How to Check Variable Type in MATLAB
-
Check Type of Variable Using the
class()
Function in MATLAB -
Check Type of Variable Using the
whos
Command in MATLAB -
Use the
isa()
Function to Check if a Variable Is of a Specific Type in MATLAB -
Use the
ischar()
Function to Check if a Variable Is of a Specific Type in MATLAB -
Use the
isnumeric()
Function to Check if a Variable Is of a Specific Type in MATLAB - Conclusion
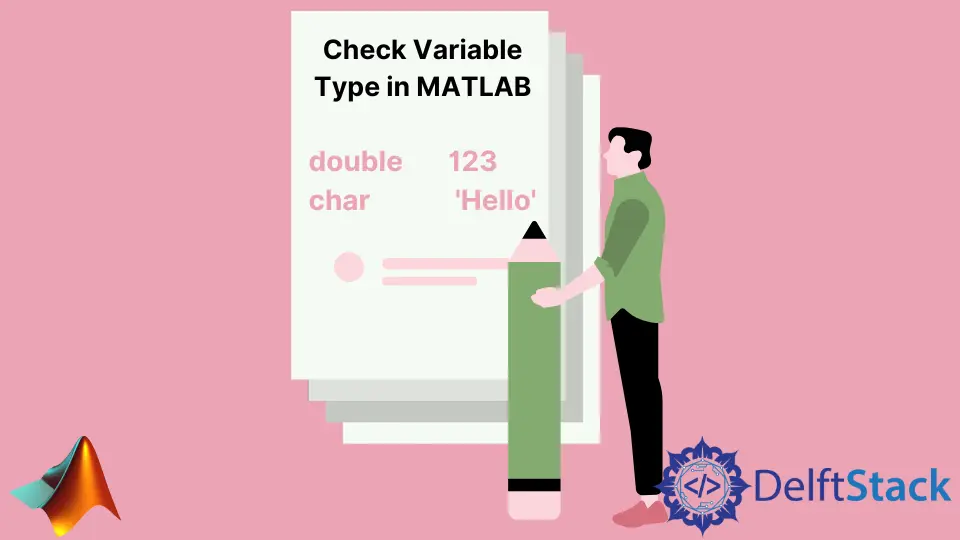
MATLAB, a powerful numerical computing environment, allows users to work with a variety of data types. Understanding the type of variables is essential for effective debugging and ensuring accurate data processing.
In this article, we’ll explore different methods to check the type of a given variable in MATLAB.
Check Type of Variable Using the class()
Function in MATLAB
The class()
function is a valuable tool for determining the type of a variable in MATLAB. This information can be invaluable for debugging and ensuring that your code processes data correctly.
The class()
function in MATLAB is straightforward in its application. Its syntax is as follows:
variableType = class(variable);
Here, variable
is the variable whose type you want to determine, and variableType
is the resulting string representing its class.
Let’s illustrate the use of the class()
function with a practical example:
numericVar = 42;
charVar = 'Hello, MATLAB!';
typeNumeric = class(numericVar);
typeChar = class(charVar);
disp(['Type of numericVar: ', typeNumeric]);
disp(['Type of charVar: ', typeChar]);
In the provided example, we start by defining two variables: numericVar
and charVar
. These variables hold a numeric value (42
) and a character array ('Hello, MATLAB!'
), respectively.
Next, we use the class()
function to determine the types of these variables. The results are stored in the variables typeNumeric
and typeChar
.
Finally, we display the results using the disp()
function, presenting the types of both numericVar
and charVar
. The output will show the class of each variable, providing insights into the dynamic typing nature of MATLAB.
Output:
Type of numericVar: double
Type of charVar: char
Here, MATLAB informs us that numericVar
is of type double
, indicating a numeric variable, while charVar
is of type char
, indicating a character array.
Check Type of Variable Using the whos
Command in MATLAB
In addition to the class()
function, MATLAB provides the whos
command for inspecting variable information, including their types. This command offers a comprehensive overview of all variables in the workspace, making it a useful tool for type-checking.
The whos
command in MATLAB is employed without parentheses and provides detailed information about variables in the workspace. When used for type checking, it displays the name, size, bytes, class, and attributes of each variable.
Let’s dive into a practical example:
numericVar = 42;
charVar = 'Hello, MATLAB!';
whos;
In this example, we start by defining two variables: numericVar
and charVar
. One contains a numeric value (42
), and the other holds a character array ('Hello, MATLAB!'
).
The key part of this example is the use of the whos
command without any arguments. When executed, whos
displays detailed information about all variables in the workspace, including their names, sizes, bytes, classes, and attributes.
This information allows us to identify the type of each variable, providing a comprehensive snapshot of the workspace.
Output:
Variables in the current scope:
Attr Name Size Bytes Class
==== ==== ==== ===== =====
charVar 1x14 14 char
numericVar 1x1 8 double
Total is 15 elements using 22 bytes
In this output, MATLAB presents a table containing the names, sizes, bytes, classes, and attributes of the variables in the workspace. For instance, numericVar
is shown as a variable of class double
, indicating its numeric nature, while charVar
is identified as a variable of class char
, signifying its character array type.
