How to Export Data From MATLAB to Excel
-
Export Data to Excel File Using the
writematrix()
Function in MATLAB -
Export Data to Excel File Using the
writecell()
Function in MATLAB -
Export Data to Excel File Using the
writetable()
Function in MATLAB
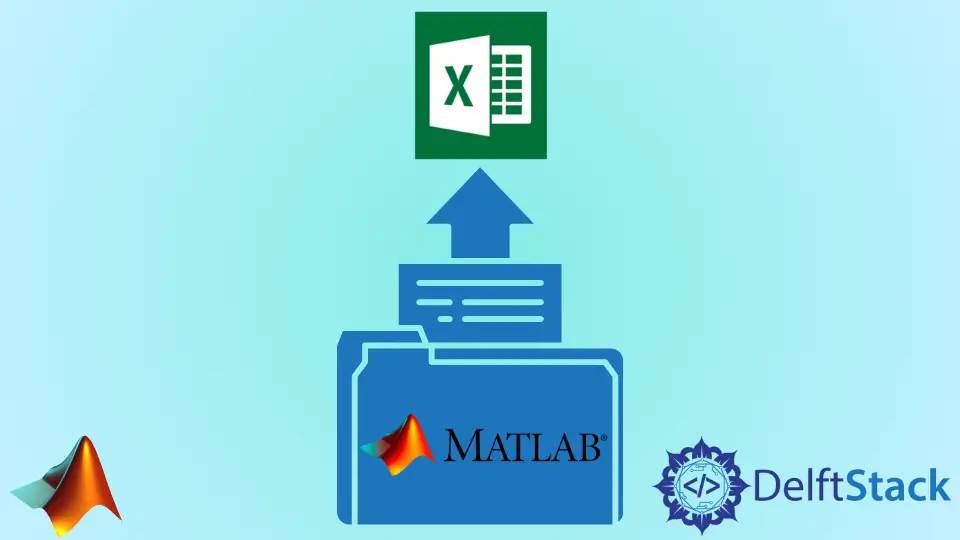
This tutorial will discuss exporting data to an excel file using the writematrix()
, writetable()
, and writecell()
function in MATLAB.
Export Data to Excel File Using the writematrix()
Function in MATLAB
The writematrix()
function is used to write a matrix to a file. If the data we want to export is saved in a matrix, we can use the writematrix()
function.
The writematrix()
function has three syntaxes shown below:
writematrix(data)
writematrix(data,file_name)
writematrix(___,Property_Name,Property_Value)
The first syntax will write the given matrix to a text file with the .txt
extension and use a comma as the delimiter to separate the elements in a row. The writematrix()
function will use the name of the matrix variable as the name of the output file, and if the function cannot use the matrix name, it will use the matrix.txt
name as the name of the file.
Each input matrix row will become a row in the output file. If the file name already exists, the function will overwrite the data, meaning the previous data will be lost and replaced with the new data.
The second syntax is used to set the file name and its extension. We can give any name to the output file.
We can use the .txt
, .dat
, and .csv
file extensions to save data in a text file where we can add delimiters. We can use the .xls
, .xlsm
, and .xlsx
file extension to save data in spreadsheets of an Excel file.
We can use the .xlsb
file extension to save data in an Excel spreadsheet only if the system supports the file format. For example, let us write a matrix to a spreadsheet file.
See the code below.
clc
clear
X = magic(3)
writematrix(X,'X.xls')
The last syntax changes the properties of the writematrix()
function using the property name and value.
We can use the FileType
property to set the file type to text or spreadsheet. We can use the DateLocale
property to set the locales used to write dates.
The DateLocale
property is useful when the input matrix contains DateTime values, and we can use it to specify the format of the date in which it will be stored in the file. Check this link to see all the formats supported by the locale that we can use to store the date values.
We can use the WriteMode
property to set the data writing mode. In the case of text files, we can change the write mode from overwrite
, the default mode, to append
, which will append the new data to the previously stored data in the file.
In the case of excel files, the default mode is set to inplace
, which will replace the data in the new data range. For example, if the new data have five rows and 5 columns, then only the 5 rows and 5 columns of the file will be replaced, and the rest of the data will remain the same.
We can use overwritesheet
mode, which will clear the specified sheet and write new data to the sheet, and if we don’t specify the sheet, the first sheet will be used. We can use the append
mode, which will append the new data at the end of the specified sheet, and if we don’t specify the sheet number, the first sheet will be used.
We can use the replacefile
mode, which deletes all the previous sheets and writes new data to the specified sheet, and if we do not specify a sheet, the first sheet will be used. We can use the Delimiter
property to set the delimiter, which is set to a comma to space
for the space delimiter, tab
for the tab delimiter, ;
for the semicolon, and |
for the vertical bar.
We can use the QuoteStrings
property to set the indicator used to write quoted text in the file. The value of this property can be minimal
, all
, and none
.
The minimal
value is the default value and will enclose the variables containing the double quotation marks, delimiters, and line endings with double quotation marks. The all
value will enclose all date, categorical, and text data with double quotes.
The none
value will not enclose any variable. We can use the Encoding
property to change the scheme used to encode characters, which is set to UTF-8
by default.
We can set the Encoding
property to ISO-8859-1
, windows-1251
, and windows-1252
. We can use the Sheet
property to specify the sheet number or name we want to use to write data, and it can be a character vector or positive integer.
We can use the Range
property to set the range of the spreadsheets we want to use to write new data. The range can be a single sheet that will specify the starting point, and it can also be a range like a form sheet 5 to sheet 10.
We can use the UseExcel
property to set the starting of Excel software when we write data to an Excel file. By default, the property is set to false, which means the Excel software will not start, but we can also set it to true, which will start the Excel software.
For example, let’s repeat the above example and change some properties mentioned above. See the code below.
clc
clear
X = magic(3)
writematrix(X,'X.xls','Sheet',3)
m = readmatrix('X.xls','Sheet',3)
Output:
X =
8 1 6
3 5 7
4 9 2
m =
8 1 6
3 5 7
4 9 2
In the above code, X
is the input matrix, and m
is the matrix saved in the Excel file. We can also set other properties in the same way we change the properties in the above code.
Check this link for more details about the writematrix()
function.
Export Data to Excel File Using the writecell()
Function in MATLAB
The writecell()
function is used to write a cell to a file. If the data we want to export is saved in a cell, we can use the writecell()
function.
writecell()
is the same as the writematrix()
function. The only difference is that the input of the writecell()
function is a cell, and the input of the writematrix()
function is a matrix.
The properties of these functions that we can change are also the same.
For example, let’s create a cell and write it to an excel file. See the code below.
clc
clear
X = {1,2,3}
writecell(X,'Y.xls')
c = readcell('Y.xls')
Output:
X =
1×3 cell array
{[1]} {[2]} {[3]}
c =
1×3 cell array
{[1]} {[2]} {[3]}
In the above output, X
is the input cell, and c
is the cell we imported from the saved file. Check this link for more details about the writecell()
function.
Export Data to Excel File Using the writetable()
Function in MATLAB
The writetable()
function is used to write a table to a file. If the data we want to export is saved in a table, we can use the writetable()
function.
The writetable()
function is also the same as the writematrix()
function; the only difference is that the input of the writetable()
function is a table, and the input of the writematrix()
function is a matrix. The writetable()
function has some additional properties that we can change.
We can use the WriteRowNames
property to set the indicator for writing the names of the rows in the output file.
By default, the WriteRowNames
property is set to false, meaning the table’s row names will not be included in the output file, but we can set it to true if we want to write row names to the output file. We can use the WriteVariableNames
to set the indicator used to write the name of the column headings of the table to the output file.
By default, the WriteVariableNames
property is set to false, which means the table’s column headings will not be included in the output file, but we can set it to true if we want to write column headings to the output file. For example, let’s create a table and write it into an Excel file.
See the code below.
clc
clear
Z = table([1;5],[10;15])
writetable(Z,'Z.xls')
m = readtable('Z.xls')
Output:
Z =
2×2 table
Var1 Var2
____ ____
1 10
5 15
m =
2×2 table
Var1 Var2
____ ____
1 10
5 15
In the above output, Z
is the input table, and m
is the table we imported from the saved file. Check this link for more details about the writetable()
function.