How to Store Date and Time in MongoDB
-
Use the
Date()
Method to Store Date and Time in MongoDB -
Use
ISODate()
to Store Date and Time in MongoDB - Conclusion
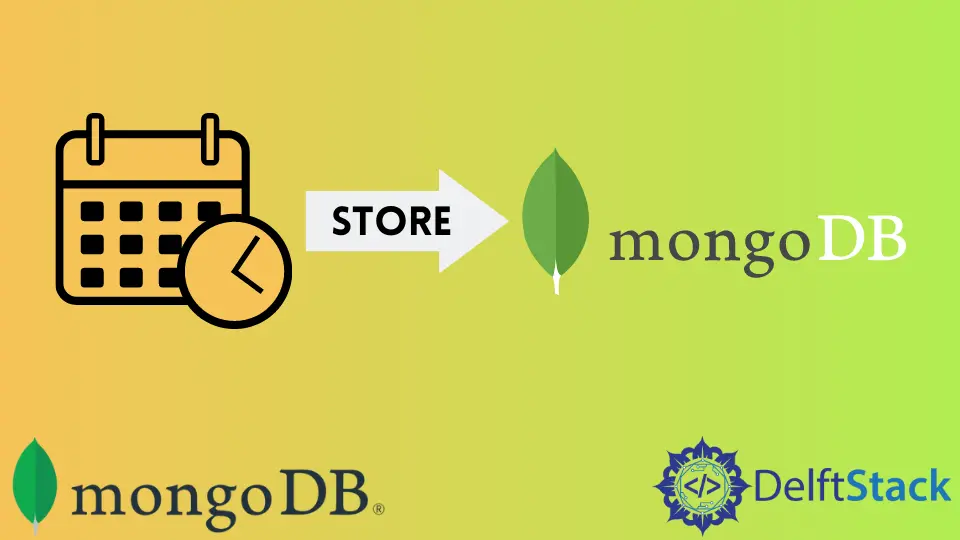
This article will discuss the best way to store the date and time. This will explain the Date()
method in detail.
Moreover, different approaches to using date queries will be described. The date query problem with ISODate not working in MongoDB will also be discussed with an explanation of code segments.
Use the Date()
Method to Store Date and Time in MongoDB
Date()
outputs the current date as a string or a Date
object.
new Date()
returns a Date
object with the current date. The ISODate helper is wrapped around this object by mongosh; the ISODate is in UTC (Universal Time).
You can use the new Date()
function Object() { [native code] }
or the ISODate()
method to define a particular date by giving an ISO-8601 date string with a year between 0 and 9999. These functions accept the following formats:
- The ISODate with the supplied date is returned by
new Date("")
. new Date("")
specifies a DateTime in the client’s local time zone and returns ISODate with that DateTime in UTC.new Date(<integer>)
returns ISODate instance with the DateTime specified in milliseconds since the UNIX epoch (Jan 1, 1970).
Behavior of the Date()
Method in MongoDB
Date
objects are internally kept as a signed 64-bit integer that represents the milliseconds since the Unix epoch.
All database procedures and drivers do not support the complete 64-bit range. Dates with years in the inclusive range 0 through 9999 are safe to deal with.
Examples for the Date()
Method
Use Date()
in a Query
The following code adds a document with the field dateAdded
set to the current date if no document with the _id
equals to 1 exists in the products
collection:
db.products.updateOne(
{ _id: 1 },
{
$set: { item: "apple" },
$setOnInsert: { dateAdded: new Date() }
},
{ upsert: true }
)
Return Date as a String
Use the Date()
method to return the date as a string, as seen in the following example:
var myDateString = Date();
Return Date as Date
Object
The Mongosh wraps objects of date type with the ISODate helper; however, the objects remain of date type.
The following example uses new Date()
to return the Date
object with the specified UTC DateTime.
var myDate = new Date("2016-05-18T16:00:00Z");
MongoDB Sort by Date
You can solve this problem using the sort()
function and the $sort
aggregate in MongoDB. You may sort the data in ascending or descending order with this tool.
Example:
db.posts.find().pretty()
{
"_id" : 1,
"title" : "MongoDB",
"body" : "Hi there",
"date" : "2021-01-01T00:00:00.000Z",
"Country" : "United Kingdom"
}
{
"_id" : 2,
"title" : "MySQL",
"body" : "Hello",
"date" : ISODate("2020-01-01T00:00:00Z"),
"Country" : "United States of America"
}
{
"_id" : 3,
"title" : "SQL",
"body" : "World",
"date" : ISODate("2021-01-01T00:00:00Z"),
"Country" : "New Zealand"
}
Some data are added to the posts collection, and the date field arranges them in ascending order, with earlier dates appearing first.
db.posts.find().sort({ date: 1 }).pretty()
{
"_id" : 1,
"title" : "MongoDB",
"body" : "Hi there",
"date" : "2021-01-01T00:00:00.000Z",
"Country" : "United Kingdom"
}
{
"_id" : 2,
"title" : "MySQL",
"body" : "Hello",
"date" : ISODate("2020-01-01T00:00:00Z"),
"Country" : "United States of America"
}
{
"_id" : 3,
"title" : "SQL",
"body" : "World",
"date" : ISODate("2021-01-01T00:00:00Z"),
"Country" : "New Zealand"
}
Because the first document has a date string rather than a date
object, the date string appears first, even if the date in document 2 is later.
MongoDB Sort by Date Not Working
There might be several reasons why MongoDB does not function, but you must remember a few procedures to sort by date in MongoDB.
- Always remember that you are storing the data in a collection.
- There are two ways to store the
date
field inside the document -Date()
andISODate()
. - When you sort the
date
field using thesort()
method, check which data type you used.
Example:
db.document.insertOne({ "productId" : 1001, "productDeliveryDateTime": new Date() });
{
"acknowledged" : true,
"insertedId" : ObjectId("611c9e39e1fdc428cf238802")
}
The date is added using the Date()
string in this example, and the collection name is the document. You may insert a date in the document using ISODate()
in the same way.
Use ISODate()
to Store Date and Time in MongoDB
Use the $gte
operator and ISODate()
to implement date query with ISODate in MongoDB.
Let’s make a collection using the document to better grasp this. The following is the query to construct a collection with a document:
> db.dateDemo.insertOne({"StudentName":"John","StudentAge":26,"AdmissionDate":new ISODate("2013-06-07")});
{
"acknowledged" : true,
"insertedId" : ObjectId("5c8a65799064dcd4a68b70ea")
}
The find()
function can display all documents in a collection.
Query:
db.dateDemo.find().pretty();
Output:
Date query using ISODate()
:
> db.dateDemo.find({"AdmissionDate":{"$gte": ISODate("2013-06-07T00:00:00Z")}}).pretty();
Output:
Conclusion
This article briefly explained the Date()
method. After which, the problem of sorting collections with the Date()
method is pointed out.
An example explains how the date query works with ISODate in MongoDB. Also, this demonstrated that the best format to store date and time in MongoDB is using the native JavaScript Date()
or ISODate()
format as it internally converts it into a BSON native Date
object.