The next() Function in NodeJS
-
Use the
next()
Function to Write CustomMiddleware
Functions in ExpressJS -
Use the
next()
Function to Pass Control to the NextMiddleware
Function in ExpressJS -
Use the
next(err)
Function to Handle Errors in a Route Using ExpressJS
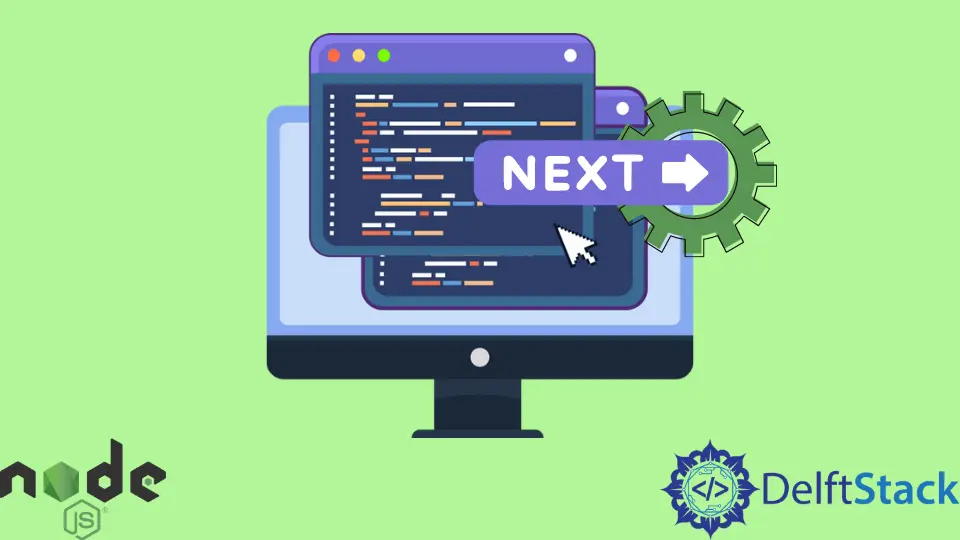
The next()
function is not part of Node js core but is used widely in Express js as middleware functions.
Use the next()
Function to Write Custom Middleware
Functions in ExpressJS
The next()
is useful when writing custom middleware functions in Express js and allows us to pass control from one middleware function to another.
Express js is a minimalist framework that provides robust features for developing web and mobile applications.
Express js makes heavy use of middleware functions and is often referred to as a routing and middleware framework.
On the other hand, middleware functions have access to the response and request cycle and are essentially used to modify response and request objects.
Middleware functions may also be used to execute some code, call the next middleware or end the request and response cycle if there is no next middleware.
A simple Express application often involves more than one middleware function. There are five types of middleware functions that we can have in express applications which are:
- Application-level middleware
- Router-level middleware
- Error-handling middleware
- Built-in middleware
- Third-party middleware
We will create two middleware
functions and learn how to use the next()
function to pass. We will also use the app.use()
function to mount a middleware function.
If a middleware
function is attached to a specific path, it will be invoked only when a request has been made to that particular path. This function accepts the following parameters:
- The path to which the
middleware
function is being mounted. - A
call-back
function contains the request and response object and the next parameter to pass control to the nextmiddleware
function. - We will begin by installing express js in our function and requiring it in our project file using the command below.
npm install express
const express = require('express');
const app = express();
const port = 8080;
app.use((req, res, next) => {
console.log("First middleware function !");
return next();
});
app.use("/tonyloi", (req, res, next) => {
console.log("Second middleware function!");
return next();
});
app.listen(8080, () =>{
console.log(`The server is running on port: ${port}` );
});
Output:
The server is running on port: 8080
First middleware function !
First middleware function !
Second middleware function!
In the above example, the first middleware
function is executed for every request made to the simple Express server that we have created above.
Using the next()
function, it then passes control to the next middleware, and when a request is made through the URL http://localhost:8080/tonyloi
, the second middleware is also executed.
Please note that since the first middleware is not bound to any path, any request made to the server will trigger its execution.
On the other hand, failure to use the next()
function in the first middleware leaves that middleware function hanging and therefore does not pass control to the next middleware function.
This is demonstrated in the example below, where we have deliberately bound both middleware functions to the same path.
Regardless, the first middleware function can still pass control to the next middleware function, as shown below.
const express = require('express');
const app = express();
const port = 8080;
app.use("/tonyloi", (req, res, next) => {
console.log("First middleware function !");
});
app.use("/tonyloi", (req, res, next) => {
console.log("Second middleware function!");
});
app.listen(8080, () =>{
console.log(`The server is running on port: ${port}` );
});
Output:
The server is running on port: 8080
First middleware function !
Use the next()
Function to Pass Control to the Next Middleware
Function in ExpressJS
As shown below, we can pass control to the next middleware when the next()
function is included.
const express = require('express');
const app = express();
const port = 8080;
app.use("/tonyloi", (req, res, next) => {
console.log("First middleware function !");
//pass control to the next middleware
return next();
});
app.use("/tonyloi", (req, res, next) => {
console.log("Second middleware function!");
});
app.listen(8080, () =>{
console.log(`The server is running on port: ${port}` );
});
Output:
The server is running on port: 8080
First middleware function !
Second middleware function!
The Middleware
functions are also an important part of the error handling in Express js. However, Express has a default error handler that handles errors inside route handlers and middlewares.
We still need to handle errors that occur asynchronously. This is where the next()
function is really useful.
The next()
function instructs Express to move on to the next middleware function when used without arguments.
Use the next(err)
Function to Handle Errors in a Route Using ExpressJS
However, when used with a parameter such as the next(err)
function triggers the middleware function responsible for handling errors for that particular route.
const express = require('express');
const app = express();
const port = 8080;
app.get('/users', function(req, res){
var err = new Error("Assume something went wrong in your code !");
next(err);
});
app.use(function(err, req, res, next) {
res.status(500);
res.send("Something is wrong in your code !");
});
app.listen(8080, () =>{
console.log(`The server is running on port: ${port}` );
});
Output:
Something is wrong in your code !
Therefore, the next(err)
function can also handle errors, as shown above. This function skips all other middleware inline and instead executes the error handling middleware when an error has been detected.
We should also note that error handling middlewares should always contain four parameters, as we have done above.
Furthermore, we should also specify that the next object failure to do this middleware will be regarded as regular middleware and will not handle errors.
Finally, we can also use the next('route')
function to skip the subsequent middleware functions on the current route. This function acts like an exit function that we can use to jump to the next matching route.
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedIn