How to Pause Bash Shell Script for X Seconds
- Pause Bash Script for X Seconds
- Pause Bash Script for a Variable Amount of Time
- Pause Bash Script Until User Input Occurs
- Combine Timed Pauses With User-Input Pauses
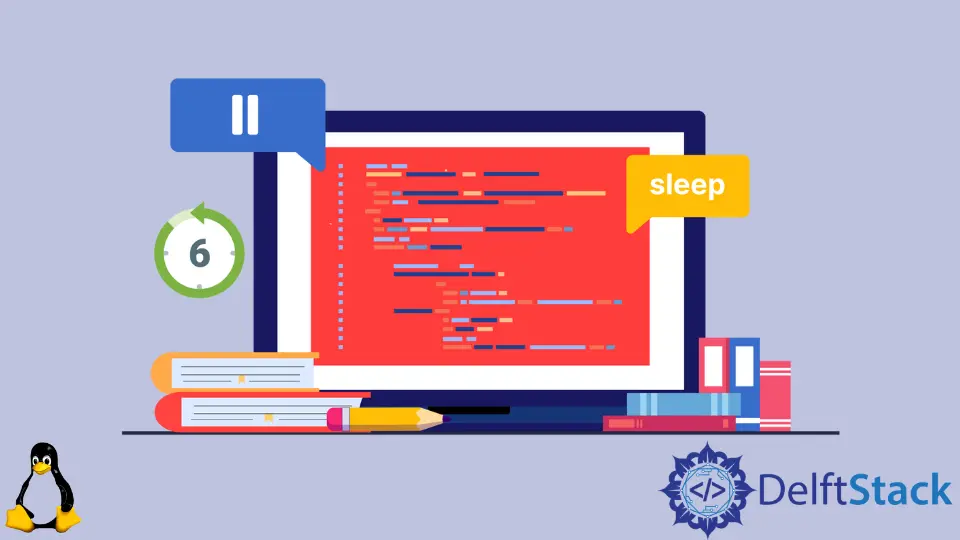
Bash scripts are pretty useful for running multiple commands that you may not want to have to type out line by line into the Bash command shell. In some scenarios, you may need to pause your script, possibly to accept input from a user, or to sleep until the result of a command has been returned.
This tutorial will explain the different ways to pause your Bash shell script.
Pause Bash Script for X Seconds
Suppose you write your script from scratch, and you decide that you’d like to show potential users a welcome message so that you have to delay starting the script to let your users can read the message, which will explain what your script will do before it starts running further Bash commands. The sleep
command in Bash can help you achieve this, detailed here. Its syntax is as follows:
sleep n
Where, n
is the number of seconds you’d like it to wait. n
can also be in smaller units, such as milliseconds, just by specifying the number as a decimal number - so if you’d like to pause for 30 milliseconds, sleep 0.030
should do the trick!
Keep in mind that n
is a compulsory argument, so not telling sleep
how long to run for wouldn’t quite work out. Running without a value for n
would produce the following error.
user@linux:~$ sleep
sleep: missing operand
Try 'sleep --help' for more information.
If you’d like to wait far longer than just seconds, sleep
also accepts arguments specifying the unit of time (i.e. minutes, hours, days), so if you’d like to wait a whole week, sleep 7d
is your solution!
Here are some use cases for sleep
.
# wait 500 milliseconds
sleep 0.5
# wait 1 second
sleep 1
# wait 20 seconds
sleep 20
To display a message and wait 1 minute:
echo "Hello! This script will run in exactly a minute!"
sleep 1m
To rerun a script to kill long-running Python processes every week.
while true
do
killall --older-than 7d python3
sleep 7d
done
Pause Bash Script for a Variable Amount of Time
In some cases, you’d like to wait a variable amount of time, probably because you’d like to run the script with varying delays based on how many scripts are running. A good example is if you’re running the same script multiple times that may need to modify the same file, each invocation of the script will need a different delay to ensure no two invocations modify the file simultaneously.
In that case, you could make n
an actual Bash-style variable, $N
, and set its value at some point in the script. One example is as follows:
N=5
sleep $N
The script that this is placed into should sleep for 5 seconds.
If you leave out the initialization for $N
, and instead specify the value as you’re calling the script, you can use the value of N
defined in the shell environment to provide the sleep
time period to the script.
user@linux:~$ cat test.sh
#! /bin/bash
sleep $N
user@linux:~$ export N=3
user@linux:~$ chmod +x test.sh
user@linux:~$ ./test.sh
As a result, you should see the script run for 3 seconds. You can even override the environment value and specify it in the script invocation by doing N=2 ./test.sh
.
You can even consider randomizing the amount of delay by using $RANDOM
as the argument to sleep
instead - thus, you can say sleep $RANDOM
.
If the range of values from $RANDOM
is too large for you, applying a modulus operation with an optional added value to ensure a minimum delay should allow you to sleep for a range of seconds. For example, to sleep for a certain time, anywhere between 10 and 30 seconds, you could do:
sleep $((10 + RANDOM % 21))
Pause Bash Script Until User Input Occurs
Sometimes, waiting a specific amount of time won’t cut it - you might want to ensure that the user has read your welcome message and/or is ready to start running your script. In that case, you want to wait for them to type something to indicate they’re ready to start.
For this, we’ll use the read
command, detailed here. read
will wait for data to be entered on standard input - the command line into which you type commands could be called the standard input - and a final Enter keystroke signals to the command that the data has been entered. The string can then be placed into a variable or simply ignored, which is the usecase we’ll consider here.
The simplest invocation of read
requires no arguments:
read
It should cause a new line to be printed, into which you type something. Pressing Enter should return you to the Bash prompt, regardless of whatever you typed previously.
Combine Timed Pauses With User-Input Pauses
An excellent use-case that would require you to combine timed pauses with user-input pauses is to ensure that you want your script to continue running if the user doesn’t respond, and it is not important for them to do so. The read
command allows us to do this by specifying a -t
parameter, which takes several seconds for the command to wait for before timing out if the Enter<> key is not pressed. It would be used as follows.
read -t 5
As a result, read
will quit when the Enter key is pressed, or if 5 seconds pass, whichever comes first.
Another use-case would be waiting for a specified amount of time specified by the user themselves. In that case, you could wait for the user to input an integer value, then pass it as the argument to sleep
, as follows.
echo -n "Enter time to sleep: "
read $time
sleep $time
Of course, this is under the assumption that the user would enter a valid argument for sleep
.
Let’s recap with some examples!
sleep 3
will pause a script for exactly 3 seconds.sleep 3d
will pause a script for exactly 3 days.sleep $N
will pause a script for the number of seconds specified as$N
.sleep $((10 + RANDOM % 21))
will sleep for a random period of time within 10-30 seconds.read
waits for the user to input something.read -t 3
waits for the user to input something but times out at 3 seconds if the Enter key is never pressed.read $time && sleep $time
will take a string argument from the user and use it as the argument tosleep
, allowing the user to specify how long to sleep.