Module.exports in Node JS
- Using Modules in Node JS
- Using Third-Party Modules in Node JS
- Using Local Modules in Node JS
-
Using the
module.experts
in Node JS
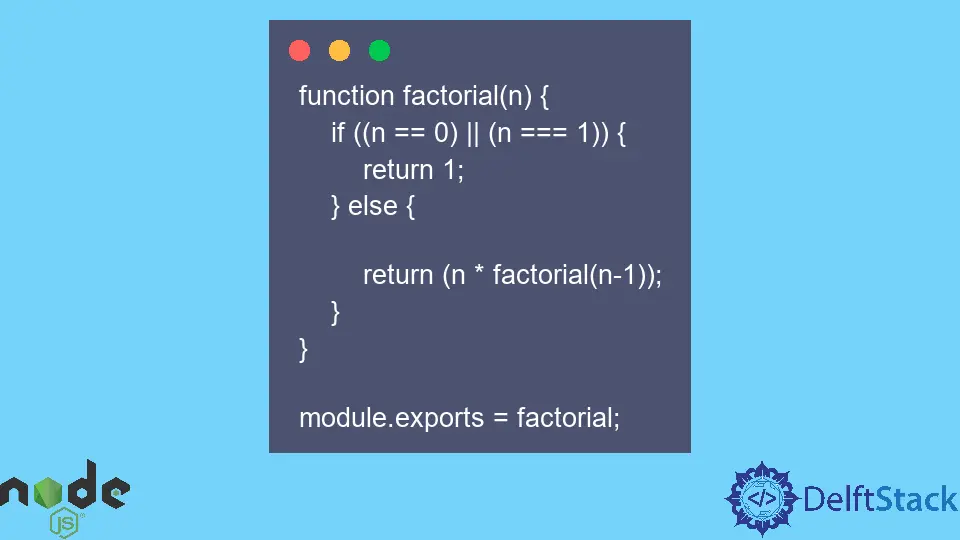
In Node js, module.export
exposes modules on the server-side and as provided for under the CommonJS format.
Node js modules can be referred to as prebuilt bundles of code containing javascript objects and functions and can be used by external applications.
Using Modules in Node JS
Modules are an essential part of writing programs since they make our lives easy as developers by allowing us to reuse code
, debug
, easily understand
and manage complex applications
.
There are three types of modules in Node js; those that intrinsically come with the Node js installation are known as the Core
modules. These modules are easy to use within our programs since we only need to access their functionality.
A typical example of this would be the HTTP
module.
const http = require('http');
Using Third-Party Modules in Node JS
We also have third-party
modules that require installation before using them in our programs. These modules are usually installed using the npm package.
A typical example of such modules is the express package. After the installations, such packages are added as dependencies under the package.json
file.
We can then import them into our programs using the require keyword
.
npm install express
const express = require('express');
Using Local Modules in Node JS
Finally, we also have local
modules. As the name suggests, these are modules that we create by ourselves to use to perform various operations.
Unlike the previous two types of modules, these modules require us first to export them to make them available and then import them into the program where we intend to implement them.
There are two widely used exporting and importing modules on the server-side, especially with Node js. These are the CommonJS and ESM
formats.
The CommonJS format allows us to create modules in unique contexts in different Node js files.
Modules that conform to this format may be referred to as CommonJs
modules, and under this format, we use the module.exports
object to expose a module and the default require
keyword to import them into programs.
The ESM
format, on the other hand, uses the export
keyword to export
modules and the import
keyword to import them according to the ES6
syntax.
Using the module.experts
in Node JS
Using the module.exports
we can export literals
, functions
, classes
, and objects
. The keyword
module represents the current
module, while the keyword export exposes any object as a module.
We can export literally as modules by simply assigning them to module.exports
. It is because exports are themselves an object, and thus any literal assigned to it will be exported as a module.
In the example below, we have created a Node js application with our main file being index.js
and description.js
containing the literal we want to export as modules.
Using the description.js
containing the literals.
let tesla = 'Largest electric car manufacturer';
module.exports = tesla;
Using the index.js
as our main file.
var desc_tesla = require('./description.js');
console.log(desc_tesla);
Output:
Largest electric car manufacturer
In the Node application above, we have exported a String literal
and imported it into index.js
by specifying the path to the root directory where description.js
resides.
We can also export a function as a module by simply creating a function as we would typically do in javascript and then assigning the name of the function to module.exports
.
Now that the module system knows that we want to expose this function as a module, we can access it by simply requiring it in the index.js
file, as shown below.
Using the description.js
containing the literals.
function factorial(n) {
if ((n == 0) || (n === 1)) {
return 1;
} else {
return (n * factorial(n - 1));
}
}
module.exports = factorial;
Using the index.js
as our main file.
var factorial = require('./description.js');
console.log(factorial(7));
Output:
5040
Similarly, we can export objects as modules. An object is a standalone entity with properties and types.
We can assign the object to module.exports
or the object name to module.exports
. Once we have exposed the object, we can access it in our desired location using the require()
method below.
Using the description.js
containing the literals.
const car = {
make: 'Tesla',
model: 'S plaid',
top_speed: '200mph',
year: 2019
}
module.exports = car;
Using the index.js
as our main file.
var car = require('./description.js');
console.log(car.make);
console.log(car.model);
console.log(car.top_speed);
Output:
Tesla
S plaid
200mph
Once we have imported the object into the index.js
, we can access the object’s properties using the dot notation.
In the examples above, we have been importing modules using ./description.js
with ./
specifying that description.js
is located in the root folder.
However, this may not always be the case; in some instances, modules may be located in separate folders. We need to specify the absolute location import such modules starting with ./
pointing to the root folder.
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedIn