express also allows you to work with template engines such as pug
, jade
, and ejs
, making it even easier to design HTML pages.
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedIn