How to Create Low Pass Filter in MATLAB
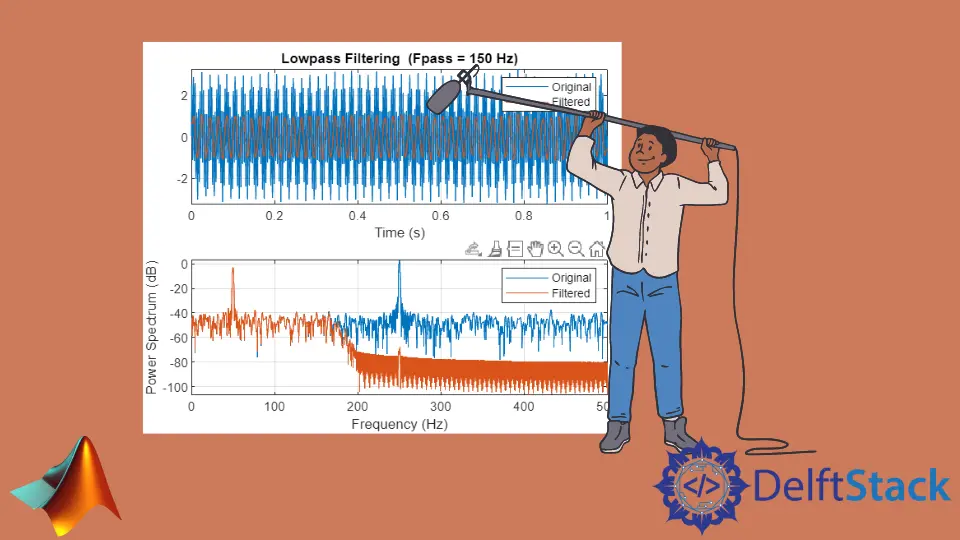
This tutorial will discuss using the lowpass()
function to design and filter a signal in Matlab.
Use the lowpass()
Function to Design and Filter a Signal in MATLAB
A low pass filter is used to filter low-frequency signals from a signal containing multiple frequencies.
For example, if we have a signal which contains two different frequency signals and we want to filter the low-frequency signal. We can do this using a low pass filter which will only allow the low-frequency components from the input signal and block the high-frequency signal.
In MATLAB, we can use the built-in function lowpass()
to filter a signal.
We have to pass the input signal, passband frequency, and the sampling frequency of the input signal in the lowpass()
function. The input signal should be a vector or matrix of type single or double.
The passband frequency should be between 0 to half of the sampling frequency. The sampling rate should be a positive real scalar.
For example, let’s create a signal, add some random noise, and filter it using the lowpass()
function. See the code below.
freqS = 1e3;
time = 0:1/freqS:1;
signal = [1 2]*sin(2*pi*[50 250]'.*time) + randn(size(time))/10;
lowpass(signal,150,freqS)
Output:
The blue signal is the input signal in the output, and the other is the filtered signal.
As you can see in the above figure, the low-frequency signal is filtered. You can also check the power spectrum graph to check the passband frequency at which the signal is filtered.
You can use the above code to filter a high-frequency noise or tone from a musical signal. You can also save the output of the lowpass()
function in a variable, but you have to plot it separately.
If we don’t specify any output, the lowpass()
function will plot the original and filtered signal on the same graph along with their frequency domain plot. We can set other properties of the lowpass()
function like the stopband attenuation, the lowpass filter steepness, and the impulse response type of the filter.
We can also filter data from a timetable using the lowpass()
function. The lowpass()
function filters all variables in the timetable and all columns inside each variable.
By default, the lowpass()
function will use any impulse response. Still, we can change it to finite impulse response by passing the string fir or infinite impulse response by passing the string iir
in the lowpass()
function using the property ImpulseResponse
.
The default steepness value is 0.85, but we can set it to any value in the interval 0.5 to 1 using the property steepness
. The default stopband attenuation is 60, but we can change any positive scalar in dB using the property StopbandAttenuation
.
To change a property, we have to pass its value by its name like lowpass(....,'StopbandAttenuation', 60)
.