How to Insert Records if Not Exists in MongoDB
-
Upsert
in MongoDB -
Upsert
With thefindAndModify()
Method in MongoDB -
Upsert
With theupdate()
Method in MongoDB -
Upsert
With Operator Expressions in MongoDB -
Upsert
With Replacement Document in MongoDB -
Upsert
With Aggregation Pipeline in MongoDB -
Upsert
With Dotted_id
Query in MongoDB
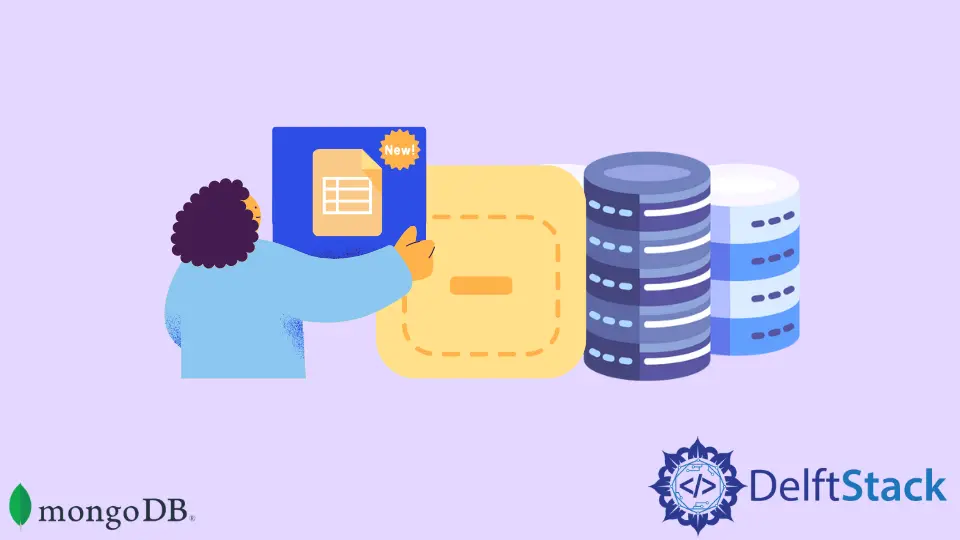
In this article, inserting records in MongoDB collections is discussed briefly. Different ways to insert these records are also explained.
In addition, Upsert
and $setOninsert
are briefly covered.
Upsert
in MongoDB
Upsert
is a MongoDB option that is used for update operations like update()
, findAndModify()
, and so on. Or, to put it another way, upsert
is the result of combining update and insert (update + insert = upsert
).
If the value of the option is true
and the document or documents that match the specified query are identified, the update action will update the matched document or documents. Alternatively, suppose the value of this option is true
, and no document or documents match the provided document.
In that case, this option creates a new document in the collection with the fields specified in operation. The upsert
operation option value is false
by default.
If the upsert
value in a shared collection is true
, you must include the full shared key in the filter.
Syntax:
upsert: <boolean>
The upsert
option value is either true
or false
.
Now you will learn the usage of the upsert
option.
Upsert
With the findAndModify()
Method in MongoDB
With the findAndModify()
function, you may utilize the upsert
option. This option’s default value in this method is false
.
If you set this option’s value to true
, the procedure will conduct one of the following operations.
- If a document or the documents found to match the given query criteria, the
findAndModify()
method updates the document/documents. - If no document/documents match the given query criteria, then the
findAndModify()
method inserts a new document in the collection.
Syntax:
db.Collection_name.findAndModify(
{
selection_criteria:<document>,
sort: <document>,
remove: <boolean>,
update: <document>,
new: <boolean>,
fields: <document>,
upsert: <boolean>,
bypassDocumentValidation: <boolean>,
writeConcern: <document>,
collation: <document>,
arrayFilters: [ <filterdocument1>, … ]
})
By adjusting the value of the upsert
option to true
, you will now insert a new document into the employee
collection.
db.employee.findAndModify({query:{name:"Ram"},
update:{$set:{department:"Development"}},
upsert:true})
Here the value of the upsert
option is set to true
; the findAndModify()
method inserts a new document with two fields (name: "Ram"
and department: "Development"
) because no document matches the name, Ram
.
