How to Generate Unique ID in Node JS
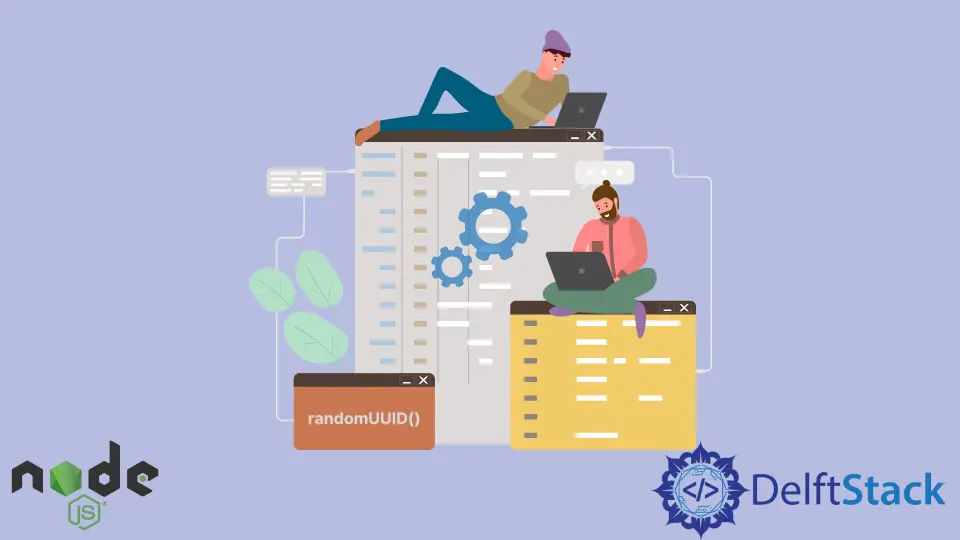
Also known as globally unique identifiers in Microsoft’s version, Universally Unique Identifiers are 128-bit
labels used for creating unique IDs.
These IDs
are commonly used to identify entities in databases to record individual identities uniquely. When used in this context, these IDs
are often referred to as database keys.
How to Generate Unique ID in Node JS
There is almost zero chance that anybody in the world would generate two similar UUIDs
. This is the reason why they are referred to as universally unique.
The Internet Engineering Task Force has come up with a publication known as the RFC that defines a set of standards for creating algorithms that generate these UUIDs
to preserve their uniqueness around the globe.
There are several Node js modules for creating UUIDs in Node JS. However, the most commonly used package is the UUID package.
We can install the UUID package using the npm package installer command below.
npm install uuid
This package can be used with Node js 8, 10, 12, and 14
, but it is also cross-platform, implying that we can use ES6 syntax
or CommonJS
to generate cryptographically substantial random numbers securely.
Now since the UUID package has support for RFC4122 version 1, 3, 4, and 5 UUIDs
, that means we can generate different types of UUIDs as we wish.
Version 1 is a time-based UUID that uses a combination of random numbers, date-time
values, and the device’s MAC address to generate a universally unique ID.
Version 4 UUIDs are perhaps the most simple IDs since they generate from characters a to z and 0 to 9
.
On the other hand, version 3 and 5 UUIDs use preexisting namespace date and name data to generate random alphanumeric UUID.
Using the CommonJS syntax, we can generate version 4 and version 1 of UUIDs, as shown below.
const {v4: uuidv4} = require('uuid');
const {v1: uuidv1} = require('uuid');
console.log(uuidv4());
console.log(uuidv1());
Output:
ddd3b16f-2b6c-4fef-9f21-aaa52d1f983c
69b42bc0-7a15-11ec-a689-5191cd2179b0
Alternatively, we can use ES6 module syntax instead of CommonJS and still generate UUIDs of any version, as shown below.
Remember to set type
: module
in package.json
to use this syntax.
import {v1 as uuidv1, v4 as uuidv4} from 'uuid';
console.log(uuidv4());
console.log(uuidv1());
Output:
9cae2569-010e-4a69-867c-65601234e2a5
0b2567c0-7a17-11ec-9b81-19257147bda6
We can also generate UUIDs using the crypto module added from version 4.17.0
of Node js.
In earlier versions of Node js, there was no way to generate UUIDs natively other than by using external packages such as the UUID package.
Using this module, we can generate up to 128 RFC4122 version 4 UUIDs
using the randomUUID options
method by simply requiring the crypto
module at the top of our program.
By default, Node js will cache enough data to generate up to 128 version 4 UUIDs
using this method. However, we can also generate the UUIDs without caching by setting the object that disables EntropyCache
to true.
const crypto = require('crypto');
console.log(crypto.randomUUID());
Output:
7a2e09e9-4059-438d-89cf-c2badb76ed3c
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedIn