How to Create and Export Classes in ES6 Using Node JS
-
Create
Classes
inES6
Using Node JS -
Use the
constructor()
Method to Create Classes in ES6 Using Node JS -
Use the
module.export
Method to Export Modules in ES6 Using Node JS
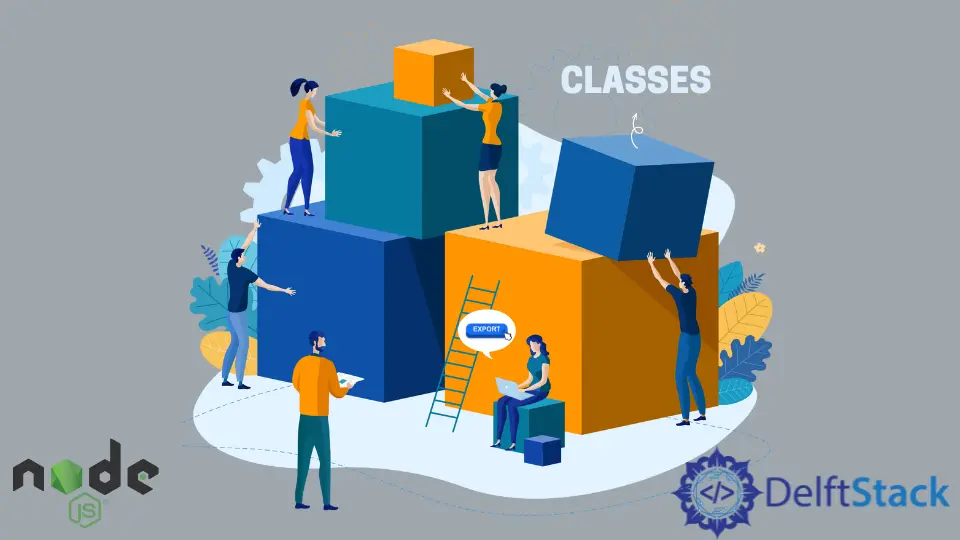
The Classes
are blueprints for creating objects in Javascript. In the new language specification ECMAScript 2015
, classes are created using the keyword class and constructor()
method.
Before these new developments, developers relied on constructor functions to create object-oriented designs. It was often an impediment for new developers trying to learn the language.
In addition to this, while no new concepts were introduced in these new developments, the ability to create classes
like developers do in other languages allows us to have a more elegant syntax.
Besides this, novice developers can easily relate this new syntax to other languages such as java. There are two ways of creating classes in this new ES6
syntax.
Create Classes
in ES6
Using Node JS
You can choose to create class declarations
or class expressions
. This conforms to regular javascript functions with function expressions
and functions declarations
.
Use the constructor()
Method to Create Classes in ES6 Using Node JS
Due to this, classes are often referred to as special functions
. We will use the keyword class and the constructor
method to create a class declaration, as shown below.
class Employee {
constructor(name, age) {
this.name = name;
this.age = age;
}
getName() {
return this.name;
}
}
In the example above, we have created a class named Employee
. We have also initialized the instance’s properties using the constructor()
method.
This method is automatically called when instantiating an object of the class. According to the ES6
syntax, we can only have one constructor method within a class, and classes should always be declared before they are called.
We can create an object that automatically calls the constructor
method using the class name. Using this object, we can also call methods created within the class.
class Employee {
constructor(name, age) {
this.name = name;
this.age = age;
}
getName() {
return this.name;
}
getAge() {
return this.age;
}
}
let obj = new Employee('Isaac Tonyloi', 21);
console.log(obj.getName());
console.log(obj.getAge());
Output:
Isaac Tonyloi
21
Alternatively, we can also define classes as expressions. Although most of the constructs of a class definition remain unchanged, we can choose to have a class expression named
or not named
.
One advantage of class
expressions is that we can pass a class as an argument to a function since classes are first-class citizens.
Here is how we can rewrite the above class declaration as a class expression. In this case, the class expression is not named
.
let Employee = class {
constructor(name, age) {
this.name = name;
this.age = age;
}
getName() {
return this.name;
}
getAge() {
return this.age;
}
}
let obj = new Employee('Isaac Tonyloi', 21);
console.log(obj.getName());
console.log(obj.getAge());
Output:
Isaac Tonyloi
21
In the example above, you will notice that creating an object is the same when creating a class expression and class declaration.
Class expressions cannot be called before they are created, like class declarations. Classes can be imported just like any other module in Node JS.
A common way of working with Python modules is by using the module.export
method to export a module and the require()
method to import it into another module.
Use the module.export
Method to Export Modules in ES6 Using Node JS
The module.export
method is used to export modules on the server-side and provided under the CommonJS
format.
In the example below, we use the class expression we have just created in the previous example as the module we want to export.
We will, however, first create a simple Node JS application with a file containing this class definition and another file where we want to export this class too.
Index.js
let Employee = class {
constructor(name, age) {
this.name = name;
this.age = age;
}
getName() {
return this.name;
}
getAge() {
return this.age;
}
}
module.exports = Employee;
Server.js
let Employee = require('./index.js');
let obj = new Employee('Isaac Tonyloi', 21);
console.log(obj.getName());
console.log(obj.getAge());
In the example above, we have posted our class expression defined in index.js
using the module.exports
method. In the file named server.js
, we have imported the index.js
file as a module using the require()
method.
Now that we have the class definition available in sever.js
, we created an instance for the Employee
and used it to access its methods.
You can also perform other operations, such as extending the class in a different file using the same method.
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedIn