HTTP Proxy Server in NodeJS
- Create a Simple HTTP Proxy in NodeJS Using the HTTP-Proxy Middleware
-
Use the
required()
Method to Import Our Dependencies Into Our Main File in NodeJS - Use the Proxy Server to Rewrite the Path Using the Proxy Server in NodeJS
-
Use the
curl
Tool to Test Proxy Server and Endpoints in NodeJS
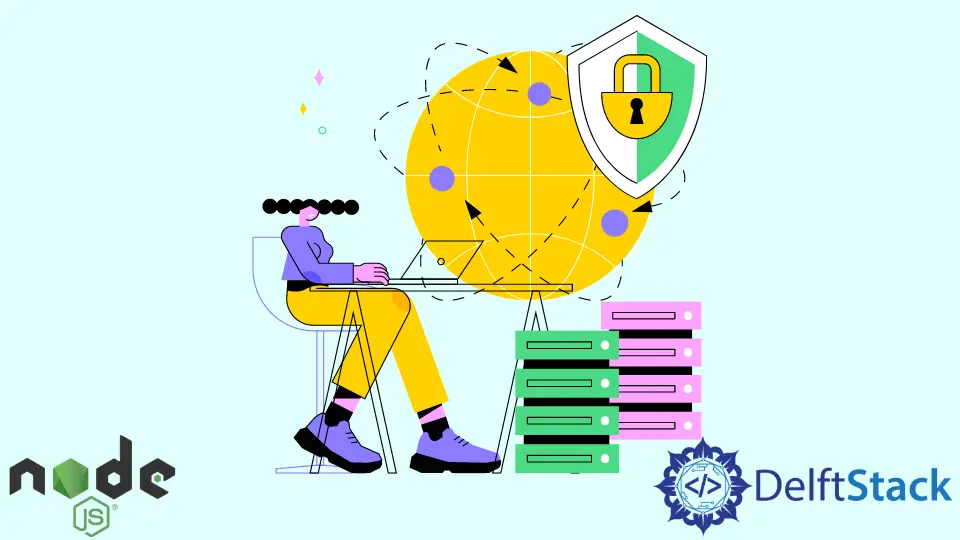
A proxy server is an intermediary server that sits between the server providing the resource and the client.
Proxy servers may be used to unmodified broker transactions between the server and the client at the very basic.
These servers are gateways or tunneling proxies and are generally classified as forwarding.
Also, used as anonymous proxies, which allow the user to browse anonymously. We also have a reverse proxy server, a type of proxy server that provides additional services.
The purpose of these servers is to increase performance, provide security, and increase reliability by offering services such as load balancing.
Reverse proxies can also provide additional security to users by shielding them against attacks such as DDoS attacks, performing SSL encryptions on behalf of the main server, and cache content.
Create a Simple HTTP Proxy in NodeJS Using the HTTP-Proxy Middleware
His article will look at how we can create a simple HTTP proxy in NodeJS using the HTTP-proxy middleware. First, we need to create a new NodeJS project and initialize it.
npm init
Remember that you have to have NodeJS and npm installed on your system for this to work.
This command will allow us to generate a package.json
file that will contain the dependencies used in the program and the author name, and the license.
Once we have set this basic program in place, we can install express js and the http-proxy middleware. Express js is a minimalist web framework that we can use without obscuring the features of NodeJS.
Http-proxy is a one-liner node.js proxy middleware for connecting, expressing, and browser-sync. We can install them using the command below.
npm install express
npm install npm install --save-dev http-proxy-middleware
Running the command above adds them as dependencies to the package.json file below.
{
"name": "proxyy",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"author": "isaac tonyloi",
"license": "MIT",
"dependencies": {
"express": "^4.17.2"
},
"devDependencies": {
"http-proxy-middleware": "^2.0.2",
"install": "^0.13.0",
"npm": "^8.4.0"
}
}
Use the required()
Method to Import Our Dependencies Into Our Main File in NodeJS
We will first begin by importing our dependencies into our main file, index.js
, using the required()
method illustrated below.
const express = require('express');
const { createProxyMiddleware } = require('http-proxy-middleware');
We will then create a simple express server that listens to port 8080 and a proxy server that allows us to mirror requests to their corresponding path of the target site.
In this example, we will use dummyapi.io as our target site. Dummy API is a fake data sandbox API that allows us to play around with real and fake user data.
Similar to jsonplaceholder
but provides interfaces for both REST data API endpoint and GraphQL APIs and more features.
We can perform various changes to our path using this proxy before sending it to the target server. For example, we can rewrite, remove the base path or add to the path.
Use the Proxy Server to Rewrite the Path Using the Proxy Server in NodeJS
const express = require('express');
const { createProxyMiddleware } = require('http-proxy-middleware');
const app = express();
const PORT = 8080;
const API_SERVICE_URL = "https://dummyapi.io";
app.use('/user_info', createProxyMiddleware({
target: API_SERVICE_URL,
changeOrigin: true,
pathRewrite: {
[`^/user_info`]: '',
},
}));
app.listen(PORT, () => {
console.log(`Proxy server listening at port number:${PORT}`);
});
We can start the server by executing the command node index.js on the terminal.
Output:
[HPM] Proxy created: / -> https://dummyapi.io
[HPM] Proxy rewrite rule created: "^/user_info" ~> ""
Proxy server listening at port number:8080
Now if we send a request to the endpoint localhost:8080/user_info/data/v1/user/60d0fe4f5311236168a109ca
the proxy server will rewrite this path to https://dummyapi.io/data/v1/user/60d0fe4f5311236168a109ca
.
Use the curl
Tool to Test Proxy Server and Endpoints in NodeJS
We can use the curl tool to test these endpoints and see our proxy server work. Please note that we have also added a header containing the app-id to the request, as shown below.
curl -H "app-id: 61fcc16fcc2b30f8f01dfdb5" localhost:8080/user_info/data/v1/user/60d0fe4f5311236168a109ca
{"id":"60d0fe4f5311236168a109ca","title":"ms","firstName":"Sara","lastName":"Andersen","picture":"https://randomuser.me/api/portraits/women/58.jpg","gender":"female","email":"sara.andersen@example.com","dateOfBirth":"1996-04-30T19:26:49.610Z","phone":"92694011","location":{"street":"9614, Søndermarksvej","city":"Kongsvinger","state":"Nordjylland","country":"Denmark","timezone":"-9:00"},"registerDate":"2021-06-21T21:02:07.374Z","updatedDate":"2021-06-21T21:02:07.374Z"}
We have successfully created an http proxy server that redirects and modifies a request to a particular service.
In addition to this, we can also perform authorizations of our request using this proxy server as the authentication middleware.
This ensures that only authorized users have access to a certain service. Below is a simple implementation of real software; we usually have more sophisticated authorizations.
const express = require('express');
const { createProxyMiddleware } = require('http-proxy-middleware');
const app = express();
const PORT = 8080;
const service_endpoint = "https://dummyapi.io";
app.use('', (req, res, next) => {
if (req.headers.authorization) {
next();
} else {
res.sendStatus(403);
}
});
app.use('/user_info', createProxyMiddleware({
target: service_endpoint,
changeOrigin: true,
pathRewrite: {
[`^/user_info`]: '',
},
}));
app.listen(PORT, () => {
console.log(`Proxy server listening at port number:${PORT}`);
});
To test out whether our proxy server authorizes our requests, we can send a request to the endpoint without including authorization information. This will return an error, as shown below.
>curl -H "app-id: 61fcc16fcc2b30f8f01dfdb5" localhost:8080/user_info/data/v1/user/60d0fe4f5311236168a109ca
Output:
Forbidden
This means that our authorization middleware is working, and we need to pass our credentials into the headers to access the service.
curl -H "app-id: 61fcc16fcc2b30f8f01dfdb5" -H "Authorization: isaactonyloi" localhost:8080/user_info/data/v1/user/60d0fe4f5311236168a109ca
{"id":"60d0fe4f5311236168a109ca","title":"ms","firstName":"Sara","lastName":"Andersen","picture":"https://randomuser.me/api/portraits/women/58.jpg","gender":"female","email":"sara.andersen@example.com","dateOfBirth":"1996-04-30T19:26:49.610Z","phone":"92694011","location":{"street":"9614, Søndermarksvej","city":"Kongsvinger","state":"Nordjylland","country":"Denmark","timezone":"-9:00"},"registerDate":"2021-06-21T21:02:07.374Z","updatedDate":"2021-06-21T21:02:07.374Z"}
E:\>curl -H "app-id: 61fcc16fcc2b30f8f01dfdb5" localhost:8080/user_info/data/v1/user/60d0fe4f5311236168a109ca
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedIn