How to Parse XML in NodeJS
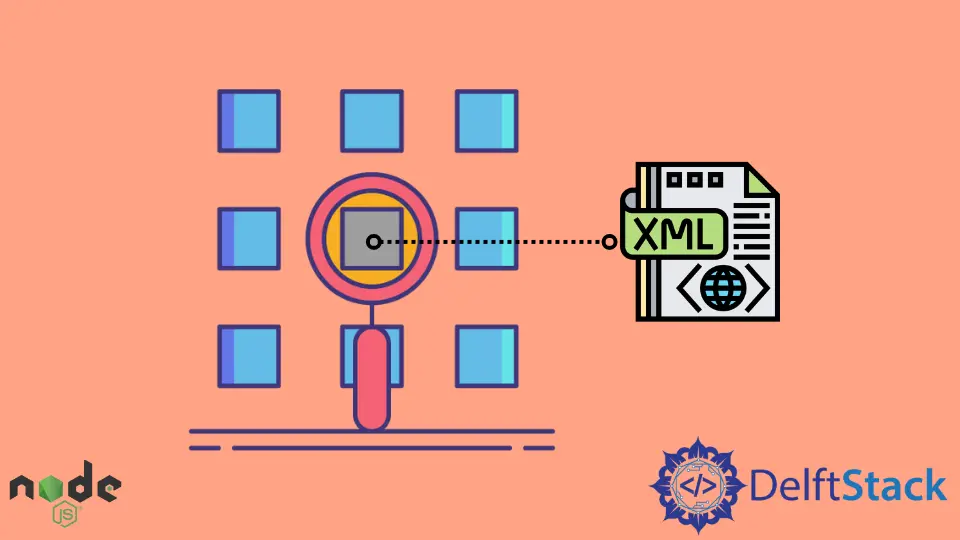
XML stands for Extensible Markup Language. It is a simple text-based format designed to store and transfer data.
Using the XML Parsing in NodeJS
XML parsing is the process of reading and modifying XML
data so that client applications can efficiently work with the data.
Besides reading XML
documents, parsers also verify that a given XML
document conforms to the standard XML
syntax and checks for any document violations or errors.
Parsers perform this validation by checking and validating that components of an XML document conform to the Document Type Definition(DTD)
or the schema pattern.
One of the commonly used and straightforward libraries that we can use to parse XML is xml2js
. It is a simple bidirectional XML to javascript object converter that uses sax-js
and xmlbuilder-js
.
The fastest and most straightforward way to install xml2js
and all its dependencies is by using the node package manager(npm)
using the command below.
npm install xml2js
We will first create a skeleton node applications server with an index.js
file to write our code.
Since we have installed xml2js
as a separate module, we will use the require()
function to include it in the main file index.js
and have it as one of the dependencies enlisted in the package.json
file.
Once the module is in place, we can expose the Parse object and parse the XML file. The code below shows that we can parse them for small XML files.
var parseString = require('xml2js').parseString;
var xml =
'<root><railroad><gate>mile</gate><actually><wall>about</wall><whether>whom</whether><generally>choose</generally><alive>-110838449.82186222</alive><dug>1150240574</dug><keep>1763043002.325139</keep></actually><hello>flower</hello><strike>-914757363.3499522</strike><danger>-200144457</danger> <whose>1022707429</whose></railroad><stove>1072975804</stove><row>-1686689624.6101847</row><changing>96738623.18869686</changing><compass>917572500</compass><die>suggest</die></root>'
parseString(xml, function(err, result) {
console.dir(result);
});
Output:
root: {
railroad: [ [Object] ],
stove: [ '1072975804' ],
row: [ '-1686689624.6101847' ],
changing: [ '96738623.18869686' ],
compass: [ '917572500' ],
die: [ 'suggest' ]
}
}
However, this may not be the ideal method of parsing XML for huge files if we write production standard code. We can use the fs.readFileSync()
to read the XML file synchronously before parsing it using the fs
module.
The fs.readFileSync()
is a built-in API for the fs module that returns the contents of a file synchronously. Once we have the file in place, we can use the parsed object to parse the file, as shown below.
Using the Parsed Object to Parse the File
const xml2js = require('xml2js');
const fs = require('fs');
const parser = new xml2js.Parser({attrkey: 'ATTR'});
let xml_file = fs.readFileSync('file.xml', 'utf8');
parser.parseString(xml_file, (error, result) => {
if (error === null) {
console.log(result);
} else {
console.log(error);
}
});
Output:
{
root: {
railroad: [ [Object] ],
stove: [ '1072975804' ],
row: [ '-1686689624.6101847' ],
changing: [ '96738623.18869686' ],
compass: [ '917572500' ],
die: [ 'suggest' ]
}
}
We can also read the file asynchronously and then parse it, as shown in the code below.
const xml2js = require('xml2js');
const fs = require('fs');
const parser = new xml2js.Parser({attrkey: 'ATTR'});
fs.readFile('file.xml', (err, data) => {
if (!err) {
parser.parseString(data, function(error, result) {
if (error === null) {
console.log(result);
} else {
console.log(error);
}
});
}
});
Output:
{
root: {
railroad: [ [Object] ],
stove: [ '1072975804' ],
row: [ '-1686689624.6101847' ],
changing: [ '96738623.18869686' ],
compass: [ '917572500' ],
die: [ 'suggest' ]
}
}
Suppose the source of this XML data is a specific endpoint. Then, in that case, we will first need to send an HTTP GET
request to that particular endpoint.
As demonstrated below, this endpoint’s response will then be passed to the xml2js
Parse object for parsing.
const https = require('https');
const xml2js = require('xml2js');
const parser = new xml2js.Parser({attrkey: 'ATTR'});
let req = https.get(
'https://4f567984-6c81-4a85-8b5a-61ab9621f67f.mock.pstmn.io/dishon/file.xml',
function(res) {
let xml_data = '';
res.on('data', (stream) => {
xml_data = xml_data + stream;
});
res.on('end', () => {
parser.parseString(xml_data, (error, result) => {
if (error === null) {
console.log(result);
} else {
console.log(error);
}
});
});
});
Output:
{
root: {
railroad: [ [Object] ],
stove: [ '1072975804' ],
row: [ '-1686689624.6101847' ],
changing: [ '96738623.18869686' ],
compass: [ '917572500' ],
die: [ 'suggest' ]
}
}
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedIn