How to Check CPU Time in MATLAB
-
Use the
cputime
Command to Check the CPU Time in MATLAB -
Use the
tic
andtoc
Commands to Check the CPU Time in MATLAB
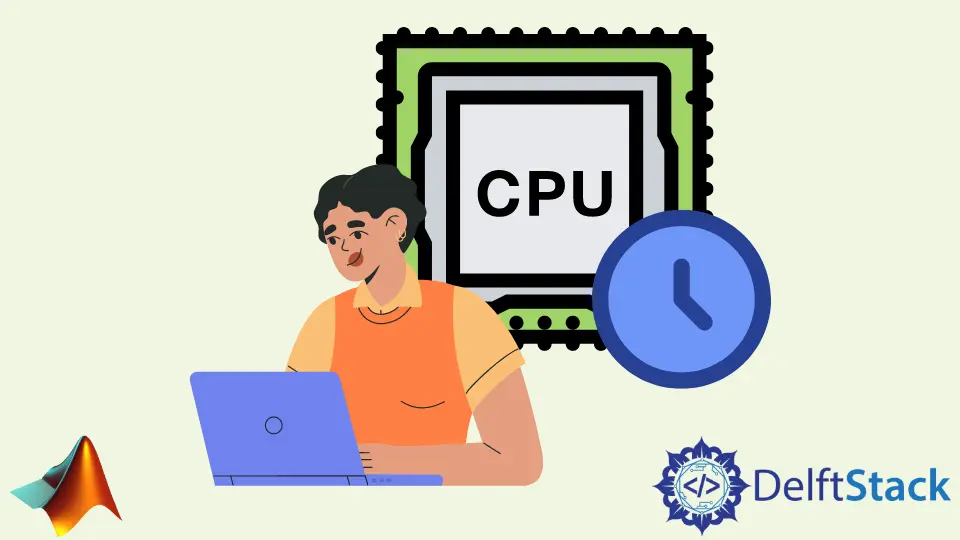
This tutorial will discuss checking the CPU time using the tic
, toc
, and cputime
commands in MATLAB.
Use the cputime
Command to Check the CPU Time in MATLAB
We can use the cputime
command to check the CPU time taken by Matlab in running a specific task. We can note the CPU time before and after the execution of a task, and their difference will be equal to the CPU time taken by that specific task.
For example, let’s find the CPU time taken to create a surface plot in Matlab. See the code below.
clc
clear
Start_t = cputime;
surf(peaks(50))
End_t = cputime;
Elapsed_time = End_t - Start_t
Output:
Elapsed_time =
0.3906
In the above code, the clc
and clear
commands are used to clear the command and workspace window of Matlab. The output is in seconds, which means the task took 390 milliseconds to complete the surface plot.
Note that the output can change depending on the CPU the Matlab is using. If we run the code repeatedly, the output might change, so it is good to run the program several times and take the average of all the periods to minimize the error.
The cputime
command returns the time since Matlab has started running in seconds. The accuracy of finding the time taken by a specific task is less if we use the cputime
command because every time we run the code again, the time will change dramatically, so we should use the tic
and toc
commands explained below.
Use the tic
and toc
Commands to Check the CPU Time in MATLAB
We can use the tic
and toc
commands to check the CPU time taken by Matlab in running a specific task. To check the CPU time, we must define the tic
command before the task and the toc
command after the task.
The tic
command will save the current time, and the toc
command will use the previously stored time to calculate the elapsed time. For example, let’s find the CPU time taken to create a surface plot in Matlab.
See the code below.
clc
clear
tic
surf(peaks(50))
toc
Output:
Elapsed time is 0.105429 seconds.
In the above output, we can see that the time taken by the surface plot is different from the time we found with the cputime
command. We can run an experiment on both methods above to check which function is accurate.
We can use the pause()
function to give a pause in the program and then check the time of the task, which should be close to the pause time. See the code below.
clc
clear
tic
pause(1)
toc
Output:
Elapsed time is 1.010429 seconds.
In the above code, we have given a pause of 1 second, and the output is close to 1 second. Now let’s use the cputime
command to check the time taken.
See the code below.
clc
clear
Start_t = cputime;
pause(1)
End_t = cputime;
Elapsed_time = End_t - Start_t
Output:
Elapsed_time =
0.2656
The difference between the actual pause time and the output in the above output is very high. So we should use the tic
and toc
commands to check the CPU time taken to run a task.
We can also use the timeit()
function to check the time taken to execute a function before executing it in Matlab. Check this link for more details about the timeit()
function.
Check this link to precisely measure the performance of your Matlab code.