How to Round Down to the Nearest Integer in MySQL
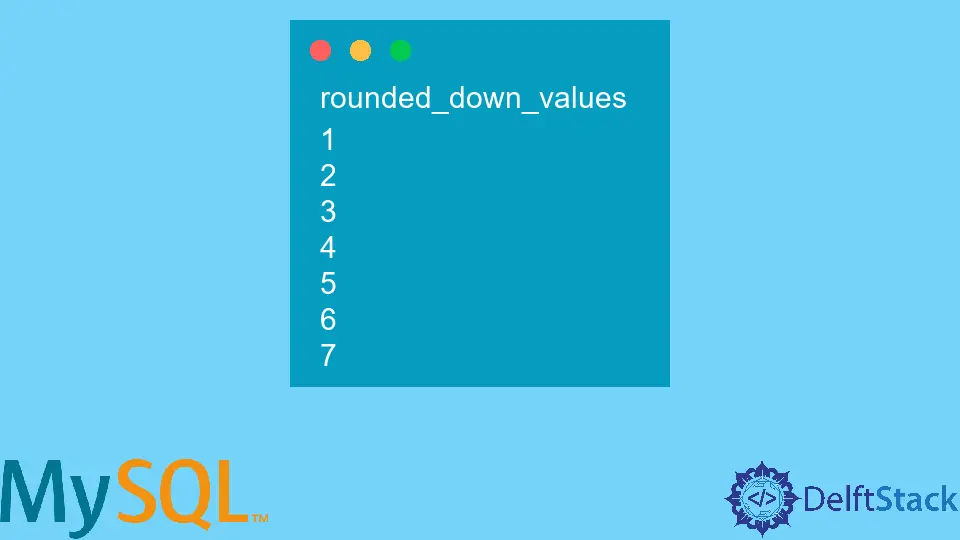
In this tutorial, we aim at exploring how to round down to the nearest integer in MySQL.
Generally, while updating information in a particular database table in MySQL, we need to round down specific floating-point values to the nearest integer. MySQL helps us do this task with the help of the FLOOR()
function.
Let us try to understand more about this function.
The FLOOR()
method in MySQL takes one parameter and gives one integer value as the output. The basic syntax of this function in MySQL is FLOOR(A)
, where A
stands for any integer or floating-point value.
For example, if the A
value is 4.4
, the output of FLOOR(4.4)
would be 4
. And if the value of A
is 3.9
, the output of FLOOR(3.9)
would be 3
.
Thus, we can see that the values are rounded down to the nearest integer. Let us understand how this method works in a particular table.
Before starting, let us create a dummy dataset to work on.
-- create the table student_information
CREATE TABLE student_information(
stu_id float,
stu_firstName varchar(255) DEFAULT NULL,
stu_lastName varchar(255) DEFAULT NULL,
primary key(stu_id)
);
-- insert rows to the table student_information
INSERT INTO student_information(stu_id,stu_firstName,stu_lastName)
VALUES(1.3,"Preet","Sanghavi"),
(2.7,"Rich","John"),
(3.9,"Veron","Brow"),
(4.4,"Geo","Jos"),
(5.3,"Hash","Shah"),
(6.6,"Sachin","Parker"),
(7.0,"David","Miller");
stu_id
supports floating-point values and integers, as the data type for stu_id
is defined as float
.Let us aim at rounding down the stu_id
from the student_information
table.
Round Down in MySQL
The basic syntax to get the round down all the values of a particular table column in MySQL can be illustrated as follows.
SELECT FLOOR(stu_id) as rounded_down_values from student_information;
The code above floors every stu_id
from the student_information
table as no condition is applied. The output of the code is as follows.
rounded_down_values
1
2
3
4
5
6
7
rounded_down_values
with the AS
keyword in the given code in MySQL.Therefore, with the help of the FLOOR()
function, we can efficiently round down non-integer values to the nearest integer in MySQL.