How to Suppress Output in Bash
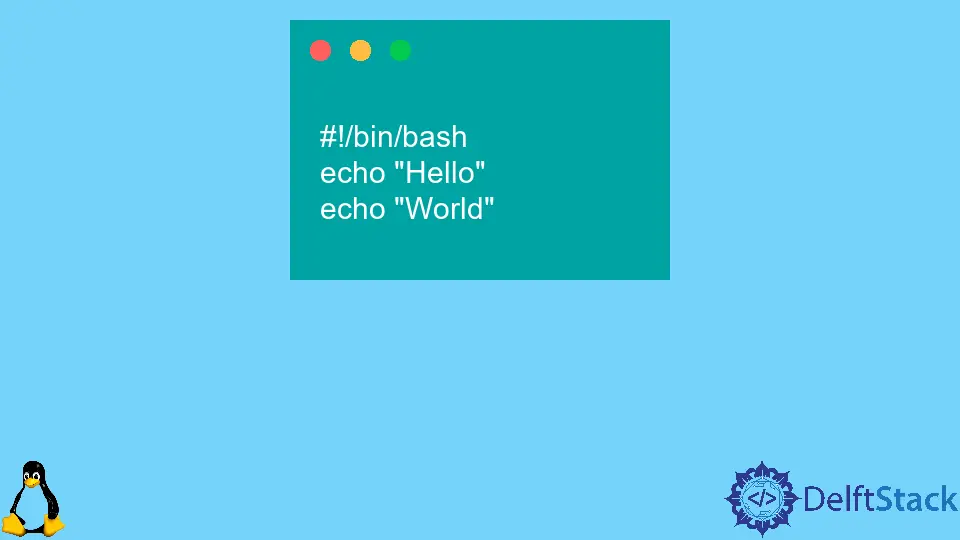
This article will explain methods to suppress the output of commands using Bash. Moreover, we will also look into redirecting the output to a file for later use.
Introduction to Shell and Scripting
Shell is a program that runs a command line interface in Linux. In Linux, all users can use many different shells.
Commonly, a default GNU’s Bourne-Again Shell (Bash) is used in many Linux distributions.
A file containing a series of commands is called a script. This file is read and executed using the Bash program.
For example, assume a file with the name First.sh
has contents as follows:
#!/bin/bash
echo "Hello"
echo "World"
In the above example, First.sh
is a script file that contains two echo
commands that will display Hello World
on the terminal when the script executes. The first line, #!/bin/bash
, flags the interpreter’s location to be used for running the script.
We can execute the Bash script on the Linux terminal in two different ways.
bash First.sh
Output:
Hello
World
./First.sh
Output:
Hello
World
Suppress Output of Command in Bash Script
We can suppress the output of any commands written in a Bash script during the execution of a Bash script. We can redirect the output to a null
device.
For example, if we want to suppress the output of the First.sh
script, we can use the following command.
./First.sh > /dev/null
This command suppresses the output of the First.sh
script and does not display anything on the terminal. However, if the First.sh
script contains any error, it will still be displayed on the terminal.
Consider the following command in the First.sh
script.
#!/bin/bash
echo "Hello
echo "World"
In the script, "
is missing in the first echo
command. The output of the following script run using ./First.sh
is as follows:
./First.sh: line 3: unexpected EOF while looking for matching `"'
./First.sh: line 4: syntax error: unexpected end of file
If we run this script using the command ./First.sh > /dev/null
will show the same output.
./First.sh: line 3: unexpected EOF while looking for matching `"'
./First.sh: line 4: syntax error: unexpected end of file
Suppress Errors of a Bash Script
If any Bash script contains an error, the ./scriptname > dev/null
command does not suppress the error and displays the error on the terminal, as discussed above.
We can suppress the error from the terminal, also. The following commands can suppress the output and the error from the terminal while executing the First.sh
Bash script.
./First.sh &>/dev/null
./First.sh >/dev/null 2>&1
./First.sh >/dev/null 2>/dev/null
The first command ./First.sh &>/dev/null
maybe not work for all Linux shells.
Record or Store the Output of Bash Scripts
The output is suppressed when we use the /dev/null
during the execution of the Bash script. However, we can store or record the output in customized files.
Consider the following First.sh
script.
#!/bin/bash
echo "Hello"
echo "World"
We can use the following command to run the First.sh
script to store or record the Bash output.
./First.sh > outputfile.out
When we execute the above command, it will not show anything on the terminal; however, a new file with the name outputfile.out
is created (if not exists already), and the contents of the file are:
Hello
World
If the outputfile.out
already exists, the existing content is varnished, and the script’s new output is stored in the file.