How to Select Single or Multiple Fields for All Documents in a MongoDB Collection
- Select Single or Multiple Fields for All Documents in a MongoDB Collection
- Use the Projection Method to Select Single or Multiple Fields for All Documents in a MongoDB Collection
- Conclusion
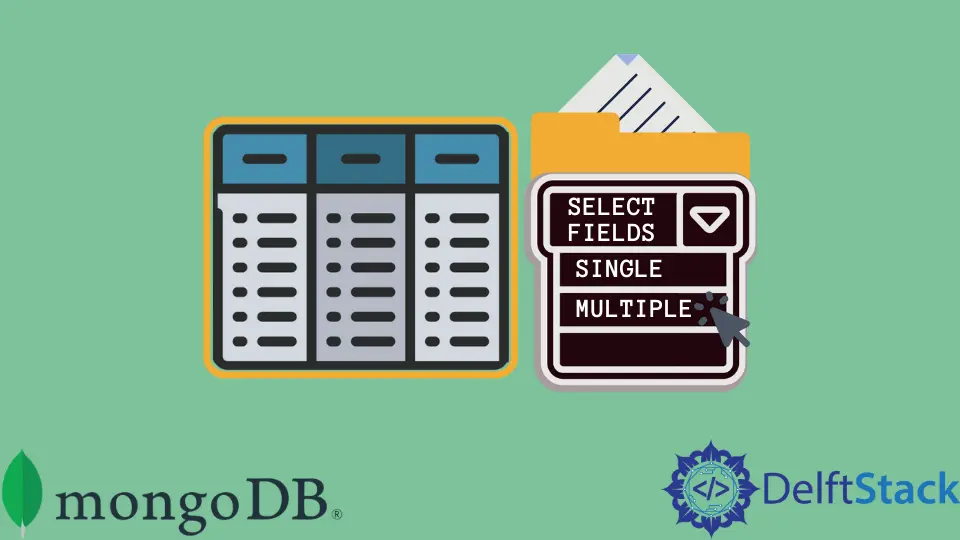
Data is produced at an unprecedented pace now following the global spread of the internet. Since it will require you to collect or request the necessary data from the database to conduct analysis, you must choose the right tool to query the data.
In this article, you will learn to select single or multiple fields for all documents in a MongoDB collection by performing basic query operations through projection.
Select Single or Multiple Fields for All Documents in a MongoDB Collection
MongoDB is an unstructured database management system that stores data in the form of documents. In addition, MongoDB is also very successful at processing large volumes of data.
It is the most widely used NoSQL database because it offers a comprehensive query language and universal and easy data access. For example, to limit the amount of data MongoDB sends to applications, you can include a projection document to specify or restrict fields to return.
A projection can explicitly include several fields. In the following examples used in this article, the find()
method returns all documents that match the query.
The syntax for the projection method is given below:
db.collection.find( { <someOtherArray>: <condition> ... },
{ "<array>.$" : 1 } )
The arrays used in the query and projection documents must be the same length to ensure the intended behavior. If the arrays are different lengths, the operation may error in specific scenarios.
Create a Sample Database
Before starting, you will create a sample Database with some sample data to perform all operations. A collection named teams
was made, and the following records were inserted inside the teams
collection.
This collection will be used for all the examples in this MongoDB tutorial article. The collection is given below:
db={
"teams": [
{
team: "Manchester City ",
position: "1st",
points: 70
},
{
team: "Liverpool",
position: "2nd",
points: 69
},
{
team: "Chelsea",
position: "3rd",
points: 59
},
{
team: "Arsenal",
position: "4th",
points: 54
},
{
team: "Tottenham",
position: "5th",
points: 51
},
{
team: "Manchester United",
position: "6th",
points: 50
}
]
}
Query Documents Using the find()
Method
You will need to use the find()
method to query documents from MongoDB collections. For example, the following statement will retrieve all documents from the collection.
Let’s assume you have a collection with a lot of data in a single document. You have the teams
collection, and you will select just a few fields you want.
Query:
db.teams.find({});
Output:
Use the Projection Method to Select Single or Multiple Fields for All Documents in a MongoDB Collection
A projection can explicitly include several fields. The find()
method will return all the pages that match the query in the following operation.
If you want to retrieve only selected fields from a collection, you can use the projection method.
To do that, you need first to understand the MongoDB query syntax given below:
db.collections.find(
// basic filtering based on the passed-in key values
{},
// selecting the fields
{});
The second parameter can be passed to the find()
method. It will also work with the findOne()
method.
Now let’s select the team
field. You will need to learn one more trick here. The key will be the field you want to choose and its value.
The value will be 1
for selecting a field; 0
for deselecting a field.
Now you can look at some examples given below for a better understanding.
Select Only the Team
Name Field
The following query given below will be used for this example:
db.teams.find({},
{
team: 1
})
Output:
Here we have successfully selected only the team
field. But there is also the _id
field included without you passing it explicitly.
But by default, the mongo query will always have the _id
field.
Deselect the _id
Field
In the following example, you can try to deselect the _id
field itself.
The query for this is given below:
db.teams.find({},
{
team: 1,
_id: 0
})
Output:
Select Only the Team
Name and Points
Field
The following statement will fetch only the team
name and the points
field.
The following query given below will be used for this example:
db.teams.find({},
{
team: 1,
points: 1,
_id: 0
})
Output:
Conclusion
So after going through this MongoDB tutorial, you learned how to select single or multiple fields for all documents in a MongoDB collection by performing basic query operations through projection which specifies or restricts fields to return.