Upsert
With the update()
Method in MongoDB
With the update()
function, you may utilize the upsert
option. The default value of this parameter in this function is false
.
If you set the value of this option to true
, the procedure will do one of the following.
- If a document or documents are found that match the given query criteria, then the
update()
method updates the document/documents. - If no documents meet the specified query criteria, the
update()
function adds a new document to the collection.
Make a unique index on the name field to prevent MongoDB from adding the same document more than once. For example, if many documents demand the same update with upsert: true
, only one update
action successfully inserts a new document with a unique index.
Syntax:
db.Collection_name.update({Sele ction_ criteria}, {$set:{Update_data}}, {
upsert: <boo. lean >,
multi: <boo. lean>,
writeConcern: < document>,
collation: < document>,
arrayFilters: [ <filter document1>, … ],
hint: <document|string>
})
By changing the value of the upsert
option to true
, you will insert a new document into the employee
collection.
db.employee.update({name:"Priya"}, {$set: {department: "HR"}},{upsert:true})
Because the value for the upsert
option is set to true
, the update()
function inserts a new document with two fields (name: "Priya"
and department: "HR"
) because no document matches the name Priya
.
Upsert
With Operator Expressions in MongoDB
Suppose no document from the given collection matches the filter. In that case, the update
parameter is a document with update
operators.
The value of the upsert
option is true
; the update
operation creates new documents based on the equality clauses from the given query
parameter and applies the expressions from the update
parameter.
Or, to put it another way, when the upsert
option is true
and no document fits the supplied filter, the update
operation creates a new document in the given collection, with the fields specified in the query
and update
documents.
Example:
By changing the value of the upsert
option to true
, you will put a new document into the example
collection.
db.example.update({Name: "Rekha"}, // Query parameter
{$set: {Phone: '7842235468 '}, // Update document
$setOnInsert: {Gender: 'Female'}},
{upsert: true})
The update()
function produces a new document with the query condition’s field "Name: Rekha"
and applies the $set
and $setOnInsert
actions.
Upsert
With Replacement Document in MongoDB
Suppose no document from the supplied collection meets the filter and the update
parameter includes a replacement document, and the value of the upsert
document is set to true
. The update
action inserts a new document in the collection, with the fields specified in the replacement document.
MongoDB does not generate a unique _id
field for the new document if the replacement document includes an _id
field. Alternatively, if the replacement document lacks an _id
field, MongoDB creates a new _id
field for the new document.
Note that distinct _id
field values in the query parameter and replacement document are not permitted. If you do, you will encounter problems.
Example:
By adjusting the value of the upsert
option to true
, you will now insert a new document into the example
collection.
db.example.update({Name:"Hema"}, // Query parameter
{Name:"Hema", Phone:8332564578}, // Replacement document
{upsert:true})
Upsert
With Aggregation Pipeline in MongoDB
The aggregation pipeline is a multi-stage pipeline in which documents are accepted as input and produced as a resultant collection of documents.
The resulting documents are then taken as input and created in the following step (if available) until the last stage. The number of stages in the pipeline might range from one
to n
.
Suppose no document fits the specified filter and the update
parameter includes an aggregation pipeline, and the value of the upsert
option is set to true
. In that case, the update
operation will insert a new document into the collection.
This new document is formed using the equality clause in the query
parameter, and then the pipeline is applied to it to create the document to insert.
Example:
By adjusting the value of the upsert
option to true
, you will now insert a new document into the employee
collection.
db.employee.update({name:"Ram"}, [{$set: {department: "HR", age:31}}],{upsert:true})
Upsert
With Dotted _id
Query in MongoDB
You’ve seen how the update()
function may change data in a collection depending on a query and how the upsert
option can add a new field if no matching documents are found.
However, upsert
with a dotted _id
query is an exception, and attempting to insert a document in this manner would result in an error from MongoDB.
Illustration:
Take a look at the following updating operation. The update will fail while creating the document to insert since the update
operation specifies upsert: true
, and the query provides conditions on the _id
field using dot notation.
db.employee.update({"_id.name":"Roma", "_id.uid":0},{age:21}, {upsert:true})
So, this article discussed the problem of inserting records in empty fields in MongoDB. Upsert
is briefly explained with different scenarios.