How to Determine Which Characters Are Letters in MATLAB
-
Use the
isletter()
Function to Check Which Characters Are Letters in MATLAB - Use Regular Expression to Check Which Characters Are Letters in MATLAB
- Use ASCII Values to Check Which Characters Are Letters in MATLAB
- Conclusion
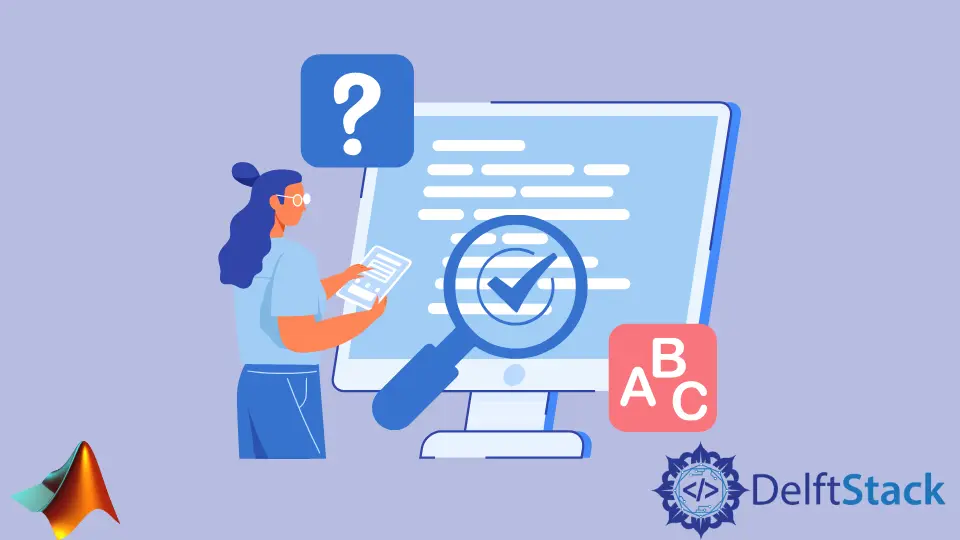
Checking which characters are letters in MATLAB involves the identification of alphabetic characters within a given string or character array.
This tutorial will discuss the ways to determine which characters are letters in Matlab.
Use the isletter()
Function to Check Which Characters Are Letters in MATLAB
The isletter()
function in MATLAB is a built-in function that checks if each element of an input array is a letter (alphabetic character). The function provides a logical array with dimensions identical to those of the input array, where each element is true
if the corresponding element in the input array is a letter and false
otherwise.
Basic Syntax:
isLetterArray = isletter(inputArray);
Parameter:
inputArray
: This is the input array (e.g., a string or a character array) that you want to check for letters.
For example, let’s check a given character if it’s a letter or not.
Example Code 1:
c = '5';
isletter(c)
Output:
ans = 0
In the code above, we have a character '5'
assigned to the variable c
, and we are using the isletter()
function to check if this character is a letter. In this case, the character '5'
is not a letter, and therefore, the isletter()
function returns a logical value of 0
(false).
We can also check if a string or array of characters contains letters or not. If a character inside the string or array of characters is a letter, then the isletter()
function will return 1
in its place and 0
elsewhere.
For example, let’s create a string containing letters as well as numbers and check how many letters are present inside the string.
Example Code 2:
c = '25 street';
isletter(c)
Output:
ans =
0 0 0 1 1 1 1 1 1
In the code, we have a character array '25 street'
assigned to the variable c
, and we are using the isletter()
function to determine which characters in the array are letters. The isletter()
function evaluates each element in the array individually and returns a logical array where each element corresponds to whether the corresponding character is a letter or not.
This output indicates that the character '2'
, 5'
, and the spaces are not letters (denoted by '0'
), while the characters 's'
, 't'
, 'r'
, 'e'
, and 't'
are letters (denoted by '1'
).
Use Regular Expression to Check Which Characters Are Letters in MATLAB
In MATLAB, the regexp
function is used for regular expression matching in strings. Regular expressions (regex
or regexp
) are patterns that describe sets of strings.
The regexp
function searches for these patterns within a given string and returns the starting indices of the matched substrings. We can use regular expressions to check which characters are letters in a string.
Basic Syntax:
indices = regexp(str, pattern);
Parameters:
str
: The input string in which you want to search for the pattern.pattern
: The regular expression pattern to match.
The output indices
is an array containing the starting indices of substrings that match the specified pattern within the input string.
Example Code:
OldString = '25 street';
letterIndices = regexp(OldString, '[a-zA-Z]');
disp(letterIndices);
Output:
4 5 6 7 8 9
In the code, we are working with the string '25 street'
assigned to the variable OldString
. Next, the regexp()
function is employed with the pattern '[a-zA-Z]'
, which signifies a regular expression that matches any lowercase or uppercase letter.
Lastly, the function returns an array containing the indices of the characters that satisfy this pattern. The output indicates that, according to the specified regular expression, the letters in the string '25 street'
are located at positions 4
, 5
, 6
, 7
, 8
, and 9
and these positions correspond to the letters 's'
, 't'
, 'r'
, 'e'
, and 't'
, respectively, in the given string.
Use ASCII Values to Check Which Characters Are Letters in MATLAB
We can use the ASCII values of characters to distinguish between letters and non-letters within the string. The use of ASCII values allows for a numerical comparison to determine whether a character falls within the range associated with letters in the ASCII character set.
Example Code:
OldString = '25 street';
isLetterArray = (OldString >= 'A' & OldString <= 'Z') | (OldString >= 'a' & OldString <= 'z');
disp(isLetterArray);
Output:
0 0 0 1 1 1 1 1 1
In the code, we are examining the string '25 street'
assigned to the variable OldString
. The objective is to discern which characters in the string are letters by comparing their ASCII values.
Through logical indexing with the expression (OldString >= 'A' & OldString <= 'Z') | (OldString >= 'a' & OldString <= 'z')
, each character’s position in OldString
is assessed to ascertain whether it falls within the ASCII range of uppercase or lowercase letters.
The resulting logical array, isLetterArray
, contains '1'
for positions where the characters are letters and 0
where they are not. The output indicates that the characters '2'
, '5'
, and the spaces are not letters (denoted by 0
), while the characters 's'
, 't'
, 'r'
, 'e'
, and 't'
are letters (denoted by 1
).
Conclusion
In this MATLAB tutorial, we explored efficient methods for character analysis to determine which characters are letters. The isletter()
function emerged as a straightforward tool, providing logical arrays to identify alphabetic characters.
We also delved into alternative techniques, such as using regular expressions with the regexp()
function for flexible pattern matching and leveraging ASCII values for numerical comparisons.
By understanding these methods, we gain versatile approaches to character analysis, empowering them to make informed choices based on our specific programming needs.