Use the isa()
Function to Check if a Variable Is of a Specific Type in MATLAB
The isa()
function serves as a versatile tool for precisely checking the type of a given variable. Unlike the class()
function and whos
command, isa()
is particularly handy when you want to perform a logical check for a specific type.
The isa()
function in MATLAB has the following syntax:
isOfType = isa(variable, 'typeName');
Here, variable
is the variable whose type we want to check, and 'typeName'
is the name of the type we are checking against. The result is a logical value (1
for true, 0
for false) stored in the variable isOfType
.
Let’s illustrate the use of the isa()
function with a practical example:
numericVar = 42;
charVar = 'Hello, MATLAB!';
isNumeric = isa(numericVar, 'double');
isChar = isa(charVar, 'char');
disp(['Is numericVar a double? ', num2str(isNumeric)]);
disp(['Is charVar a char? ', num2str(isChar)]);
In this example, we define two variables: numericVar
and charVar
. One holds a numeric value (42
), and the other contains a character array ('Hello, MATLAB!'
).
We then utilize the isa()
function to check if each variable is of a specific type. For numericVar
, we check if it is of type double
, and for charVar
, we check if it is of type char
.
The results are stored in the variables isNumeric
and isChar
.
Finally, we use disp()
to output the results. The num2str()
function is used to convert logical values to strings for display purposes.
Output:
Is numericVar a double? 1
Is charVar a char? 1
In this output, MATLAB confirms that numericVar
is of type double
, and charVar
is of type char
. The logical values (1
in both cases) indicate that the variables match the specified types.
This logical output is especially useful when you need to conditionally execute code based on the type of a variable.
Use the ischar()
Function to Check if a Variable Is of a Specific Type in MATLAB
When you have a specific need to check if a variable is of character type in MATLAB, the ischar()
function is a reliable choice. This function returns a logical value, indicating whether the variable is a character array or not.
The ischar()
function in MATLAB follows a straightforward syntax:
isCharacter = ischar(variable);
Here, variable
is the variable whose type we want to check, and isCharacter
is the resulting logical value (1
for true, 0
for false).
Here’s an example to illustrate the use of the ischar()
function:
charVar = 'Hello, MATLAB!';
isCharVar = ischar(charVar);
disp(['Is charVar a char? ', num2str(isCharVar)]);
In this example, we define a variable charVar
that holds the character array 'Hello, MATLAB!'
.
The ischar()
function is then used to check if charVar
is indeed of type char
. The result, a logical value, is stored in the variable isCharVar
.
Finally, we use disp()
to output the result. The num2str()
function is employed to convert the logical value to a string for display purposes.
Output:
Is charVar a char? 1
In this output, MATLAB confirms that charVar
is of type char
by returning a logical value of 1
. This functionality is particularly useful when you need to conditionally execute code based on whether a variable is a character array or not.
Use the isnumeric()
Function to Check if a Variable Is of a Specific Type in MATLAB
Specifically, the isnumeric()
function is a handy tool for verifying whether a given variable is of numeric type. This function simplifies the process of checking if a variable contains numerical data.
The isnumeric()
function in MATLAB follows a simple syntax:
isNumericType = isnumeric(variable);
Here, variable
is the variable whose type we want to check, and isNumericType
is the resulting logical value (1
for true, 0
for false) indicating whether the variable is of numeric type.
Let’s illustrate the use of the isnumeric()
function with a practical example:
numericVar = 42;
charVar = 'Hello, MATLAB!';
isNumericNumericVar = isnumeric(numericVar);
isNumericCharVar = isnumeric(charVar);
disp(['Is numericVar numeric? ', num2str(isNumericNumericVar)]);
disp(['Is charVar numeric? ', num2str(isNumericCharVar)]);
In this example, we have two variables: numericVar
, which contains the numeric value 42
, and charVar
, which holds the character array 'Hello, MATLAB!'
.
We use the isnumeric()
function to check if each variable is of numeric type. The logical results are stored in the variables isNumericNumericVar
and isNumericCharVar
.
The disp()
function is then employed to output the results. The num2str()
function converts the logical values to strings for display purposes.
Output:
Is numericVar numeric? 1
Is charVar numeric? 0
The output confirms that numericVar
is indeed of numeric type (logical value 1
), while charVar
is not of numeric type (logical value 0
).
Conclusion
MATLAB provides a variety of methods to check variable types, from basic functions like class()
to more advanced tools like whos
and logical checks with isa()
.
In addition to the mentioned type-checking functions, MATLAB provides a diverse set of functions tailored for specific data type-checking. For instance, the iscell()
function proves useful for verifying whether a variable is of cell
type, allowing you to discern if the data is organized in a cell array structure.
The isinteger()
function comes in handy when the goal is to determine whether a variable holds integer values, facilitating precision in handling numerical data. If you’re dealing with floating-point numbers, the isfloat()
function becomes a valuable tool, enabling you to confirm whether the variable aligns with the float
type.
MATLAB offers a comprehensive suite of these specialized functions, such as islogical()
for logical arrays, isdatetime()
for date and time values, and isstring()
for string arrays. These functions allow you to perform type checks, ensuring that your code adapts dynamically to diverse data structures and types encountered during execution.